SDK error handling
Hello π
We have our custom integration, using REST API.
Endpoint example:
SDK folder:
API Client folder:
Using integration with Next.js application.
when calling this endpoint fails, getting eror:
What am I missing, how/where should we properly handle errors with integration ?
Thanks!
30 Replies
Hi π , if you rethrow the error, isn't this the expected behavior?
hm. it seems i'm not following, sorry
expected behaviour would be to get Error I send from
api-client
endpoint - as I am catching it there..
something like that :
but I am getting
that is why I am asking.. how should I handle errors normally ?
- I handle errors on api-client
endpoint method
- on sdk
endpoint method
but when I catch error in Next.js app - I can't see the message - which actuatlly sends our API, but SDKError: Request failed with status code 400
π
and I see in Chrome Network tab - my expected error, but not in the .catch
method of the SDK response πI see, I'll check with the engineering team and get back to you.
this is the code of the default error handler, it expects an object with a message so simply throw { message: throw error.response.data.detail } instead, or add your error handler. Because this is a default handler, we do not expose any sensitive or additional data as we are not able to predict all possible data structures and its sensitivity
it applies to the latest middleware package
@valerii.f ^^
Hey @bartoszherba ,
thanks for the update.
could you please clarify - what do you mean
or add your error handler
?
Where exactly can I add this handler?In the
middleware.config
file you can add an errorHandler
key with a function
this way you can customize/override default loggerit doesn't seems to be working for me π¦
bellow is my
middleware.config.js
file
server restarted after updating the file.
@bartoszherba Could you please point me where am I wrong ?
Thanks!Hi @bartoszherba , @rohrig , I am also getting the same issue when i try to add error handler in my configuration file. Can you help us where we are going wrong. Thanks!
Could you share middleware version that you are using?
A configurable error logger was added in 3.1.0
Hi @bartoszherba , my version in same as what you mentioned which is 3.1.0 . When I tried to add logger inside of error Handler i am not seeing anything logged in terminal.
Hi @bartoszherba , First of all Thanks. It worked but not in all cases. In one scenario where at the time of log in i am using the unified API . In that case ,this error handler is not getting invoked at all and instead of my customized error handler it is using old error handling mechanism .
Could you provide me with the actual code, or simplified so I can try to reproduce your issue?
integrations : {
commerce : {
...getCommerceConfig(INTEGRATION),
extensions:(extensions)=>[...extensions],
errorHandler:(error:Unknown,req:Request,res:Response)=>{
console.log(error)
}
}
AnotherIntegrationName : {
...getCommerceConfig(INTEGRATION)
extensions:(extensions)=>[...extensions,{custom extensions}],
errorHandler:(error:Unknown,req:Request,res:Response)=>{
console.log(error)
}
} } Hi @bartoszherba , The customized error handler in middleware.config file is ineffective at capturing GraphQL errors specifically. Instead, it successfully intercepts errors such as network errors and syntax errors. It appears that the predefined default error handler manages GraphQL errors upon reception.
} } Hi @bartoszherba , The customized error handler in middleware.config file is ineffective at capturing GraphQL errors specifically. Instead, it successfully intercepts errors such as network errors and syntax errors. It appears that the predefined default error handler manages GraphQL errors upon reception.
because graphql error is not an error that is thrown but 200 response with an error object inside, so we do not catch it
In my local setup, I noticed that when a commerce platform generates a response containing an error message in its body, that response is forwarded to the middleware. However, the middleware fails to relay the received response back to the Next.js application. As a result, the request remains pending in the application browser. Consequently, I believe implementing this customized error handler could resolve the issue.
does it happen in with customized code or core?
core
can you upgrade to the latest version?
Upon receiving a response containing an error message in its body, the message is transmitted from the commerce platform to the middleware. Within the middleware, the predefined error handler registers the message as a GraphQL error and logs it in the middleware console. Subsequently, the response fails to propagate to the client application browser. Consequently, throughout the process, the request in the network tab remains indefinitely pending, neither displaying a success nor failure status.
Upgrade to the latest version should fix this problem
Is this the correct command for verifying the middleware version? npm show @vue-storefront/middleware version . if it is true then my version of middleware is 3.8.0
yarn list --pattern @vue-storefront/middleware
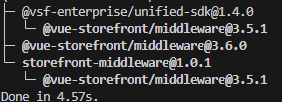
Is this a updated version or do i need to upgrade my version ? If I should upgrade to latest version, can you help me with upgrade command.
