How to do this with LINQ?
```
var values = new []{ 1, 2, 3, 4, 5}
int winner;
for(int i = 0; i < values.Count; i++)
{
var value = values[i];
var r = value * 2;
if(r > 7)
{
winner = r;
break;
}
}
41 Replies
What does value end up doing
var winner = values.FirstOrDefault(x => x * 2 > 7)
?I know I can do values.Select(v => v * 2).Where(r => r > 7).First()
but I want to do it efficiently
More like LastOrDefault
I don't want to do the computation if something wins early
why last? it breaks at the first winner
they’re comparing indices not values are they not?
Ah true
yeah i'm assuming there's a typo in this part
This one is pretty much efficient, linq chain is executed as many times as needed
yeah the only efficiency to be gained is typing less by putting the whole expression in FirstOrDefault
(default because there may not be any winner)
in my case I have a more complex algorithm I don't want to run if there is an early winner
and performance wise, a plain loop is probably faster than linq anyway
First(OrDefault) runs till it either finds a winner or collection ends
Their difference is the behavior in case of no winners
the problem with first or default is it doesn't remember the computation
Tempted to agree but LINQ’s probably been optimized to hell they could be virtually the same
Wym
values.FirstOrDefault(v => v x 2 > 7) x 2
This, or
I would have to do the math again on the winning value
cause it doesn't remember the computation that happened in the lamba
values.Select(x => x * 2).FirstOrDefault(x => x > 7)
^
but then you don't know who the winner is
I want to terminate early
Do they need to?
so I can't just select on all values
select doesn't enumerate the whole list
I guess you don't understand linq chains
linq is evaluated lazily
the computation is only done when the next value is needed, like when you're materializing the result with First
It doesn't equal to two loops: one for .Select and one for FirstOrDefault
It equals to one loop which firstly transforms and then checks and may or may not return
how many values are you computing this on?
All Select does is create an object to hold the lambda and source array/list (very watered down explanation)
because unless it's millions it doesn't even matter
a single multiplication is very cheap
oh it runs both lambdas on the first value and will only proceed to the next if the 2nd lambda condition is not met?
essentially
More or less
oh, you learn something new everyday
thanks guys 🙂
$close
Use the /close command to mark a forum thread as answered
Yeah both lambdas get evaluated in the FirstOrDefault call
i will reiterate that if performance is the goal and you're concerned about a single multiplication taking too long, you shouldn't be using linq
But linq is so comfy 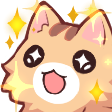
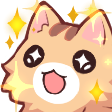