Db Connection
Good afternoon guys, I need some help setting up the database connection for my project.
I have the knowledge to do it in an amateur way, but a few months ago I started a course and I feel that things are evolving, and in this course we haven't gotten to the implementation of the Db in the project, and to test and get ahead I've been trying to figure out the best way to do it.
My project is divided into
- Application (Services/Dtos/Contracts).
- Domain.
- Infra (Data/IoC/Contracts).
- Presentation (Asp.Net MVC).
The big question for me is how I'm going to make the connection and where.
I don't intend to use the Entity Framework just yet
I'd be grateful if anyone could shed some light on this.
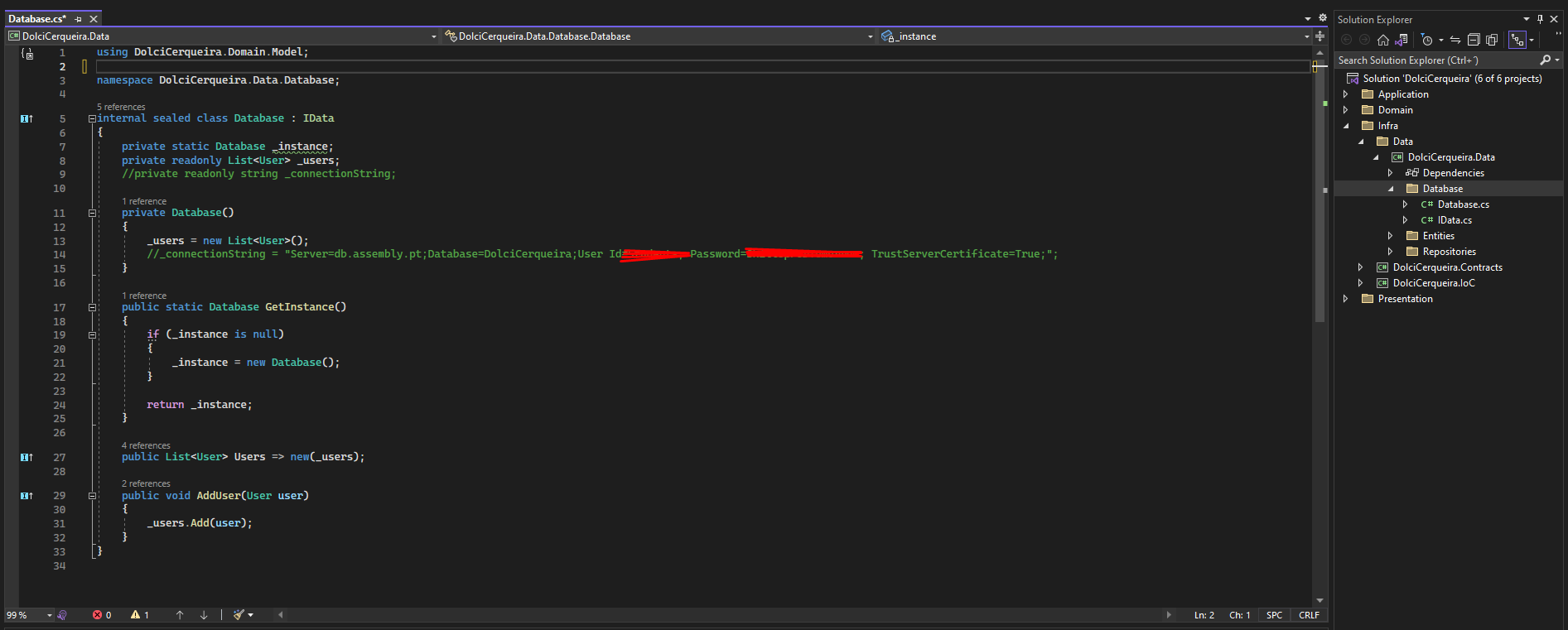
2 Replies
What database are you attempting to connect to. Usually there'll be a database provider that handles communication with whatever your db is. e.g. for postgres, it's https://www.npgsql.org/
Expanding on this, some sample code for creating a db connection on this:
The connection string ideally should be securely stored. There's some additional info on exactly how to do that here There are cloud secrets managers like AWS Secrets Manager, there's
dotnet user-secrets
, there's Environment Variables, the least "secretive" next to hardcoding it would be storing it in appsettings.json (This is generally discouraged)
If you're not using Entity Framework you could look into Dapper