Hi @bartoszherba, @rohrig , I upgraded my middleware version to 3.8.0 but I am still seeing the same issue in my local.
hey π , I've raised the issue internally. I'll let you know what we find. Thanks for your patience. π
Yeah sure @rohrig , Thanksπ
@lucifer2732 can you share the code and reproduction steps? I can't reproduce this issue.
Hi @bartoszherba , We haven't customized extensively, yet we've encountered this issue persistently since the beginning. Our data retrieval from CommerceTools relies on GraphQL queries. The default method for logging in users is LoginCustomer, sourced from the unified module. And all these are predefined things from storefront side.
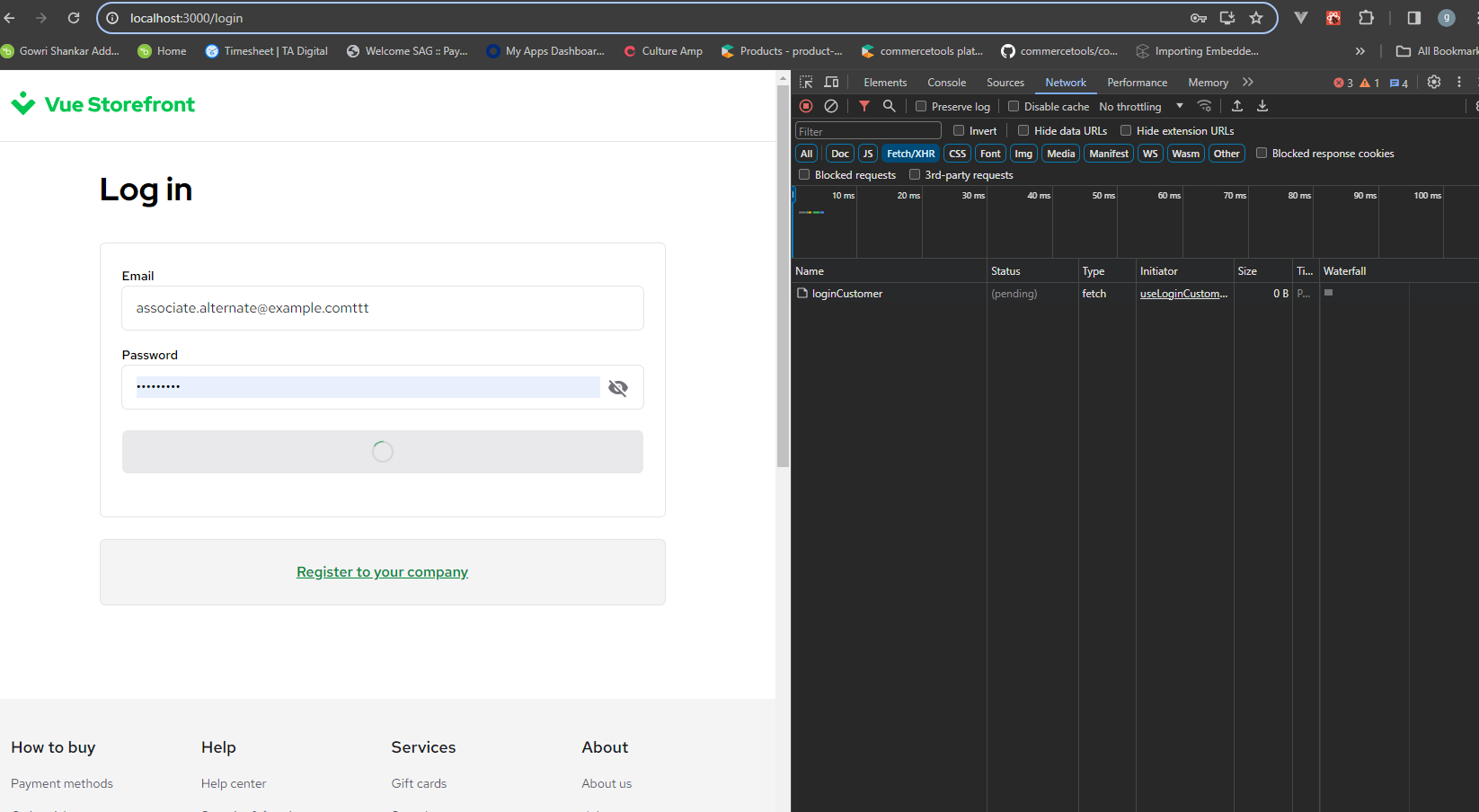
