Why isn't the autocomplete working the autocomplete function isn't even getting called.
Why isn't the autocomplete working the autocomplete function isn't even getting called.
Code:
21 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!
- ✅
Marked as resolved by staffWhere do you call autocomplete()?
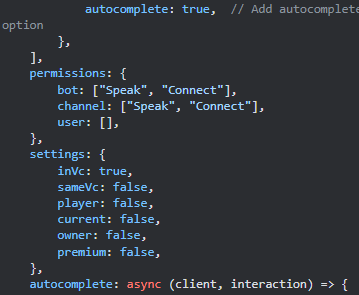
That’s where you define it
You should be calling it somewhere in your interaction handler.
When you receive an autocomplete interaction
isn't the
autocomplete: async (client, interaction)
supposed to do that?That’s where the logic is
How and when does that code run?
You should be calling the function somewhere.
Otherwise it’s just defined and unused
this loads the commands
Do you have an interaction create event listener?
no
but it works exept the autocomplete
What works? Logging in? That’s all I see that could be working.
And don’t redeploy commands in the ready event.
You only need to deploy them when you change a commands parameters, add, or remove a command.
This is probably a good section to review.
here's the error
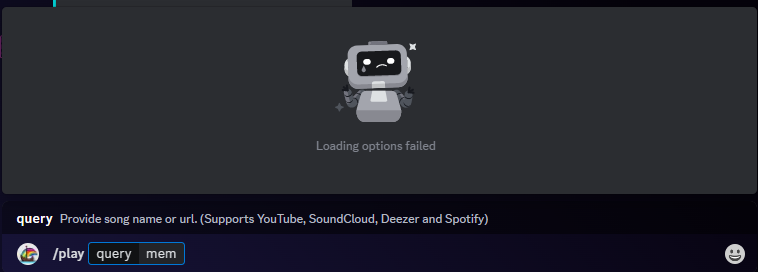
Again you’re not responding to the interaction. Does any other command work?
every command work exept the autocomplete
So show your interaction create event handler
to be honest i'm not the one who wrote the code and the person who wrote the code made it so hard to find a single piece of code. there's like 100 files of stuff in this
GitHub
GitHub - adh319/Lunox: Simply powerfull Discord Music Bot that used...
Simply powerfull Discord Music Bot that used Poru Lavalink client & DiscordJS v14 - GitHub - adh319/Lunox: Simply powerfull Discord Music Bot that used Poru Lavalink client & DiscordJS v14
i even wrote two sperate commands and put it in the commands/slash folder and they just worked
Autocomplete interactions are a little different. They require you to handle them separately and use respond() to send the list of options to discord.
If you have an event handler you may have a separate interactionCreate file that handles executing commands. You would need to handle the autocomplete interactions in there.