How to resolve "Cannot find 'AKMicrophoneTracker' in scope"?
Trying to create app that checks your pitch (from microphone) using AudioKit from github.com/AudioKit/AudioKit, but something ain't working in code. I tried to even use GPT4 to help, but it couldn't figure it out. Keep going circles.
Trying to create app that checks your pitch (from microphone) using AudioKit from github.com/AudioKit/AudioKit, but something ain't working in code. I tried to even use GPT4 to help, but it couldn't figure it out. Keep going circles.
Error upon building:
// This section imports necessary libraries.
import SwiftUI // Library for building user interfaces on Apple platforms.
import AudioKit // Library for audio processing.
// This is the main content view of your app.
struct ContentView: View {
// This section declares properties that store data for the view.
// 'pitch' holds the detected pitch frequency value.
@State private var pitch: Double = 0.0
// 'isRecording' is a flag that checks whether the microphone is currently recording.
@State private var isRecording = false
// 'mic' is an instance of the AKMicrophoneTracker class from AudioKit which tracks microphone input.
private var mic: AKMicrophoneTracker!
// This is the initializer for the ContentView.
// It's called when an instance of ContentView is created.
init() {
// Here, the microphone tracker is initialized.
mic = AKMicrophoneTracker()
}
// The 'body' property defines the user interface for this view.
var body: some View {
VStack {
// This is a text label that displays the detected pitch.
Text("Pitch: \(pitch, specifier: "%.2f") Hz")
.padding() // Adds padding around the text.
// This is a button the user can tap to start or stop recording.
Button(action: {
// This checks if it's currently recording.
if self.isRecording {
// If so, it stops the microphone tracker.
self.mic.stop()
} else {
// Otherwise, it starts the microphone tracker.
self.mic.start()
}
// The 'isRecording' flag is toggled to its opposite value.
self.isRecording.toggle()
}) {
// This displays the button's label, which changes based on whether it's recording.
Text(isRecording ? "Stop Recording" : "Start Recording")
}
}
// This section repeatedly fetches the frequency from the microphone tracker every 0.1 seconds.
// and updates the 'pitch' property with the detected frequency.
.onReceive(Timer.publish(every: 0.1, on: .main, in: .common).autoconnect()) { _ in
self.pitch = self.mic.frequency
}
}
}
// This section provides a preview of the ContentView for the Xcode canvas.
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
// This section imports necessary libraries.
import SwiftUI // Library for building user interfaces on Apple platforms.
import AudioKit // Library for audio processing.
// This is the main content view of your app.
struct ContentView: View {
// This section declares properties that store data for the view.
// 'pitch' holds the detected pitch frequency value.
@State private var pitch: Double = 0.0
// 'isRecording' is a flag that checks whether the microphone is currently recording.
@State private var isRecording = false
// 'mic' is an instance of the AKMicrophoneTracker class from AudioKit which tracks microphone input.
private var mic: AKMicrophoneTracker!
// This is the initializer for the ContentView.
// It's called when an instance of ContentView is created.
init() {
// Here, the microphone tracker is initialized.
mic = AKMicrophoneTracker()
}
// The 'body' property defines the user interface for this view.
var body: some View {
VStack {
// This is a text label that displays the detected pitch.
Text("Pitch: \(pitch, specifier: "%.2f") Hz")
.padding() // Adds padding around the text.
// This is a button the user can tap to start or stop recording.
Button(action: {
// This checks if it's currently recording.
if self.isRecording {
// If so, it stops the microphone tracker.
self.mic.stop()
} else {
// Otherwise, it starts the microphone tracker.
self.mic.start()
}
// The 'isRecording' flag is toggled to its opposite value.
self.isRecording.toggle()
}) {
// This displays the button's label, which changes based on whether it's recording.
Text(isRecording ? "Stop Recording" : "Start Recording")
}
}
// This section repeatedly fetches the frequency from the microphone tracker every 0.1 seconds.
// and updates the 'pitch' property with the detected frequency.
