❔ Removing dupes in a linked list
I need to make a function that gets a linked list, and returns that linked list with all sequences of 3 or more consecutive equal numbers be removed
for example: 1,3,3,3,4,5,5,6,9,9,9,9
becomes
1,4,5,5,6
129 Replies
$details
When you ask a question, make sure you include as much detail as possible. Such as code, the issue you are facing, and what you expect the result to be. Upload code here https://paste.mod.gg/ (see $code for more information on how to paste your code)
well i dont have any code written as i dont have an idea as to were to start
sadly
thats also my issue
alright
let's break down the problem
I have a very vague idea but no more than that
gotta start somewhere
what is your vague idea?
so
first thing is

p1 being a pointer on first object p2 on the one after
and inside that if clause i would put it into a new list
hold on
ill write it so its more clear
so
A) your plan is to build a new list, rather than trying to modify the existing one
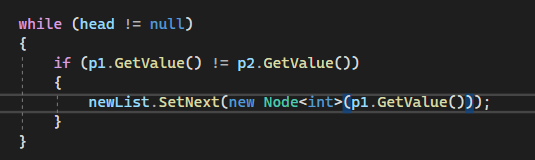
yeah thats what they want in the question, sorry i didnt specify
great
although it really doesnt matter since i can make a copy of the old list i have a function for that
you're allowed to use the built-in
LinkedList
here, or you have to write your own?