Week 55 — What is JUnit and what is it used for?
Question of the Week #55
What is JUnit and what is it used for?
6 Replies
In order to verify a program working correctly, it is possible to write additional programs that test the functionality the program.
Since programs often are fairly complex, it can become unreasonable to test a whole program as a black box hence developers can write "unit tests" that test small parts of the program.
These unit tests can be compiled together with the program and run specific methods of the said program.
JUnit is a framework that simplifies writing unit tests in Java.
With JUnit, test methods are annotated with
@Test
and have access to various methods like assertEquals
that check whether results are as expected.
For example, a program could contain a method that adds two numbers:
Then, one can write a test class with test methods that runs the add
method with different parameters and check the results:
IDEs can then run tests and report which tests run successfully and which fail. In the above example, all tests run successfully.
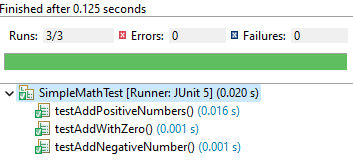
However, we can also add failing tests like the following:
Running this test will fail and IDEs can report that.
When tests fails, developers should investigate the test failures and fix the issues in the code if the fails were caused by a bug in the program.
📖 Sample answer from dan1st
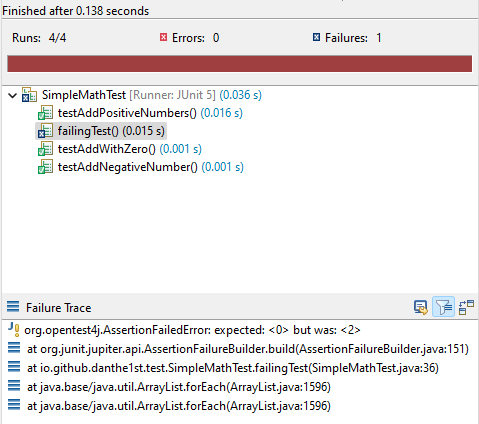
JUnit is a testing framework. You can use it to write tests, to see if the code you wrote still does exactly what it's supposed to do. IDEs or build tools can then use the junit engine to run those tests, and optionally export the results to a file for further documentation, or let the build fail if any tests failed.
All JUnit test methods have the
@Test
annotation, to signify they should be executed by the junit engine. If a JUnit test method runs without any exceptions, it is considered successful, and the test passes. If a test method throws an AssertionFailedError
, the test is considered failed. If any other exceptions get thrown, the test is also considered failed, but for the reason that the test itself has an error somewhere.
JUnit provides the Assertions
class, which has utility methods for writing assertions, which fail with an AssertionFailedError. You can use the methods provided by that class to test the output of library functions.
There are also ways of making sure code throws / does not throw a certain exception:
there are a lot of other features in junit that make writing tests easier, and all of those can be read about here: https://junit.org/junit5/docs/current/user-guide/
⭐ Submission from 0x150
The JUnit API is one of the Unit testing frameworks for Java programming, means testing a unit of code by writing "TestCases" and Check whether the output matching the expected value using "Assertion methods"
Submission from n.karthi