The code does not change the new number
embed part
button part
const args = msg.content.trim().split(/ +/g);
let num = args[1];
if (num === undefined) {
msg.reply("**`-` قم بوضع المبلغ**");
} else {
let regex = /^([0-9]+\.?[0-9]*)([a-zA-Z]*)$/;
let matched = num.match(regex);
if (matched !== null) {
let amount = parseFloat(matched[1]);
let unit = matched[2].toLowerCase();
if (unit === 'k') {
amount = amount * 1000;
} else if (unit === 'm') {
amount = amount * 1000000;
}
let replaced = amount.toLocaleString();
let waistTax = 2.50;
let tax2 = Math.floor(amount * waistTax / 100);
let tax3 = Math.floor(tax2 + probot.taxs(amount));
let tax1 = Math.floor(tax2 + probot.taxs(probot.taxs(amount)));
if(amount < 5000) return msg.reply(`**\`-\` قم بوضع مبلغ أكبر من \`5000\`**`);
if(amount > 300000000) return msg.reply(`**\`-\` قم بوضع مبلغ أقل من \`300000000\`**`);
let taxEmbed = new MessageEmbed()
.setAuthor({ name: `Bot Tax.`, iconURL: `` })
.setColor("#382c5e")
.setThumbnail('')
.addFields(
{ name: '**💰 2.5% Tax:**', value: `${tax2}` },
{ name: '**💰 Price (1 Tax):**', value: `${probot.taxs(amount)}` },
{ name: '**💰 Price (2 Taxes):**', value: `${probot.taxs(probot.taxs(amount))}` },
{ name: '**💰 Price (1 Tax) + 2.5%:**', value: `${tax3}` },
{ name: '**💰 Price (2 Tax) + 2.5%:**', value: `${tax1}` }
)
.setFooter({ text: msg.guild.name, iconURL: '.' })
.setTimestamp();
const args = msg.content.trim().split(/ +/g);
let num = args[1];
if (num === undefined) {
msg.reply("**`-` قم بوضع المبلغ**");
} else {
let regex = /^([0-9]+\.?[0-9]*)([a-zA-Z]*)$/;
let matched = num.match(regex);
if (matched !== null) {
let amount = parseFloat(matched[1]);
let unit = matched[2].toLowerCase();
if (unit === 'k') {
amount = amount * 1000;
} else if (unit === 'm') {
amount = amount * 1000000;
}
let replaced = amount.toLocaleString();
let waistTax = 2.50;
let tax2 = Math.floor(amount * waistTax / 100);
let tax3 = Math.floor(tax2 + probot.taxs(amount));
let tax1 = Math.floor(tax2 + probot.taxs(probot.taxs(amount)));
if(amount < 5000) return msg.reply(`**\`-\` قم بوضع مبلغ أكبر من \`5000\`**`);
if(amount > 300000000) return msg.reply(`**\`-\` قم بوضع مبلغ أقل من \`300000000\`**`);
let taxEmbed = new MessageEmbed()
.setAuthor({ name: `Bot Tax.`, iconURL: `` })
.setColor("#382c5e")
.setThumbnail('')
.addFields(
{ name: '**💰 2.5% Tax:**', value: `${tax2}` },
{ name: '**💰 Price (1 Tax):**', value: `${probot.taxs(amount)}` },
{ name: '**💰 Price (2 Taxes):**', value: `${probot.taxs(probot.taxs(amount))}` },
{ name: '**💰 Price (1 Tax) + 2.5%:**', value: `${tax3}` },
{ name: '**💰 Price (2 Tax) + 2.5%:**', value: `${tax1}` }
)
.setFooter({ text: msg.guild.name, iconURL: '.' })
.setTimestamp();
const collector = msg.channel.createMessageComponentCollector({ type: 'SELECT_MENU' });
collector.on('collect', async (collect) => {
if (collect.values[0] === 'amount') {
collect.reply({ content: `\`c ${amount}\``, ephemeral: true });
