I wonder if I should be using the IEquatable interface instead of IComparable to compare on objects
I wanna ask you for your opinion to use the IEquatable interface instead of IComparable? I mean obviously I dpn't need to sort my list of ogjects or perform any sorting, therefore I might need to use IEquatable since it only checks if an object is equal to another object, what do you think?https://paste.mod.gg/fsksuwwdtlvl/3
BlazeBin - fsksuwwdtlvl
A tool for sharing your source code with the world!
70 Replies
right, IEquatable would be a more specific solution if you only need to test if two objects are equal
Awesome, it makes sense what you mentioned bro. Then I'll go with the IEquatable option π π
But how does the interface
IEquatable
does the comparing mate? I mean based on what order?it doesn't, you have to implement the code that decides if the objects are equal
interfaces are just a way to say "this class has this method," it doesn't say how that method is implemented
for example, if you have a class
then you must write a method in
MyClass
like
or else you will get a compiler error because the class does not implement the interfaceBut I do have a method already mate, should this method be specifically for the interface?
Aha! I see, so there should be a saparated method that belongs to the interface where we perform the comparison operations on two objects, right?
correct, interfaces require that you implement all of the methods and properties that they declare exactly as they declare them
it can't use any method, you have to follow the rules of the interface
Aha! Ok! But brother, if you look at my code I have separated class for each logic e.g. class Customer, class Card, class DataImporter, Fomr1 and lastly multiple sub-classes, so where the interface should be defined based on what you mentioned?
I'm not sure what you mean. Implement it on classes where you need a generic equality implementation
Sorry, I'm already done with this step bro. I found out that I need to define the interface class IEquatable in every class that is involved with equitable operation.
correct
i don't need to see the entire class, i get the idea
You were right
Ok
but it looks like you still need to actually implement them
Yeah only impelementing the equality operation in the base class DataImporter, right?
what? no, you have to write a method body for every class you've put the IEquatable interface on
this just causes a crash because you're throwing an exception
Because this is where we are adding objects to the list dataList and then we need to compare data received from the client program with the stored data in the list
Jimmacle
for example, if you have a class
then you must write a method in
MyClass
like
or else you will get a compiler error because the class does not implement the interfaceQuoted by
React with β to remove this embed.
Oh! Really, didn't know that mate.
that's the whole reason for interfaces
they force classes to implement certain properties and methods so they can be used in more generalized code
Aha! Ok! This is the purpose of using interfaces as Thinker told me too.
for example
this method will work with any class that implements IEquatable for itself
You're right mate π π
Ok! You're right. It looks clear.
So now I should figure out the small steps that I'm gonna be taking in order to implement the interface's logic.
I need to think for a moment π€
I mean currently I'm adding objects into the generic list and my goal is to use the interface to compare the received data from the client with the data in the list
that doesn't sound like a good reason to implement IEquatable
Really
like i said before, its main benefit is allowing you to define classes that can be tested for equality in generic/library code
i'd have to see a concrete example of what "the received data from the client" and "the data in the list" looks like
My friend the idea behind using this particularly interface is because I'm adding objects in the list and I thought it could be a good practice to get introduced to using such interface that's all.
Ok! Didn't you see the Fom1 class where the logic of receiving data from a client program is implemented?
BlazeBin - fsksuwwdtlvl
A tool for sharing your source code with the world!
I'm required to create a custom generic base class and a generic list and add the values of Customer class & Card class both in one generic list.
To be honest with you, I'm not really sure about the equality operations inside the methods in both classes Customer & Card, so I think you are right by avoiding the use of this type interface, because all I need is to perform operations in Dataimporter & Form1 classes not more than that.
it's ultimately up to you, there's no particular reason to do it here but you still can
one thing that looks weird is how you have generic methods in generic classes that accept a different type, like
DataImporter<Customer>.VerifyData<Customer>(receivedData);
i would think VerifyData
should use the same T
specified in DataImporter
because as it is now, i don't think the generic type specified for DataImporter is actually used anywhereBut if I implement the the interface's operation in the DataImporter class, what am I gonna do with the methods in Customer & Card? .
Aha! Ok! So you mean I shouldn't separate them when performing the invocation and they should have type
<T>
as a an argument type for the parameter type DataImporter<T>
i'm saying making a method in a generic class generic only to use the same type is redundant
and i don't understand your other question
What do you mean here by it should use the same T mate?
What does redundant means?
DataImporter is already generic, the method does not also have to be generic because you can use the T from the class's type arguments
it means you don't have to do both
Could you please answer this question, becuase I'm a bit confused?
i told you i don't understand what you're asking there
Yes that's right, it should be generic based on the requirements π
why are you implementing any interfaces on DataImporter?
Aha! Ok! That's a good point. I made the method generic because it's a list type method which's a generic?
but your class is generic and your method is generic, why?
pick one or the other
Let me be more clear so you get the idea π I'm implementing an interface the
EIquatable
in both classes Customer & Card
and the interface is demanding me to define this method, I need to implement an equality implementation for the received data, my question, what should I do with this method? Should just stay there that's it and don't put any code in the body? i told you what you need to do there 2 times
look at the method and think about what you might have to put inside
Aha! Ok! So that means there's no need to make the method as a generic, got it.
