✅ Formatting A Phone Number
I'm trying to create a function that will format a universal phone number. What I mean is if the user saves an australian phone number, then when the program goes to display that phone number it will format it appropriately, the same for other countries and american numbers too. I asked ChatGPT and it gave me
this code, but I'm not sure if it's what I'm looking for. Any tips? Thanks
50 Replies
i personally use a C# port of google's
libphonenumber
for this kind of thingGitHub
GitHub - twcclegg/libphonenumber-csharp: Offical C# port of https:/...
Offical C# port of https://github.com/googlei18n/libphonenumber - GitHub - twcclegg/libphonenumber-csharp: Offical C# port of https://github.com/googlei18n/libphonenumber
ok. illl have to do some messing around with that to figure out how to use it
it might be overkill for your application, but i also validate phone numbers (as much as possible without actually calling them) and there's no way i'm maintaining that myself 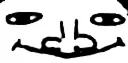
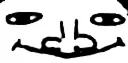
well it may be a bit overkill. I was just looking for a way to format the numbers when displayed. I'm building a phone book application. The home screen shows all the user's stored contacts and I'm making a helper function to format the phone numbers as they're pulled from the database before being displayed. So like if they stored US phone numbers, it would format to
+1 (123) 456-7890
. If they stored an australian phone number then it would format and display like +61 (02) 123-4567
and same for like a British number +44 20 7123 4567
so basically no matter what the prefix is for the phone number, it would take that prefix, determine the necessary format for that countries phone number, and then format it appropriately.
As per validation, that will happen on another screen before storing the number in the database. I need that too so that the user can only store valid phone numbersphone numbers, email addresses, street addresses, etc, they all suck at the global scale.
this library is actually pretty neat and does what I need it to, however, I just may need a bit of help getting to automatically determine how to format. So like an example
with this method, I would have to manually insert
"CH"
and INTERNATIONAL, NATIONAL, or E164
for formatting the number. Is there a way I can have this library, or create a helper function to, determine the phone numbers originality whether the user enters the numbers prefix +41
or not?
like in that example, the +41
doesn't exist, but they used "CH"
to get the origin of the number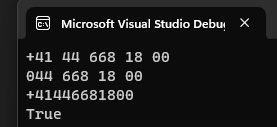
so it used the
"CH"
to determine the numbers prefix. How could I get the program to do that automatically. Let's say I entered a US phone number (123) 456-7890
. How could I get the function to recognize that it needs to be done like phoneUtil.Format(phoneNumber, "US")
without having to manually put in the "US"
part?I mean, this is why folks put a drop down on phone number entry
for country
you could have a dictionary, if I'm reading what you're asking correctly
I could do a drop down. That's not a bad idea, but then I would have to look up and manually type out every countries prefix
Mayor McCheese
REPL Result: Success
Console Output
Compile: 624.913ms | Execution: 75.970ms | React with ❌ to remove this embed.
that's not a bad idea honestly
you can json serialize a dictionary
alternatively you can use "smart enums"
the only downfall to doing the dictionary is if the user doesn't enter a prefix, but I could always make that a required selection from a drop down. Just finding a library that contains all the countries prefixes would be beneficial to that dictionary
There's some fancy packages that make smart enums
I think it would be easier if I had the user select the numbers prefix from a list tbh
yep
so like they would select the prefix from the list, and then I would use that prefix against the dictionary to pull the country 2 letter code for the format
so now to create a function to generate a dictionary of all countries codes and prefixes
https://stackoverflow.com/questions/34837436/uwp-get-country-phone-number-prefix#:~:text=Some%20copy%2Dpasta%20and%20little%20language%20changes%20later%2C%20we%20have%20the%20following%20code%3A
I found this, thoughts?
