Roles not being changed
else if(interaction.isStringSelectMenu()) // String Select Menu Interactions
{
try
{
let roles = interaction.member.roles.cache;
const value = interaction.values;
const COUNTERSTRIKE = interaction.guild.roles.cache.get(ROLE_IDS.GAMES_COUNTERSTRIKE);
const FORTNITE = interaction.guild.roles.cache.get(ROLE_IDS.GAMES_FORTNITE);
const LEAGUEOFLEGENDS = interaction.guild.roles.cache.get(ROLE_IDS.GAMES_LEAGUEOFLEGENDS);
const MINECRAFT = interaction.guild.roles.cache.get(ROLE_IDS.GAMES_MINECRAFT);
const OVERWATCH = interaction.guild.roles.cache.get(ROLE_IDS.GAMES_OVERWATCH);
const RAINBOWSIX = interaction.guild.roles.cache.get(ROLE_IDS.GAMES_RAINBOWSIX);
const ROBLOX = interaction.guild.roles.cache.get(ROLE_IDS.GAMES_ROBLOX);
const ROCKETLEAGUE = interaction.guild.roles.cache.get(ROLE_IDS.GAMES_ROCKETLEAGUE);
const VALORANT = interaction.guild.roles.cache.get(ROLE_IDS.GAMES_VALORANT);
var isCounterstrike = false;
var isFortnite = false;
var isLeagueoflegends = false;
var isMinecraft = false;
var isOverwatch = false;
var isRainbowsix = false;
var isRoblox = false;
var isRocketleague = false;
var isValorant = false;
roles.map(COUNTERSTRIKE => COUNTERSTRIKE.id);
roles.map(FORTNITE => FORTNITE.id);
roles.map(LEAGUEOFLEGENDS => LEAGUEOFLEGENDS.id);
roles.map(MINECRAFT => MINECRAFT.id);
roles.map(OVERWATCH => OVERWATCH.id);
roles.map(RAINBOWSIX => RAINBOWSIX.id);
roles.map(ROBLOX => ROBLOX.id);
roles.map(ROCKETLEAGUE => ROCKETLEAGUE.id);
roles.map(VALORANT => VALORANT.id);
for(var i = 0; i < value.length; i++)
{
if(value[i] == 'games_counterstrike')
isCounterstrike = true;
else if(value[i] == 'games_fortnite')
isFortnite = true;
else if(value[i] == 'games_leagueoflegends')
isLeagueoflegends = true;
else if(value[i] == 'games_minecraft')
isMinecraft = true;
else if(value[i] == 'games_overwatch')
isOverwatch = true;
else if(value[i] == 'games_rainbowsix')
isRainbowsix = true;
else if(value[i] == 'games_roblox')
isRoblox = true;
else if(value[i] == 'games_rocketleague')
isRocketleague = true;
else if(value[i] == 'games_valorant')
isValorant = true;
}
if(!isCounterstrike)
roles.delete(ROLE_IDS.GAMES_COUNTERSTRIKE);
else if(!isFortnite)
roles.delete(ROLE_IDS.GAMES_FORTNITE);
else if(!isLeagueoflegends)
roles.delete(ROLE_IDS.GAMES_LEAGUEOFLEGENDS);
else if(!isMinecraft)
roles.delete(ROLE_IDS.GAMES_MINECRAFT);
else if(!isOverwatch)
roles.delete(ROLE_IDS.GAMES_OVERWATCH);
else if(!isRainbowsix)
roles.delete(ROLE_IDS.GAMES_RAINBOWSIX);
else if(!isRoblox)
roles.delete(ROLE_IDS.GAMES_ROBLOX);
else if(!isRocketleague)
roles.delete(ROLE_IDS.GAMES_ROCKETLEAGUE);
else if(!isValorant)
roles.delete(ROLE_IDS.GAMES_VALORANT);
return interaction.member.roles.set(roles)
.then((member) =>
interaction.reply(
{
embeds: [EMBEDS.ROLE_CHANGED],
ephemeral: true,
}))
}catch(err)
{
return interaction.reply(
{
embeds: [EMBEDS.ERROR],
ephemeral: true,
});
}
}
else if(interaction.isStringSelectMenu()) // String Select Menu Interactions
{
try
{
let roles = interaction.member.roles.cache;
const value = interaction.values;
