Voice player sometimes doesn't start playing music
Hi, like the title says, the voice player randomly doesn't start playing music
The code responsible for it is the boilerplate from https://discordjs.guide/voice/audio-player.html#playing-audio modified only by a 20sec timeout which just disconnects the bot:
Relevant package versions:
"@discordjs/opus": "^0.8.0",
"@discordjs/voice": "^0.13.0",
"ffmpeg-static": "^5.1.0",
"libsodium-wrappers": "^0.7.10",
"node-opus": "^0.3.3",
"opusscript": "^0.0.8",
Any leads would be appreciated and in advance I'm sorry for the incomplete info, i can't run it in debug mode right now
6 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!i thought about that overlap being the case yeah
but it doesn't happen often enough as far as i know
it would send a message twice within that 20 seconds for example if that timeout was destroying new connections i think
yeah, sorry about that
are we on the same page on what that would be supposed to be doing?
as in if you called all of it twice within 20 seconds, the first timeout would destroy the second
look through the bot's messages but i doubt that ever happens
is there something else that could be bricking it?
there's a few opus libraries installed for example, i'm not sure if they interfere with each other
I can share it but there's nothing past the boilerplate and what I sent as far as voice goes
one sec
I just looked through its logs and the shortest period between these calls was like 3 minutes
yes it's only a part of one server. there just wasn't much point in having the bot there throughout the game
it could just sit there and play/pause each time but does that change anything?
oh, and it doesn't leave the channel, just joins and doesn't play anything so that rules out the overlap
i'm waiting for log access, would be a bit easier 😐
Nope, that's the bug behavior
or in other words it's coded to join, play, and then leave in more or less 20 seconds
but it sometimes just joins and doesn't play music
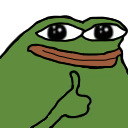
Doesn't <Connection>.destroy() do exactly that? https://discordjs.guide/voice/voice-connections.html#deletion
discord.js Guide
Imagine a guide... that explores the many possibilities for your discord.js bot.
from what i understand, disconnect is very similar
just lets you rejoin without having to instantiate a new connection
there's a new player being created too so it's not just the song ending (the mp3 file is an hour long i think)
probably didn't want to bother with cutting it
i'm a co-dev on it
that's true, but if something else is already scheduled there, may as well
do you know if any errors would be logged without the debug mode enabled for both?
rip
i've also been told just now that the bot only does that whenever there's more people playing (more people on the voice channel)
there wouldn't be any extra intents, verifications or anything like that needed by default right?
oh yeah you can't, we have that
why though?
wait, how come
it's only playing in one voice channel
and the initial play is triggered from a web panel which doesn't happen often enough
it is the bot's code, just called elsewhere
and the overlap is a non issue because of this
not quite, the bot is just controlled in some part from that panel
i.e. the function i sent doesn't get called anywhere within the bot, it's just exposed
it's all 1 process as far as i know, the bot being imported to an express server
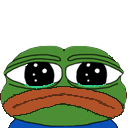
hopefully unrelated, but I noticed some of your deps, including
@discordjs/voice
, appear to be outdated
even if it doesn't fix this particular issue, I'd recommend updating to avoid some other issuesyep, will do. thanks
okay, a bit more context this time
this is what it logged at least twice