So if I don't put code or implement an equality comparison then I will get an exception thrown, right?
there is a very explicit
throw new NotImplementedException();
, so yes
all that method does is throw an exception
you need to change it to determine equality between your object and another instance of your objectI don't think it would throw an exception if an object is not equal to another object, it should throw when an operation is invalid.
nobody said it would
it's very clear what the method does now, and it's not what you want so you have to change it
Yeah explicitly it's saying if you don't feed the method with equality operation, that means you're not using the interface.
that's not what it says at all
Just assumption
you don't need to assume anything
look at the method, it's one line
Ok
when you call Equals what happens
It compares if an object is equal to another object
faraj, just look at the method
don't tell me what it should do, tell me what it does do
Ok
I was focusing on the exception and not on the method signature type π€¦ββοΈ
It's basically a boolean type
that doesn't answer my question
when you call that Equals method, what happens
It should check if the value is true or false
Because it's a boolean type
i'm really trying to give you a second chance here man
one more chance to answer my question
if you can't tell me what a one line method does i can't help you
Sorry bro, this is the first time I use this interface and this method, in c# we usually define a boolean type method that executes code inside the body and then we are able to return either true or false e.g. when validating.
Ok! Let me think then.
hopefully someone else will be able to find a way to help you learn, but that person is not me
good luck
hhhmmm
Ok
What is wrong with that if I don't know yet/haven't learn yet a new advanced concept like this interface, you should teach me what it is instead of putting me in a test?
this isn't new and advanced, it's literally a method that is one line long and does something very obvious
don't ping me again
If you don't, then I'll just go simply google it, learn it and get back to you with an answer, then we can continue instead of walking away.
Ok! I won't, thanks for helping me out though.
@The king of kings I'm afraid this is going to have to be your final warning in regard to how you use this Discord. I do not doubt the level of effort you put into learning and I do not doubt you want to learn programming, but you have been part of this Discord guild for well over a year now and show the same patterns when learning something.
Whilst I do not want to discourage your learning or your endeavour to becoming a programmer, this is not how you go about it and it is not sustainable to how you would or should learn if you want to make this a viable career for you.
There are numerous examples where there are fairly obvious error messages, solutions, answers or prompts given to you, but it seems to be a recurring pattern that they're either ignored (as a product of inexperience or on purpose, I'm not sure) or the same question comes up again in another form later down the line.
This server is a resource for many people - people who volunteer their time. It's difficult for me to see this recurring pattern and not see it as a sign of clumsiness or disrespect at worst.
Do you understand what I'm saying?
You wrote all this just to show me a message, man you too much time, but I donβt honestly. Excuse me, do you mind if I ask you why am I getting warned for? What have I done that is standing against the serverβs rules lol? Please leave me alone and donβt show up with your disappointed messages telling me βyou have been for a year in c#β
You have demonstrated in this message why I am warning you. I do not know if it is a language barrier or not, but repeated ignorance of subject matter in messages does not help you or the people trying to help. You need to read and understand what is being said to you.
Sorry for the delay
Could you show me what I have demonstrated that is against the rules? I promised some people last night I will not disturb anyone π€
I haven't written anything that is rude or hearts someone's feelings
There are numerous examples where there are fairly obvious error messages, solutions, answers or prompts given to you, but it seems to be a recurring pattern that they're either ignored (as a product of inexperience or on purpose, I'm not sure) or the same question comes up again in another form later down the line.I don't know if this is just clumsiness on your part. I will give you the benefit of the doubt. But you need to stop ignoring what people are actually saying one way or another.
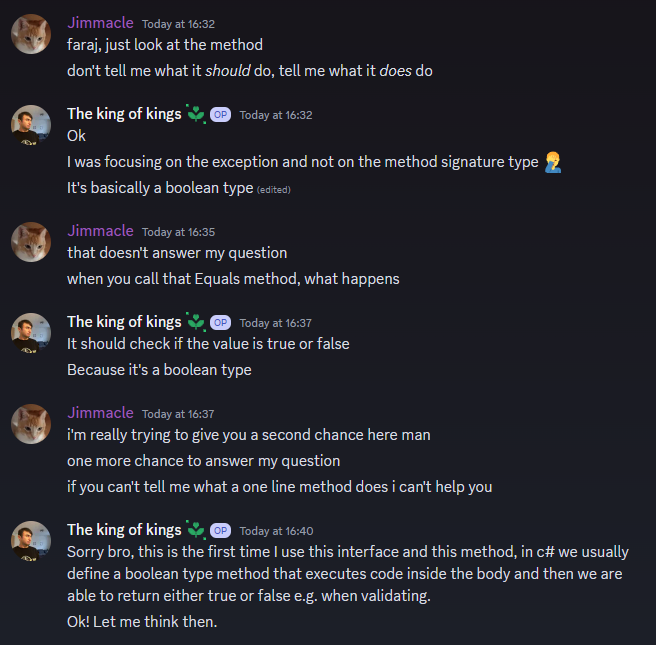
re-read this exchange, if you do not understand why this (amongst other messages of the same pattern) causes an issue, you need to tell me you do not understand.
I will explain it one more time for you.
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.