Unable to Promote or Demote users
I have buttons to promote and demote user roles, however they are currently doing nothing.
83 Replies
define "doing nothing"
it is not calling the function
how do you know?
I added Console.WriteLine to the functions and got nothing back
that proves nothing
set a breakpoint
I did also do that, it never got triggered
at the beginning?
at the start of the Promote and Demote functions
how about
OnInitializedAsync()
?Ill give it a shot, see how it goes
aside from the breakpoint being triggered when I load the page, nothing else
according to docs, your syntax is wrong
ASP.NET Core Blazor event handling
Learn about Blazor's event handling features, including event argument types, event callbacks, and managing default browser events.
As in
@onclick="() => DemoteUser(user.UserId)"
to
@onclick="@(() => DemoteUser(user.UserId))
that is what the docs suggest, yes
It does nothing
as in, it does not fix the problem. I had tried it earlier as wewll
or
@onclick="@((e, args) => DemoteUser(user.UserId))"
e args causes a build issue
just using e also does not fix anything
try just
@onclick="OnButtonClick"
with a new function with no argsI did try that
does the function get called?
no
let's see the whole file
This is the file
add the following imports
I have them all except for
@using Microsoft.AspNetCore.Authorization
I mean
no
you don't
except for
Microsoft.AspNetCore.Authorization
which you DO already haveThis is what is in my _Imports.razor file
I have not modified this file at all
add them to your component file, for good measure
Every single one of them come up as unusued
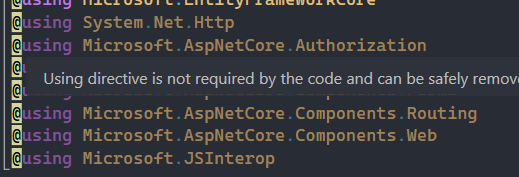
that's a lie
at least it was last time I used blazor
Adding them to my
@page "/Admin/ManageAdmin"
did nothingk
The _Imports files deals with them
yes, I know
welp, I'm out of ideas
go back to a fresh sample template that works and try and figure out what you're doing differently
The defualt Counter page works fine
within this same app?
Not really, thats in the .Client app
Though they share the same imports
....and this isn't?
It is in my main one
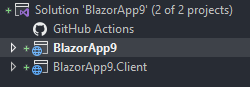
what is that supposed to mean ?
see pic above
so....
what?
what is that supposed to tell me?
idk, the fact @onlick works in my application, but not in the webpage I want it to work in
that makes no sense
what doesn't make sense?
what is your definition of "application" and "webpage"
cause those are the same thing
app is the project, webpage is the single page I am having issues with
so
it doesn't work in your application
because the page is in your application
Sure, all I know is @onclick in Counter works in the .Client project, but does not work in my server project
why do you have a server project?
why are you putting client stuff in your server project?
why is anything that's working in the client project relevant to what works in the server project?
go back to a fresh sample template that works and try and figure out what you're doing differently
this project is about as fresh as they come
you've changed something
yeah, added external auth and users to the db
nothing I have changed should even be close to whatever is affecting this
haven't touched imports
something is
and it's something you changed or added
Aight, this is what I have changed in my Program.cs
Added google auth
added AddRoles<..>()
start removing those things until your blazor code starts working
Code to seed users (this works)
or go back to a blank template and start adding things incrementally until it stops working
if it helps this is a .NET 8 project
oh oh oh
I think I know what might be doing it in
I don't think I broke it. I think it was broken to begin with
My Server project has no
_framework/blazor.web.js
In hindsight, I think what I mentioned above is wrongwhich part?
about the _framework
cause my .Client doesn't have it and the @onclick in there works fine
it's definitely present in a fresh template
where this works fine, BTW
FYI this is my setup for the project
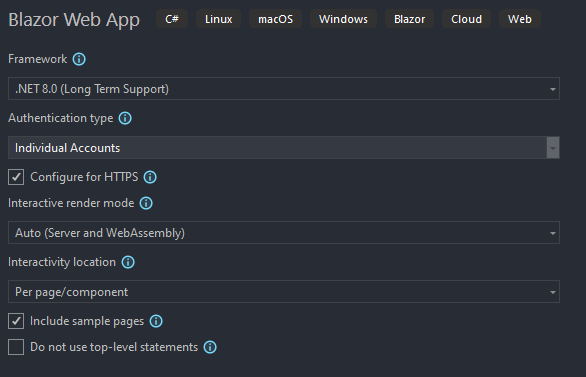
so, yeah, I'm gonna re-iterate my recommendation again
okay
see
that's what you should lead with
gonna be honest, though I had. mb
you said ".NET 8.0" and "Blazor"
and then we established later that this is "server"
this is in fact not Blazor Server, it's the whole new deal
ahh didn't realise
so, now we go back to my very first question
rather, my very first response
that proves nothing set a breakpointsetting a breakpoint in your server code proves nothing your server code IS NOT WHAT'S RUNNING not when you click the button
So do I have to move at least the button to inside .Client?
Or is it still possible to achieve it the way I first intended?
the whole point of "Interactive render mode" of "Auto (Server and WebAssembly)" is that pages are rendered server-side, on startup, but rendering transitions over to the client, as soon as the app bootstraps
so, yes
a button handler in server-side code is nonsense
so stick the button handler in the client side, then put the functionality in the server side?
which functionality?
the only functionality that should exist server-side is what needed for startup
Stuff like this Or does all of that go into my client as well?
ASP.NET Core Blazor render modes
Learn about Blazor render modes and how to apply them in Blazor Web Apps.
I suppose I could always set up services and controllers to manage endpoints that achieve the same thing. Have my client call the endpoints
Sense has been made
more likely, it seems like you have not defined this component as interactive
This is in my server side Program.cs
Components using the automatic render mode must be built from a separate client project that sets up the Blazor WebAssembly host. You can't switch to a different interactive render mode in a child component. For example, a Server component can't be a child of a WebAssembly component.
ASP.NET Core Blazor render modes
Learn about Blazor render modes and how to apply them in Blazor Web Apps.
does that mean I can't have the client call endpoints that are located in the server?
cause thats what I am probably gonna try when I get back
that is not what it means
ok good, just wanted to make sure