.onReceive(Timer.publish(every: 0.1, on: .main, in: .common).autoconnect()) { _ in
self.pitch = self.mic.frequency
}
}
}
// This section provides a preview of the ContentView for the Xcode canvas.
struct ContentView_Previews: PreviewProvider {
static var previews: some View {
ContentView()
}
}
Cannot find type 'AKMicrophoneTracker' in scope
I'm a total noob, so it must be some silly issue. Would be thankful if anyone could help.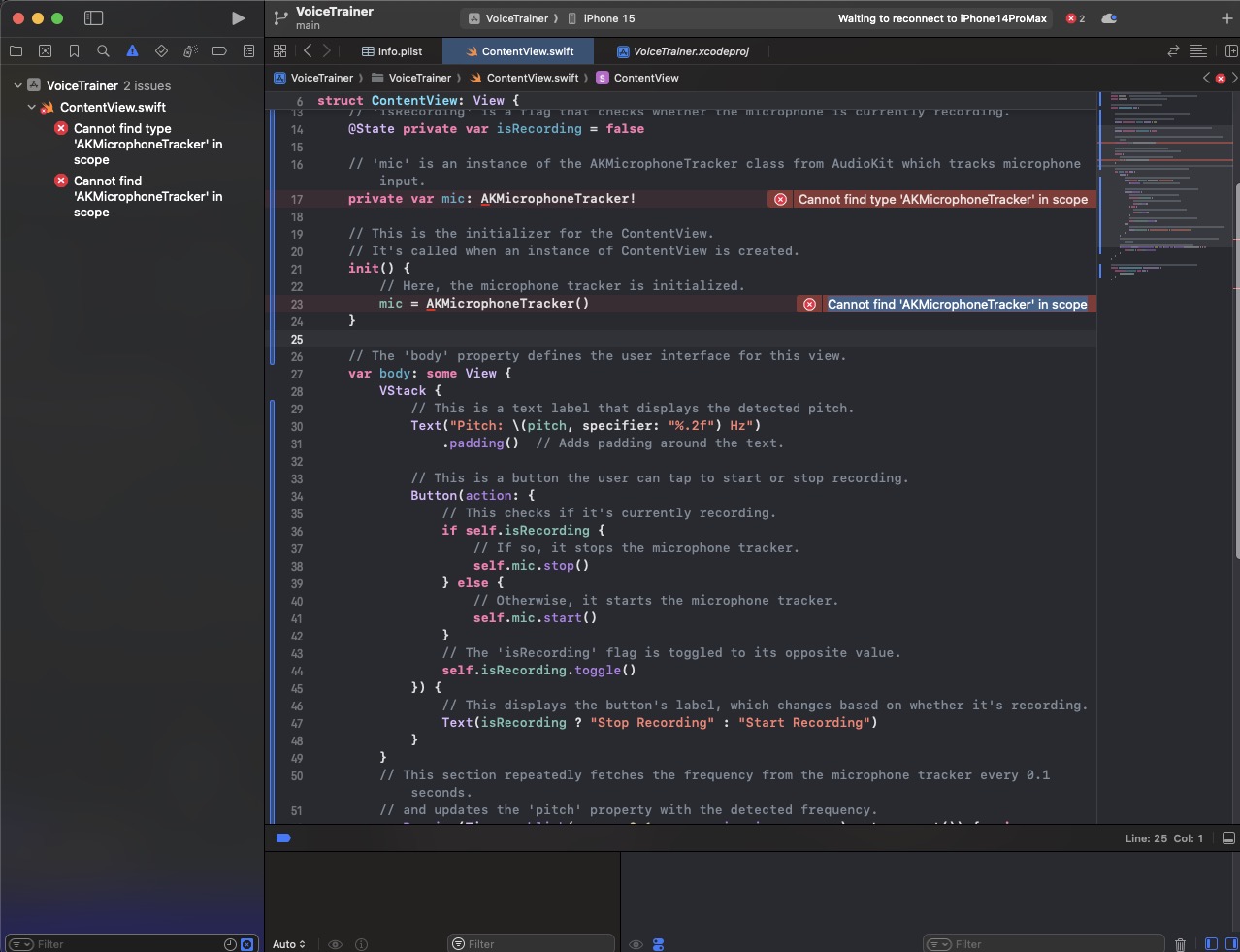
0 Replies