✅ How to find objects that changed?
I have following records:
Than I create lists with objects and trying to find objects that was changed. I don't know why but
changedItems.Count
is 1
20 Replies
but actually objects are the same
What do you mean by objects that changed
The Join call produces a list with one element, I think because it's doing the equivalent of an inner join
What is your goal with these two lists? Their contents seem to be identical except the objects will not point to the same reference
the
HashSet<T>
s use the default Equals()
check, which is a reference equality checkcap5lut
REPL Result: Success
Console Output
Compile: 580.747ms | Execution: 107.539ms | React with ❌ to remove this embed.
long story short, u would have to provide ur own implementation of
bool Equals(object? other)
for NotificationSettingsDto
.
which means u have to rewrite it as normal class as u can not override that method
(note that u have to override int GetHashCode()
as well then)
oh, and this is also the case for most other reference types (especially collections)oh no
Ok, I will try to write my own implementation of
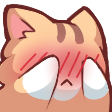
GetHashCode
and Equals
dotnet provides a helper struct for the hash code: https://learn.microsoft.com/en-us/dotnet/api/system.hashcode?view=net-8.0
but it will still be tricky because of the hash set. even with the same contents, they could have a different order which u will have to account for
is it correct?
hmm doesn't work
as expected for me haha
if the underlying arrays of the hash sets have a different size, it can affect the buckets and thus the order in which the items are stored.
so eg if u have an hashset A and B and they both contain only "hello" and "world", it could be that on iteration one would yield "hello" first, while the other yields "world" first
thus
SequenceEqual
wont be enough eitherok I will use List instead 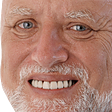
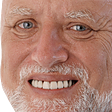
u would have to do something like
to test if they contain the same elements
for the hash code u would have to sort the elements somehow and use that to compute the hash code
I don't think it is optimal solution
Imagine doing this in each
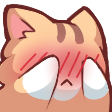
Equals
or u use a
SortedSet<T>
, then u can use at least SequenceEqual
and alikenow it works:
thanks for helping
im pretty sure the hashcode is still wrong
cap5lut
REPL Result: Success
Console Output
Compile: 458.462ms | Execution: 31.802ms | React with ❌ to remove this embed.
u would have to do something like
done!
I hope it's optimal way to get hashcode
its definitifely the recommended way ;p
but now that i think about it, it might also be okay to just use the
NotificationSettingsId
for the hash code
the contract is basically, if 2 objects of the same type yield true
for Equals()
, they have to have the same hash code,
but not vice versa, meaning two objects producing the same hash code, do not have to return true
for Equals()
thanks for helping bro 
