springframework.validation.BindException: Date
12 Replies
⌛
This post has been reserved for your question.
Hey @' .•TIP: Narrow down your issue to simple and precise questions to maximize the chance that others will reply in here.KIΛ.RΛR ’•. '! Please use
/closeor the
Close Post` button above when your problem is solved. Please remember to follow the help guidelines. This post will be automatically closed after 300 minutes of inactivity.
what i want: datepicker sends ajax request on changing the date to reload the displayed data, but when i change the date an error is thrown.
demo project to showcase my problem: https://github.com/DragonCat4012/web-thymeleaf
GitHub
GitHub - DragonCat4012/web-thymeleaf
Contribute to DragonCat4012/web-thymeleaf development by creating an account on GitHub.
like in the javascript function it always prints the current date, even when i change the date in the picker it wont change anything =.=
Have you tried parsing your string using
I have a feeling this won't work because the string looks sketchy in regards to the format pattern
(I sadly can't try it rn)
an welcher stelle O.o problem ist spring sagt das sobald ich das sobald ich nen datum gesetzt hab:
ich komm im java nciht mla zu irgendeinem print in der mapping oder der setter methode ;-;
in der url hab ich als wert das ausgewählte datum als paramter wies sein soll ;-; nur ist die seite halt put dann
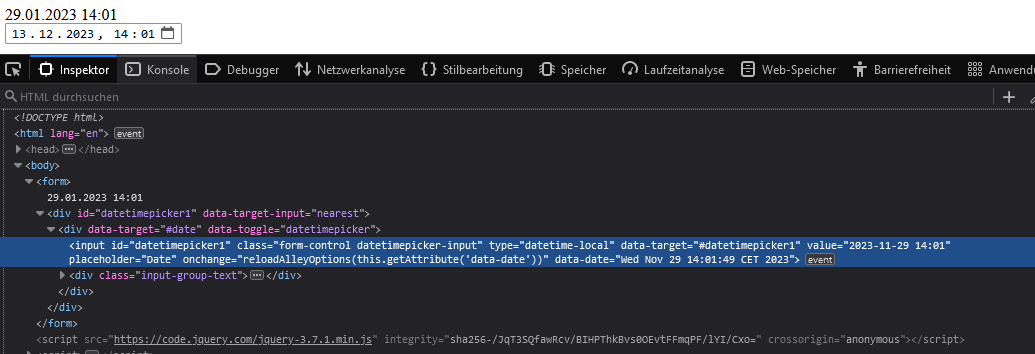
dafuq the datepicker shows a different date then its value?
Your ReservationForm specifies a datetime pattern in
@DateTimeFormat
decorator
I doubt your pattern would work considering "2023-11-29T11:20:17.719199" as possible inputyes?
yeah ik but i dont know how to change it since thymeleaf does its shit wjho knows where in the background
the correct format would be something along the lines of
yyyy-MM-dd'T'HH:mm:ss.SSSSSS
@' .•` KIΛ.RΛR ’•. '
that should at least make it parse correctly💤
Post marked as dormant
This post has been inactive for over 300 minutes, thus, it has been archived.
If your question was not answered yet, feel free to re-open this post or create a new one.
In case your post is not getting any attention, you can try to use /help ping
.
Warning: abusing this will result in moderative actions taken against you.