Waiting for user message in DM.
I've been trying to get the bot to wait for a message but it keeps logging this error
Here is my code.
I'd love a few pointers of where im going wrong <3
9 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!
- ✅
Marked as resolved by OPOh also! Feel free to ping me, It's the best way to get my attention 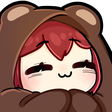
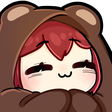
@Kira Kenjiro
Your attempt to utilize awaitMessages on interaction.channel is problematic because awaitMessages is not a method directly accessible in this context. You can try this awaitMessageComponent
ohh i see :O
okay okay i thought it was
thanks kern, I'll take alook at the component version
DM channels aren't cached by default. You have to fetch it.
interaction.user.createDM()
He need to collect messages, not message component interactionsoh you are right
Oh yeah! ok got it
i thought they we're also cached like normal channels
I am now a little lost..
I've gotten here, I've got the filter and options setup but eahm would i do something along the lines of
userDM.interaction.awaitMessages()
I'm using this but, I don't think thats how i should be doing it as it won't return the content! But.. it also doesn’t error which, isn’t helpful
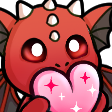
Tag suggestion for @Kira Kenjiro:
The filter for collectors has moved into the options:
Ohh! okay okay i got it, Thanks Sy <3
Yeh!! it's working now <a:pats_neko:1096725152114429962>
Thanks for the help I really appreciate it