const COUNTERSTRIKE = interaction.guild.roles.cache.get(ROLE_IDS.GAMES_COUNTERSTRIKE);
const FORTNITE = interaction.guild.roles.cache.get(ROLE_IDS.GAMES_FORTNITE);
const LEAGUEOFLEGENDS = interaction.guild.roles.cache.get(ROLE_IDS.GAMES_LEAGUEOFLEGENDS);
const MINECRAFT = interaction.guild.roles.cache.get(ROLE_IDS.GAMES_MINECRAFT);
const OVERWATCH = interaction.guild.roles.cache.get(ROLE_IDS.GAMES_OVERWATCH);
const RAINBOWSIX = interaction.guild.roles.cache.get(ROLE_IDS.GAMES_RAINBOWSIX);
const ROBLOX = interaction.guild.roles.cache.get(ROLE_IDS.GAMES_ROBLOX);
const ROCKETLEAGUE = interaction.guild.roles.cache.get(ROLE_IDS.GAMES_ROCKETLEAGUE);
const VALORANT = interaction.guild.roles.cache.get(ROLE_IDS.GAMES_VALORANT);
var isCounterstrike = false;
var isFortnite = false;
var isLeagueoflegends = false;
var isMinecraft = false;
var isOverwatch = false;
var isRainbowsix = false;
var isRoblox = false;
var isRocketleague = false;
var isValorant = false;
roles.map(COUNTERSTRIKE => COUNTERSTRIKE.id);
roles.map(FORTNITE => FORTNITE.id);
roles.map(LEAGUEOFLEGENDS => LEAGUEOFLEGENDS.id);
roles.map(MINECRAFT => MINECRAFT.id);
roles.map(OVERWATCH => OVERWATCH.id);
roles.map(RAINBOWSIX => RAINBOWSIX.id);
roles.map(ROBLOX => ROBLOX.id);
roles.map(ROCKETLEAGUE => ROCKETLEAGUE.id);
roles.map(VALORANT => VALORANT.id);
for(var i = 0; i < value.length; i++)
{
if(value[i] == 'games_counterstrike')
isCounterstrike = true;
else if(value[i] == 'games_fortnite')
isFortnite = true;
else if(value[i] == 'games_leagueoflegends')
isLeagueoflegends = true;
else if(value[i] == 'games_minecraft')
isMinecraft = true;
else if(value[i] == 'games_overwatch')
isOverwatch = true;
else if(value[i] == 'games_rainbowsix')
isRainbowsix = true;
else if(value[i] == 'games_roblox')
isRoblox = true;
else if(value[i] == 'games_rocketleague')
isRocketleague = true;
else if(value[i] == 'games_valorant')
isValorant = true;
}
if(!isCounterstrike)
roles.delete(ROLE_IDS.GAMES_COUNTERSTRIKE);
else if(!isFortnite)
roles.delete(ROLE_IDS.GAMES_FORTNITE);
else if(!isLeagueoflegends)
roles.delete(ROLE_IDS.GAMES_LEAGUEOFLEGENDS);
else if(!isMinecraft)
roles.delete(ROLE_IDS.GAMES_MINECRAFT);
else if(!isOverwatch)
roles.delete(ROLE_IDS.GAMES_OVERWATCH);
else if(!isRainbowsix)
roles.delete(ROLE_IDS.GAMES_RAINBOWSIX);
else if(!isRoblox)
roles.delete(ROLE_IDS.GAMES_ROBLOX);
else if(!isRocketleague)
roles.delete(ROLE_IDS.GAMES_ROCKETLEAGUE);
else if(!isValorant)
roles.delete(ROLE_IDS.GAMES_VALORANT);
return interaction.member.roles.set(roles)
.then((member) =>
interaction.reply(
{
embeds: [EMBEDS.ROLE_CHANGED],
ephemeral: true,
}))
}catch(err)
{
return interaction.reply(
{
embeds: [EMBEDS.ERROR],
ephemeral: true,
});
}
}
16 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!currently it just shows my "ROLE_CHANGED" embed
though no roles are actually changed
k
this didnt work either
else if(interaction.isStringSelectMenu()) // String Select Menu Interactions
{
try
{
const value = interaction.values;
const COUNTERSTRIKE = interaction.guild.roles.cache.get(ROLE_IDS.GAMES_COUNTERSTRIKE);
const FORTNITE = interaction.guild.roles.cache.get(ROLE_IDS.GAMES_FORTNITE);
