"The script will never generate a response"
I have no idea where to even start looking, or even how to reproduce.
I read this article https://zuplo.com/blog/2022/03/04/the-script-will-never-generate-a-response and I think this may be what is going on. But I have no idea what can I do to start troubleshooting.
I am using Sveltekit and in
hooks.server.ts
I validate a session cookie and get/put data using Cloudflare's KV.
I do notice if I refresh the page several times I can see the error in the logs:
"exceptions": [
{
"name": "Error",
"message": "The script will never generate a response.",
"timestamp": 1700096259217
}
],
"exceptions": [
{
"name": "Error",
"message": "The script will never generate a response.",
"timestamp": 1700096259217
}
],
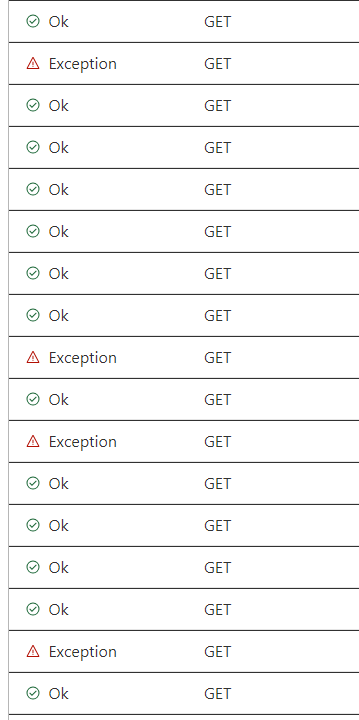
2 Replies
Do you have an example of your code that has this issue?
Hi @kian , this is the code in handle function:
I am noticing that this error occurs when I quickly reload the page. This error also happened on another project, and it seems to happen when something is being fetched by the load function.
import { handleSessionIdleState, validateSession } from "./cookie-manager";
import { redirect, type Handle } from "@sveltejs/kit";
import { PUBLIC_SESSION_COOKIE_NAME } from "$env/static/public";
import type { SessionData } from "../../login/proxy+page.server";
const config = {
loginRouteId: "/(public)/login",
loginPagePath: "/login"
};
type SessionStatus = "expired" | "active" | "idle" | undefined | null;
import { handleSessionIdleState, validateSession } from "./cookie-manager";
import { redirect, type Handle } from "@sveltejs/kit";
import { PUBLIC_SESSION_COOKIE_NAME } from "$env/static/public";
import type { SessionData } from "../../login/proxy+page.server";
const config = {
loginRouteId: "/(public)/login",
loginPagePath: "/login"
};
type SessionStatus = "expired" | "active" | "idle" | undefined | null;
const validateAndUpdateCookieSession: Handle = async ({ event, resolve }) => {
const kv = event.platform?.env?.AUTH_SESSIONS;
const cookieValue = event.cookies.get(PUBLIC_SESSION_COOKIE_NAME);
const storedSessionData = await kv?.get(String(cookieValue));
if (!storedSessionData) {
console.log("No session data found");
event.locals.sessionState = null;
return await resolve(event);
}
const storedSession = JSON.parse(storedSessionData);
let cookieSessionState: SessionStatus = await validateSession(
storedSession?.cookieExpirationDate
);
event.locals.sessionState = cookieSessionState;
switch (cookieSessionState) {
case "active":
break;
case "expired":
event.cookies.set(PUBLIC_SESSION_COOKIE_NAME, "", {
secure: false,
sameSite: "lax",
httpOnly: true,
path: "/",
domain: undefined,
maxAge: 0
});
break;
case "idle":
const { newCookie, newExpirationDate } = handleSessionIdleState(String(cookieValue));
const data: SessionData = { ...storedSession, cookieExpirationDate: newExpirationDate };
await kv?.put(newCookie.value, JSON.stringify(data));
event.cookies.set(newCookie.name, newCookie.value, newCookie.attributes);
break;
default:
break;
}
return await resolve(event);
};
const validateAndUpdateCookieSession: Handle = async ({ event, resolve }) => {
const kv = event.platform?.env?.AUTH_SESSIONS;
const cookieValue = event.cookies.get(PUBLIC_SESSION_COOKIE_NAME);
const storedSessionData = await kv?.get(String(cookieValue));
if (!storedSessionData) {
console.log("No session data found");
event.locals.sessionState = null;
return await resolve(event);
}
const storedSession = JSON.parse(storedSessionData);
let cookieSessionState: SessionStatus = await validateSession(
storedSession?.cookieExpirationDate
);
event.locals.sessionState = cookieSessionState;
switch (cookieSessionState) {
case "active":
break;
case "expired":
event.cookies.set(PUBLIC_SESSION_COOKIE_NAME, "", {
secure: false,
sameSite: "lax",
httpOnly: true,
path: "/",
domain: undefined,
maxAge: 0
});
break;
case "idle":
const { newCookie, newExpirationDate } = handleSessionIdleState(String(cookieValue));
const data: SessionData = { ...storedSession, cookieExpirationDate: newExpirationDate };
await kv?.put(newCookie.value, JSON.stringify(data));
event.cookies.set(newCookie.name, newCookie.value, newCookie.attributes);
break;
default:
break;
}
return await resolve(event);
};
const handleLogin: Handle = async ({ event, resolve }) => {
const sessionStatus: SessionStatus = event.locals.sessionState;
console.log("Session Status", sessionStatus);
if (!sessionStatus) {
if (event.route.id?.includes(config.loginRouteId)) {
// Continue with the request resolution
return await resolve(event);
} else {
throw redirect(303, config.loginPagePath);
}
}
if (
(event.route.id?.includes(config.loginRouteId) && sessionStatus === "active") ||
sessionStatus === "idle"
) {
throw redirect(303, "/");
}
return await resolve(event);
};
const handleLogin: Handle = async ({ event, resolve }) => {
const sessionStatus: SessionStatus = event.locals.sessionState;
console.log("Session Status", sessionStatus);
if (!sessionStatus) {
if (event.route.id?.includes(config.loginRouteId)) {
// Continue with the request resolution
return await resolve(event);
} else {
throw redirect(303, config.loginPagePath);
}
}
if (
(event.route.id?.includes(config.loginRouteId) && sessionStatus === "active") ||
sessionStatus === "idle"
) {
throw redirect(303, "/");
}
return await resolve(event);
};
const injectLocals: Handle = async ({ event, resolve }) => {
const sessionStatus: SessionStatus = event.locals.sessionState;
if (!sessionStatus || sessionStatus === "expired") {
return await resolve(event);
}
const kv = event.platform?.env?.AUTH_SESSIONS;
const cookieValue = event.cookies.get(PUBLIC_SESSION_COOKIE_NAME);
const storedSessionData = await kv?.get(String(cookieValue));
const { loginData } = JSON.parse(storedSessionData as string);
event.locals.loginData = loginData;
return await resolve(event);
};
const injectLocals: Handle = async ({ event, resolve }) => {
const sessionStatus: SessionStatus = event.locals.sessionState;
if (!sessionStatus || sessionStatus === "expired") {
return await resolve(event);
}
const kv = event.platform?.env?.AUTH_SESSIONS;
const cookieValue = event.cookies.get(PUBLIC_SESSION_COOKIE_NAME);
const storedSessionData = await kv?.get(String(cookieValue));
const { loginData } = JSON.parse(storedSessionData as string);
event.locals.loginData = loginData;
return await resolve(event);
};
export const AuthHooks = [validateAndUpdateCookieSession, handleLogin, injectLocals];
export const AuthHooks = [validateAndUpdateCookieSession, handleLogin, injectLocals];