Code Review (Web Api)
Is this a proper set up for a web api project? If not, what should I change?
https://pastebin.com/V4ky2YXX
25 Replies
You can rename the route to [Route("api/["controller"])] it gets the name of the controller from the filname e.g. FacilityController -> facility (it slices the controller from the name)
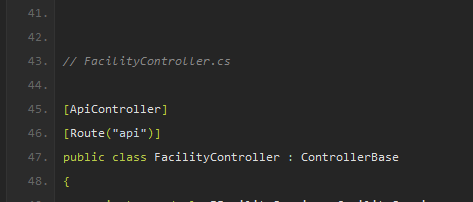
other then that it looks fine from my pov
This basically lets you avoid the repetetive code here
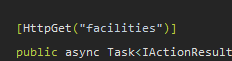
Gotcha, thanks @frostbyte. I should have mentioned, I'm more interested in if my pattern and the way I'm doing things is proper.
I'm aware I probably need to handle a bit more like if GetAllFacilities() is empty, etc what to return, and so on. But just curious about my set up before I continue on with the whole project with this format.
yeh the pattern looks great
youve got abstraction from the controller and business logic which is good
Is
service
for all this correct, or is it more repository
? I always get confused because sometimes I swear I see the names used interchangably, and other times I see both services and repositories.It would usually be called Repository
The service would be the api itself
Ok so it would make more sense to rename everything with repository in place of service?
Yeh so rename to IFacilityRepository and FacilityRepository
Ok thank you! Why do some projects have both though? A service layer and a repository layer?
Its usually related to CQRS , so a seperation of concerns. The service layer is responsible for containing the business logic and application-specific functionality. So like any service to service communication etc. The repository layer is responsible for data access and persistence
This post on SO makes it seem like what I have
service
is more correct: https://stackoverflow.com/questions/1440096/difference-between-repository-and-service
But idk
ok yeah so what that post says
Maybe i'm interpreting it wrong then.What you are doing in ur files is communicating with database which is usually in the repository layer. Since ur accessing and manipulating data.
Ah ok thank you that is helpful.
Any kind of communication with the database read and write happens in Repository
Ok I'll go and rename it all to Repository.
I think he kinda worded it wrong... its kinda misleading. I think he means that the service the (api) calls the repository methods... its kinda wrong
Yeah i was quite confused lol. So in a web api project, let's say what I have is the repository layer, what would be an example of service?
Common web application architectures - .NET
Architect Modern Web Applications with ASP.NET Core and Azure | Explore the common web application architectures
Read up on this as well 😉
Will do, thank you!
RabbitMQ configuration,Any kind of messaging services
MassTransit
Those would be in a seperate project in a class library
Gotcha, ok. So like an actual "service".
Yes
Cool thanks i think this is good, ill read the link 🙂
Frost, additionally, since you already know my set up, I don't think my Unit tests make sense. For example I am doing:
but if i changed this line to:
var result = await _facilityController.GetCitiesAsync("NYC");
it still passes, when I would think it shouldn't...
I can do more reading on my own, but just if you're interested and still around. No worries.