Stack Overflow
UWP get country phone number prefix
I want to get the country prefix for phone numbers.
e.g. if I am in the US and I enter the number 0123 - 456, I want to get the prefix +1 or 001, so the number in the end is (+1) 0123 - 456
If I a...
if I use that list, I can just pull those prefixes from that dictionary and display them and use them for the formatting
I would at least serialize the list
the world is a troubled place and things change globally more often than folks think
7 digit phone numbers became the US standard in 1967
for instance
but you're concerned more about dial codes
so given that I used that list from the stack overflow, how would I serialize it? Like what do you mean by that?
Mayor McCheese
REPL Result: Success
Result: string
Compile: 613.139ms | Execution: 46.191ms | React with ❌ to remove this embed.
Mayor McCheese
REPL Result: Success
Result: string
Compile: 641.178ms | Execution: 44.158ms | React with ❌ to remove this embed.
so you just need a class that contains the dictionary, and can derserialize it
https://pastebin.com/PbVveRmn
ok so in my playground, I turned it into a function, however, I don't think I have the return type correct. I can't have the function be a void and I'm not sure what return type it is
so, I wasn't super clear probably
the point of serializing is that you can store it in a file ( as a for instance )
would it be more efficient to store it in a file as opposed to just serializing and deserializing as needed on call?
so you'd serialize the dictionary because countries to change, not frequently, but dial codes might change, countries might change, go away, change, etc.
so then you're just replacing "a file"
if you don't care about that and want to hard code it that's probably fine too
the last country to get created was in 2011
ok I'm with you
also, depending on your audience you might want more or less dial codes
apologies. discord took a crap. https://pastebin.com/bX8tnH8a I turned it into a class that I could use to not only get all of the prefixes for the drop down list, but to get dictionary from the json file and be able to match the selected prefix from the drop down to the matching country code for the formatter and create the file if it doesn't exists on run time. Thoughts?
I'll look at it deeper in a bit
you probably don't want to read in the file every time you call those methods
ok what would be a better option?
caching it
only load it if you haven't already loaded it before
ok. you can see my updated code at https://github.com/mekasu0124/MeksPhoneBook would you mind viewing it and telling me which file would be best for that and how I would do that?
you'd add a property to keep your deserialized object and in your method have something to the effect of
return MyObject ??= LoadMyObject();
also, I have
.vs
in my .gitignore
but it won't ignore that folder for some reasonif you already commited files from that folder git will continue tracking them until deleted
deleted from the repo or the workspace?
I don't think I'm supposed to delete them?
the repo
then delete them, commit, then put them back
it's just VS metadata either way, it won't break anything permanently
hence not needing to commit them in the first place
oh ok. I got you
The point of serializing the data is to not to have to redeploy code each time you make a change to data like that
if this is a website, do you want to redeploy because you remove some contries, or add some countries?
I do apologize, but I don't think I'm fully understanding with this part. Where would I do this at? Like which file and how? I don't believe I've done this before. My CountryCodes class handles creating the file and then I just load the file and pull the resources I need
the easiest way is to have your class take a dictionary in the constructor
and disconnect the two aspects
yeah, if you don't want to lazy load then just load it in/before the constructor
and i would personally deploy the "default" list as an embedded resource instead of manually creating it in code, then copy it to the filesystem if one doesn't exist
again, this is regulatory/technical data that isn't subject to change, you can viably hard code it as well; it'll change very infrequently
ok. do you mind if I show you the path of usage for the CountryCodes? Maybe that can help better explain it and help me figure this out more easily?
ok so the code block above is my
App.axaml.cs
. When the program starts, it checks if the json file holding the country codes exists, and if it doesn't then it calls to create the codes, and then that class instance is passed to main window view model.
and in my MainWindowViewModel
that CountryCodes cc
is then passed to the view model for NewUserViewModel
and in my NewUserViewModel
(which should have been named new contact) uses that _cc
to get the list of number prefixes from the file like +1, +41, etc
. So with me passing the class around like I am, and using that class instance to call the functions appropriate to the screen, that's how I get access to the information. I have updated my code on my repo https://github.com/mekasu0124/MeksPhoneBook. I just don't guess I'm understanding what you guys mean. What file would I cache my json file in, and how would I cache it? I do apologize for seeming stupid. I hope I don't upset you guys