Casting, reflection or?
I'm trying to write a simple lib for consuming a few JSON API endpoints and processing their contents with callbacks.
I'm trying to cast the "parsed" variable to its actual class (as it is known during runtime). Psuedo code as follows:
Is this just impossible or am I implenting this stuff wrong?
44 Replies
what does the json look like? AFAIK STJ supports using a discriminator value for serializing subclasses
What is STJ?
System.Text.Json
the C# json library
All of the psuede code works, Except for when I try to run the "callback" with the TypeA signature
I don't know why it doesn't accept the implementation of an interface
you mean in your dictionary?
because the signature isn't compatible
your dictionary holds
Action<IType>
you can't give it a method that only accepts a specific implementationI tried changing that ot Action<Object> but that also didn't take
if it's specialized, just cast it inside the method
Hmm
I've been doing that but just think that is ulgy
but this code looks off to me in general
which is why i was asking what the json actually looks like
why are you getting the type of whatever GetJson returns and using that to decide how to deserialize?
It can be a lot of stuff, there are 2 commons fields but otherwise there can be objects in there, strings bools what have you
so it's completely dynamic and not a fixed set of possible models?
There is a fixed set
you should model that properly in your code
How would I go about doing that?
i don't know, your Get method doesn't make sense the way i'm looking at it
Its on a websocket, so all models can come in whenever
how do you know which type a message is?
Like so
And other "TryGetProperties" for other "top-level" action indicators
so there is no actual discriminator, you just have to see if it looks like a certain type?
Correct
that's gross
do you have control over that?
No
And such
that looks exactly like the discriminator i'm asking about, is
action
not always present in a normal message?No
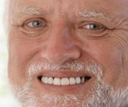
Wait I'll double check to be sure
It's only the "error" and "action" ones
but maybe "error" has "action"
i would try to find a good candidate for a discriminator because then you can use that with STJ's polymorphic serialization
How to serialize properties of derived classes with System.Text.Jso...
Learn how to serialize polymorphic objects while serializing to and deserializing from JSON in .NET.
then all you have to do to handle each message is let STJ deserialize it then pattern match on the type
Ah, I initially wrote this in .net 6
should still be available
"Beginning with .NET 7, System.Text.Json supports polymorphic type hierarchy serialization and deserialization with attribute annotations."
or not
I can update no problem, so I'll read this thx 🙂
But even with this, I'd still be casting in the DoStuffTypeA right
This is simplifying the parsing, not the typing
correct, you'd still have to decide what to do based on what the message is
which i wouldn't use a dictionary for at all, just pattern match
etc
its a lib, so that wouldn't work
As I'd have to know the methods used in the program beforehand
That's why I use the dict
do you know the messages beforehand or can those also be different?
Which ones do you mean?
the ones you're deserializing
those I know beforehand
Except if the API changes, then I'd have to update the lib
But that is fine
so you could also just have a class that holds callbacks that the user can set individually
like
That would work
it avoids the problem of having to find a common signature for all the handlers
As well as not having to do reflection
I stayed away from that type of implementation because I'd have to double everything (in .net 6)
but seeing as this new feature will probably reduce my parsing file from 300 lines to a 20 line function, that'd be fine
Guess I have a little rewrite to do, thanks :)!