How to Cache API response, with Hono JS & workers
Hello all newbie here, I am making an API where in an endpoint I am calling a third party API and sending a JSON response to the client.
I tried adding cache headers to the fetch API, but I am not able to see any
cf-cache-status
in the headers.
My approach
Home controller calls the api
i have set the cache-control headers, but cannot see the cf-cache-status
header can anyone tell me what am i doing wrong. also i am not using fetch exported by cloudflare.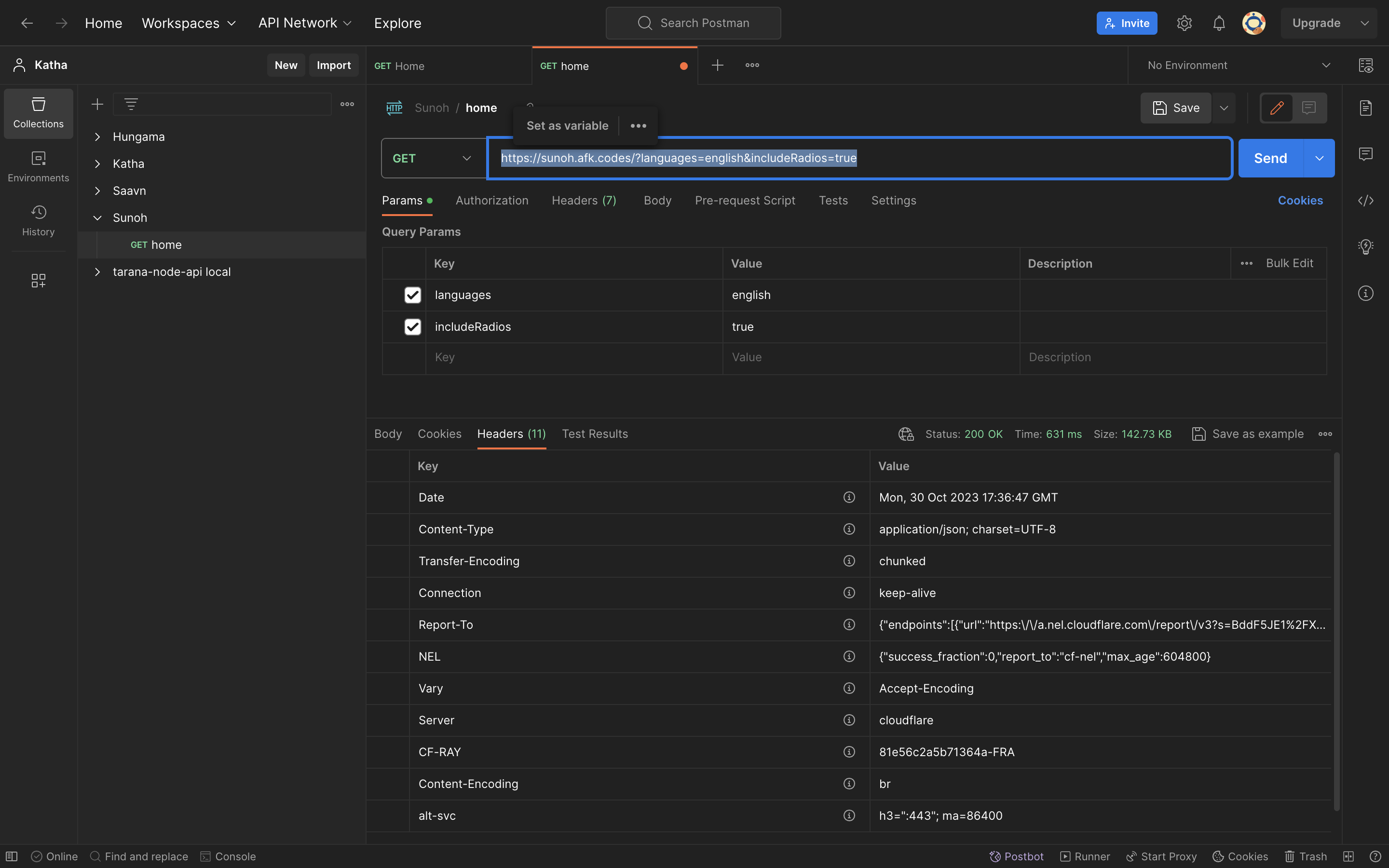
10 Replies
You're not returning the response headers from your fetch request, only the body.
If you're not modifying the body or checking any of it's properties, you can just return the response in
homeController
and return that in your Hono handler.so you are suggesting instead of data i should return the entire response ?
If you want to see the original response headers, yeah.
sure i will try that and and update here
This did not work
I'm confused. Are you trying to see the cache status of the sub request in your response?
@Cyb3r-Jok3 yes i am not seeing any thing related to cache header, also i dont think the responses are being cached so there is no way to verify
Also wanted to know if my implementation is correct or am i doing some mistake
hello @Chaika can you also have a look at my issue i have been waiting for someone to point what i am doing wrong please help me out
i read through some of your older answers as well tried to implement that i understood but it still doesn't seems to work
sorry for tagging but i am stuck and no one is replying after a single message
You are just returning the JSON data not a response with cache headers. Try using
return c.json(res, headers: {'cache-control': 'max-age=3600'});
understood, @Cyb3r-Jok3 this cache header i have added not and this shows up but
cf-cache-status
doesnt shows up in header so i have no idea if its already cached in edge or not.cf-cache is a specfic header from cloudflare that indicate cache status. Workers run before the cache so the header will no be present unless you add it
Just as an FYI, you can also use Hono's cache middleware - https://hono.dev/middleware/builtin/cache
It does mostly the same thing as others have already suggested