} else if (collect.values[0] === '2') {
collect.reply({ content: `${tax2}`, ephemeral: true });
} else if (collect.values[0] === 'tax1') {
if (amount > 4000000) {
await collect.reply({
content: `\`c 1071812517690613831 ${probot.taxs(amount)}\``,
ephemeral: true
});
} else {
collect.reply({ content: `\`c ${collect.member.id} ${tax1}\``, ephemeral: true });
}
} else if (collect.values[0] === 'tax2') {
if (amount > 4000000) {
await collect.reply({
content: `\`c 1071812517690613831 ${probot.taxs(probot.taxs(amount))}\``,
ephemeral: true
});
} else {
collect.reply({ content: `\`c ${collect.member.id} ${tax2}\``, ephemeral: true });
}
} else if (collect.values[0] === 'tax1+2') {
if (amount > 4000000) {
await collect.reply({
content: `\`c 1071812517690613831 ${tax3}\``,
ephemeral: true
});
} else {
collect.reply({ content: `\`c ${collect.member.id} ${tax3}\``, ephemeral: true });
}
} else if (collect.values[0] === 'tax2+2') {
if (amount > 4000000) {
await collect.reply({
content: `\`c 1071812517690613831 ${tax1}\``,
ephemeral: true
});
} else {
collect.reply({ content: `\`c ${collect.member.id} ${tax1}\``, ephemeral: true });
}
}
});
const MSG = await msg.reply({ embeds: [taxEmbed], components: [row2] });
const collector = msg.channel.createMessageComponentCollector({ type: 'SELECT_MENU' });
collector.on('collect', async (collect) => {
if (collect.values[0] === 'amount') {
collect.reply({ content: `\`c ${amount}\``, ephemeral: true });
} else if (collect.values[0] === '2') {
collect.reply({ content: `${tax2}`, ephemeral: true });
} else if (collect.values[0] === 'tax1') {
if (amount > 4000000) {
await collect.reply({
content: `\`c 1071812517690613831 ${probot.taxs(amount)}\``,
ephemeral: true
});
} else {
collect.reply({ content: `\`c ${collect.member.id} ${tax1}\``, ephemeral: true });
}
} else if (collect.values[0] === 'tax2') {
if (amount > 4000000) {
await collect.reply({
content: `\`c 1071812517690613831 ${probot.taxs(probot.taxs(amount))}\``,
ephemeral: true
});
} else {
collect.reply({ content: `\`c ${collect.member.id} ${tax2}\``, ephemeral: true });
}
} else if (collect.values[0] === 'tax1+2') {
if (amount > 4000000) {
await collect.reply({
content: `\`c 1071812517690613831 ${tax3}\``,
ephemeral: true
});
} else {
collect.reply({ content: `\`c ${collect.member.id} ${tax3}\``, ephemeral: true });
}
} else if (collect.values[0] === 'tax2+2') {
if (amount > 4000000) {
await collect.reply({
content: `\`c 1071812517690613831 ${tax1}\``,
ephemeral: true
});
} else {
collect.reply({ content: `\`c ${collect.member.id} ${tax1}\``, ephemeral: true });
}
}
});
const MSG = await msg.reply({ embeds: [taxEmbed], components: [row2] });
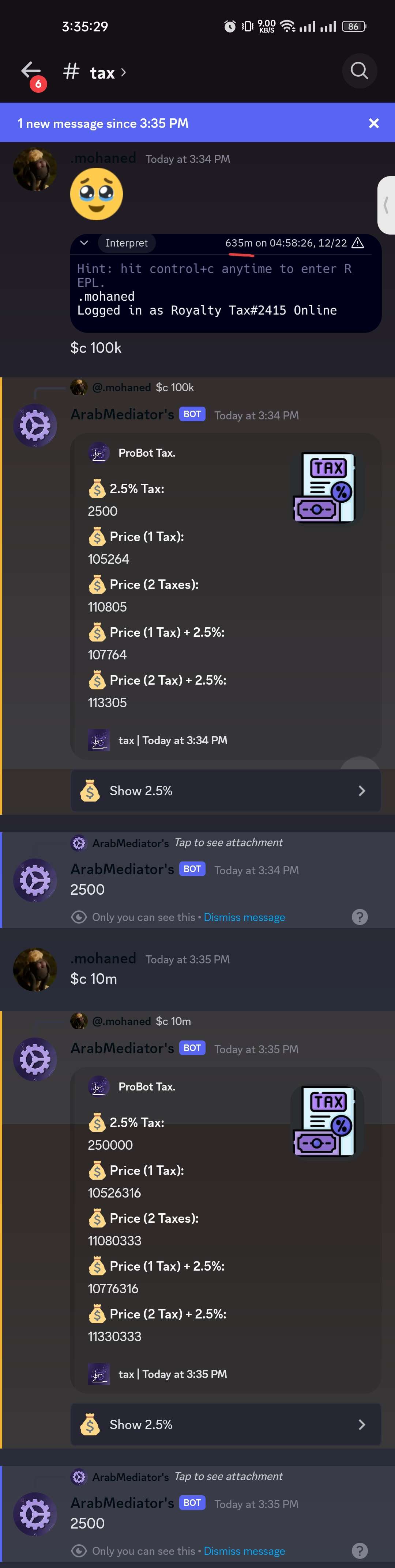
4 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!
- ✅
Marked as resolved by staffUnknown User•13mo ago
Message Not Public
Sign In & Join Server To View
he told me to come here
:)
Unknown User•13mo ago
Message Not Public
Sign In & Join Server To View