Can anyone help me to solve this error.
link to the full code i write. https://github.com/oderoi/Mojo/blob/main/examples/matrix.mojo
GitHub
Mojo/examples/matrix.mojo at main · oderoi/Mojo
Mojo Programming Language. Contribute to oderoi/Mojo development by creating an account on GitHub.
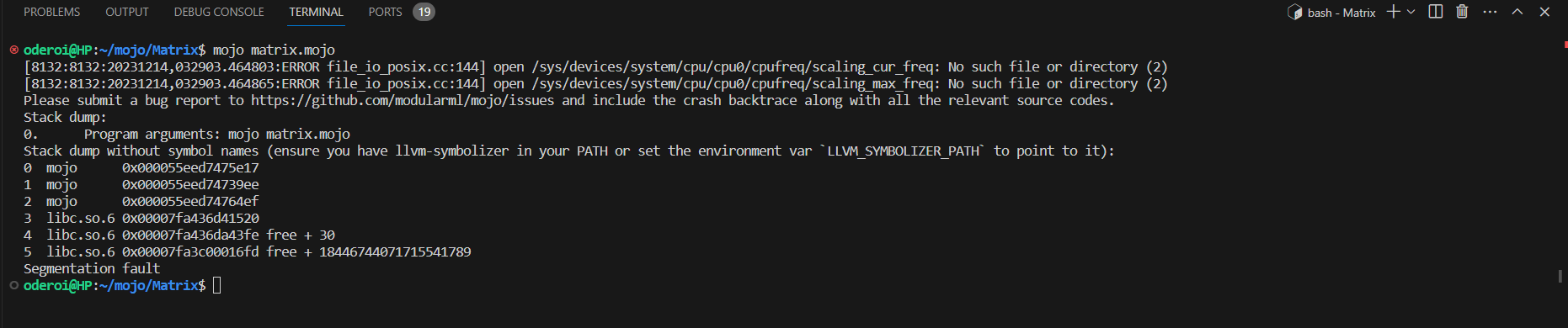
6 Replies
It looks like a double free, you're calling
A.data.free()
and etc. for all the matrices after the benchmark is run, but when they get destructed, each __del__
gets called, freeing the pointers again. You can keep the matrices alive a different way, like I just changed those A.data.free()
calls to _ = A.rows
or quite literally anything that uses the matrices, or remove the free call in __del__
. I think the first option is "better" practice for now.
This is what I ran, and it appears to work as expected.
thank you , this helps ,...
Also , if i uncomment this :
#let speedup: Float64 = gflops / base_gflops
#print(gflops, "GFLOP/s, a", speedup, "x speedup over Python")
and add :
print(gflops, "GFLOP/s", speedup, "speedup")
I get that error :
can you help me with that as well if you may.
Thank you.

Wow, this is a bad bug. Looks like an actual language one, there's something bad happening under the hood. So far I can't see a way to get around it, would be good to create an issue on the Github https://github.com/modularml/mojo/issues. I took a look and didn't see anything similar to this previously reported.
ok, thank you so much
Wait, I completely missed something
The reason is because you're passing the type
Float64
into bench
at the end, when it really expects something of the type Float64
, like 1.0
or a variable
I guess it's true the compiler should emit a better error than this, but changing that last Float64
to something like 0.5
(I don't know the actual base GFLOPS that Python runs this at) works
I think you can go ahead and still make an issue, but make it instead about a better error in this case. Because something like:
should probably produce an error less crypticHi, I have a question ,... using that Matrix struct above, can i perform vectorization in matrix multiplication fn:
@always_inline
fn mul(self, other: SIMD[type, 1])->Matrix[type]:
alias simd_width=simdwidthoftype
let result=Matrix[type](self.rows, self.cols)
for y in range(self.rows):
for x in range(self.cols):
result[y, x]=other * self.data.simd_load[1](y * self.cols + x)
return result
or should i add Tensor in struct :
struct[t: Tensor]:
is it possible ?