const LEAGUEOFLEGENDS = interaction.guild.roles.cache.get(ROLE_IDS.GAMES_LEAGUEOFLEGENDS);
const MINECRAFT = interaction.guild.roles.cache.get(ROLE_IDS.GAMES_MINECRAFT);
const OVERWATCH = interaction.guild.roles.cache.get(ROLE_IDS.GAMES_OVERWATCH);
const RAINBOWSIX = interaction.guild.roles.cache.get(ROLE_IDS.GAMES_RAINBOWSIX);
const ROBLOX = interaction.guild.roles.cache.get(ROLE_IDS.GAMES_ROBLOX);
const ROCKETLEAGUE = interaction.guild.roles.cache.get(ROLE_IDS.GAMES_ROCKETLEAGUE);
const VALORANT = interaction.guild.roles.cache.get(ROLE_IDS.GAMES_VALORANT);
let roles = interaction.member.roles.cache
.filter(COUNTERSTRIKE => COUNTERSTRIKE.id)
.filter(FORTNITE => FORTNITE.id)
.filter(LEAGUEOFLEGENDS => LEAGUEOFLEGENDS.id)
.filter(MINECRAFT => MINECRAFT.id)
.filter(OVERWATCH => OVERWATCH.id)
.filter(RAINBOWSIX => RAINBOWSIX.id)
.filter(ROBLOX => ROBLOX.id)
.filter(ROCKETLEAGUE => ROCKETLEAGUE.id)
.filter(VALORANT => VALORANT.id)
let isCounterstrike = false;
let isFortnite = false;
let isLeagueoflegends = false;
let isMinecraft = false;
let isOverwatch = false;
let isRainbowsix = false;
let isRoblox = false;
let isRocketleague = false;
let isValorant = false;
for(let i = 0; i < value.length; i++)
{
if(value[i] == 'games_counterstrike')
isCounterstrike = true;
else if(value[i] == 'games_fortnite')
isFortnite = true;
else if(value[i] == 'games_leagueoflegends')
isLeagueoflegends = true;
else if(value[i] == 'games_minecraft')
isMinecraft = true;
else if(value[i] == 'games_overwatch')
isOverwatch = true;
else if(value[i] == 'games_rainbowsix')
isRainbowsix = true;
else if(value[i] == 'games_roblox')
isRoblox = true;
else if(value[i] == 'games_rocketleague')
isRocketleague = true;
else if(value[i] == 'games_valorant')
isValorant = true;
}
if(!isCounterstrike)
roles.delete(ROLE_IDS.GAMES_COUNTERSTRIKE);
if(!isFortnite)
roles.delete(ROLE_IDS.GAMES_FORTNITE);
if(!isLeagueoflegends)
roles.delete(ROLE_IDS.GAMES_LEAGUEOFLEGENDS);
if(!isMinecraft)
roles.delete(ROLE_IDS.GAMES_MINECRAFT);
if(!isOverwatch)
roles.delete(ROLE_IDS.GAMES_OVERWATCH);
if(!isRainbowsix)
roles.delete(ROLE_IDS.GAMES_RAINBOWSIX);
if(!isRoblox)
roles.delete(ROLE_IDS.GAMES_ROBLOX);
if(!isRocketleague)
roles.delete(ROLE_IDS.GAMES_ROCKETLEAGUE);
if(!isValorant)
roles.delete(ROLE_IDS.GAMES_VALORANT);
return interaction.member.roles.set(roles)
.then((member) =>
interaction.reply(
{
embeds: [EMBEDS.ROLE_CHANGED],
ephemeral: true,
}))
}catch(err)
{
return interaction.reply(
{
embeds: [EMBEDS.ERROR],
ephemeral: true,
});
}
}
else if(interaction.isStringSelectMenu()) // String Select Menu Interactions
{
try
{
const value = interaction.values;
const COUNTERSTRIKE = interaction.guild.roles.cache.get(ROLE_IDS.GAMES_COUNTERSTRIKE);
const FORTNITE = interaction.guild.roles.cache.get(ROLE_IDS.GAMES_FORTNITE);
const LEAGUEOFLEGENDS = interaction.guild.roles.cache.get(ROLE_IDS.GAMES_LEAGUEOFLEGENDS);
const MINECRAFT = interaction.guild.roles.cache.get(ROLE_IDS.GAMES_MINECRAFT);
const OVERWATCH = interaction.guild.roles.cache.get(ROLE_IDS.GAMES_OVERWATCH);
