Delete slash command if it is "forgotten"
I have a problem with my guilds slash commands where If I delete a command in my code, it stays in the guild. Now I'm trying to code something to delete the slash command if it doesn't "exist" anymore in the code, but I'm getting an error. Here's my output:
The code to delete the slash command is the following:
Can't I use the command interaction ID to delete it?
30 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!
- ✅
Marked as resolved by staff[email protected] and node v20.3.0
I just use set as opposed to create when deploying commands to avoid the need for deleting commands
Well, put instead of post if using rest
Wdym?
Set?
Put instead of post
Oh, what's the difference?
It's temporary maybe?
put sets alls commands
Oh yeah
Whatever array of commands you provide will become the entire set of commands (new ones are created, removed ones are deleted, others are updated if needed)
The creates are still counted against the creation rate limit, but the rest isn’t
Heh, rest
I already use put lol
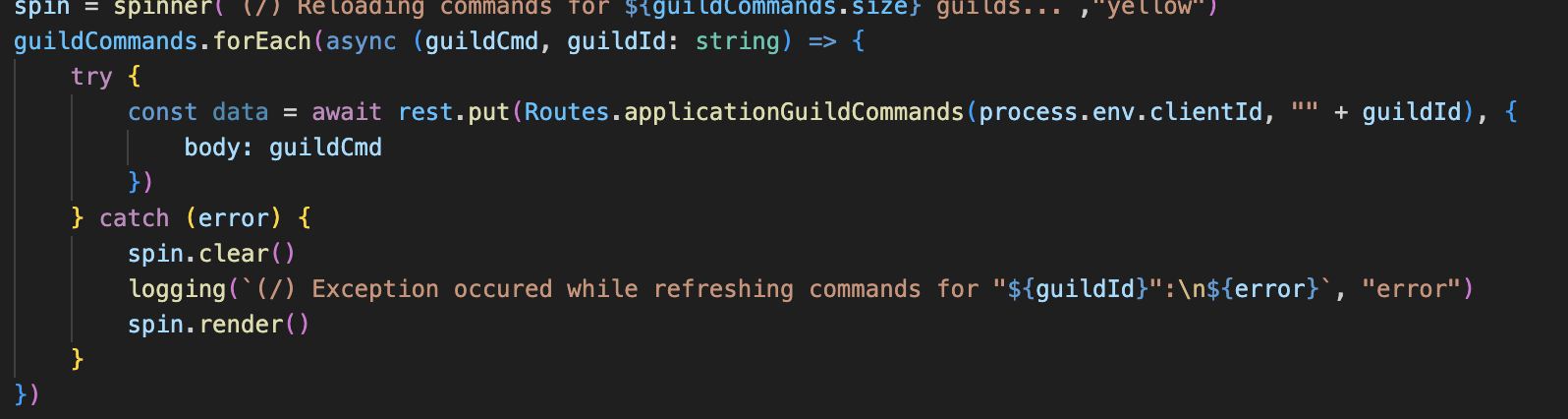
Wait maybe i'm getting old commands
Try running the old command
It shouldn’t go to the bot
Well it says the message
wait

Yea
I'm maybe guessing that i'm getting old commands before pushing
Is that from ur bot?
Yes
(dont mind the name it's not the actual bot lol)
Are you sure the command got removed from the array?
I'll try logging it
Oh yeah also
If I remove every guild commands then the guild deploy part will not run
and therefor not overwrite the old guild commands
that's why
Yeah now it works
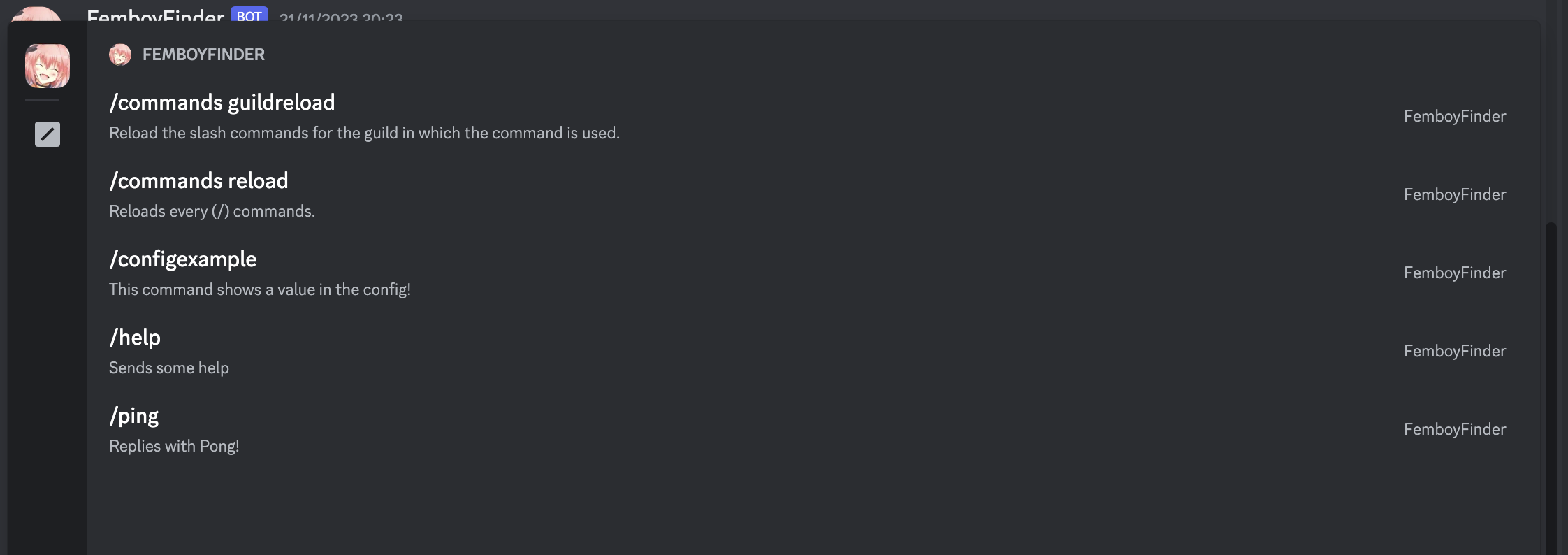
When I add a guild command
You don’t manually run the deploy script while testing?
what do you mean?
Usually, application command deployment is its own script
Oh yes it is its own script
What I mean is that If I delete all guild commands, the part where it deploys guild commands will not run, so it will not overwrite the old commands
That's why I wanted to delete the command if it was old
I’m not rly sure what your mean
My deploy script just reads the commands directory to see available commands, then sets the commands to match that
Yes but I'm talking about guild-specifc commands
My script is halfed into global, and guild commands.
The global deploy always run.
The guilds one only runs if one command in the code is guild-specific
I could send it If you want
I would just have it always deploy
Even if there are no commands
I have to go but I'll try to explain it better later
If there is no guilds in which you need to reload commands, then the code doesn't know which guilds you need to push commands to