How to swap between 3 embeds using buttons?
I would like to swap between 3 different embeds when a button is pressed. So say embed1 is displayed, then button2 would change it to embed2 and button3 would change it to embed3. Or if embed2 is displayed, then button1 would change it to embed1 and button3 would change it to embed3. But the button for the current embed is not displayed.
I'm not sure on the fundamentals of how to do this so I would really appreciate it if I could have some skeleton code on how something like this would function.
Thanks very much!
10 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!
- ✅
Marked as resolved by OPuse the customId of the button and map it to the embed you want to display
ex embed1, embed2
Yeah the part I am confused on is how to change the message to another embed once the button has been pressed
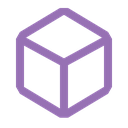
I've got the embed updating once but I'm not sure what I need to do so they can switch back and forth multiple times
Here's the skeleton code of what I have currently
use the non promisified collector
I now have it swapping back and forth but each time I click the button it still says ... for 3s and interaction failed, makes me think I'm not "acknowledging" the interaction or something
you do have to reply to the interaction
and you can replace the msg.edit with <Interaction>.update
That works perfectly, thank you very much for all your help