const RAINBOWSIX = interaction.guild.roles.cache.get(ROLE_IDS.GAMES_RAINBOWSIX);
const ROBLOX = interaction.guild.roles.cache.get(ROLE_IDS.GAMES_ROBLOX);
const ROCKETLEAGUE = interaction.guild.roles.cache.get(ROLE_IDS.GAMES_ROCKETLEAGUE);
const VALORANT = interaction.guild.roles.cache.get(ROLE_IDS.GAMES_VALORANT);
let roles = interaction.member.roles.cache
.filter(COUNTERSTRIKE => COUNTERSTRIKE.id)
.filter(FORTNITE => FORTNITE.id)
.filter(LEAGUEOFLEGENDS => LEAGUEOFLEGENDS.id)
.filter(MINECRAFT => MINECRAFT.id)
.filter(OVERWATCH => OVERWATCH.id)
.filter(RAINBOWSIX => RAINBOWSIX.id)
.filter(ROBLOX => ROBLOX.id)
.filter(ROCKETLEAGUE => ROCKETLEAGUE.id)
.filter(VALORANT => VALORANT.id)
let isCounterstrike = false;
let isFortnite = false;
let isLeagueoflegends = false;
let isMinecraft = false;
let isOverwatch = false;
let isRainbowsix = false;
let isRoblox = false;
let isRocketleague = false;
let isValorant = false;
for(let i = 0; i < value.length; i++)
{
if(value[i] == 'games_counterstrike')
isCounterstrike = true;
else if(value[i] == 'games_fortnite')
isFortnite = true;
else if(value[i] == 'games_leagueoflegends')
isLeagueoflegends = true;
else if(value[i] == 'games_minecraft')
isMinecraft = true;
else if(value[i] == 'games_overwatch')
isOverwatch = true;
else if(value[i] == 'games_rainbowsix')
isRainbowsix = true;
else if(value[i] == 'games_roblox')
isRoblox = true;
else if(value[i] == 'games_rocketleague')
isRocketleague = true;
else if(value[i] == 'games_valorant')
isValorant = true;
}
if(!isCounterstrike)
roles.delete(ROLE_IDS.GAMES_COUNTERSTRIKE);
if(!isFortnite)
roles.delete(ROLE_IDS.GAMES_FORTNITE);
if(!isLeagueoflegends)
roles.delete(ROLE_IDS.GAMES_LEAGUEOFLEGENDS);
if(!isMinecraft)
roles.delete(ROLE_IDS.GAMES_MINECRAFT);
if(!isOverwatch)
roles.delete(ROLE_IDS.GAMES_OVERWATCH);
if(!isRainbowsix)
roles.delete(ROLE_IDS.GAMES_RAINBOWSIX);
if(!isRoblox)
roles.delete(ROLE_IDS.GAMES_ROBLOX);
if(!isRocketleague)
roles.delete(ROLE_IDS.GAMES_ROCKETLEAGUE);
if(!isValorant)
roles.delete(ROLE_IDS.GAMES_VALORANT);
return interaction.member.roles.set(roles)
.then((member) =>
interaction.reply(
{
embeds: [EMBEDS.ROLE_CHANGED],
ephemeral: true,
}))
}catch(err)
{
return interaction.reply(
{
embeds: [EMBEDS.ERROR],
ephemeral: true,
});
}
}
i would recommend checking out how .map and .filter work, its basic js
I literally told yoy what you had to do yesterday, yet you still are doing some weird stuff you dont even have to
ok
what are you trying to accomplish
trying to make the select menu remove all roles that the menu offers from the user, then add the roles they selected
which you can do by passing in an array in .set
containing their current roles and the updated ones
I told you how you'd do it yesterday
but how would i get an array of roles?
cache doesnt give an array
by mapping
As i told you yesterday
i mapped here
but someone told me not to and to remove it
Mapping, again, is basic js
honestly
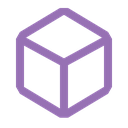
i never learned js too well
i know Java and C++
djs requires a solid js understanding
#resources #rules 3
yeah . . . . i realized