β How can I make a Console app with cool text that when displayed slowly writes whatever
I'm trying to make a little story console app and I want to make the wording so that when you want to continue you hit enter then it displays the letter one by one in quick succession as if to type fast.
33 Replies
You could do a simple
while
with Console.ReadKey
and move through a string
one character at a time:How would I do that?
How experienced are you with C# so far?
Only been using it less than a month here and there
I know all the basics but not much
So how would you start tackling this given what I've already stated and your current experience?
I'm not sure, I might try to set a timer and display a certain one of the letters correlating to the timer? But I'm probably not smart enough for that
First off, I jsut want to mention that users typically hate slow text π
but I will get you an example
one moment
ok, I'm not gonna make it realy slow, just not instant
Oh, so they're not holding down enter here
They're just pressing it once and it starts printing it out
?
Yes
I realize this may be look a little complicated but I can strip it down for you
that is a helper method that will print the output with small random delays
O_O way above what I was going to do lol
Impressive π
you could call it like
and it would slowly print
"Hello World! Hello World! Hello World! Hello World!"
I like ZP's version better because it's probably much closer to what you truly want visually, but perhaps this simplified snippet will help you better understand what their snippet is doing.
here is a simplified version of the same method:
just without the extra optional parameters
and withotu the extra parameters you don't need the extra exception handling
adding a little bit of randomness to it goes a long ways imo
Ok, Iβm had to step away from my computer but I will try it out when I get back thanks
So will I have to do this individually for each new line I write?
No; you call it for each line
I may have misunderstood your question 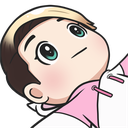
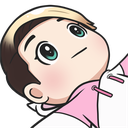
No worries I explained it bad, just a simple text adventure game. A bunch of if statement for multiple options of paths in the game, simple do this or do that, then based on the option I want it to write out the story quickly, a speed just fast enough so you canβt catch up while reading, instead instantly displaying it the second you hit enter to continue the story
so you need help with menuing?
if you can share your current code that would help
Currently I cannot but I can in a couple hour, we can continue then if youβd prefer
there is another example if that helps at all. based on your last comment you sounded like you were struggling switching between views
there are multiple ways to do it
in the code above it will start at the main menu. the user has to press either
1
, 2
, or 3
and when they do it will transition to another "view" based on what they pickMines way more basic, it like βconsole.writelineβstoryβ
String optionOne = console.read key
If optionOne == (one of the option)
{
(More Story then another if option and on and on)
}
Else if (other option)
{
More story on and on
}
that's okay, but you will likely need some loops. you don't want your game to break the second the user provides invalid input
and spliting your code up into methods can help keep it clean
How do I split it up to methods and make loops? Sorry if Iβm asking for too much, you donβt need to help with the whole game
this (reply) example alreay splits things up into methods and includes loops
for exmaple, if you are on the main menu and you press "4", the game will not crash
it won't do anything
it will loop until the user provides valid input
Ah
the user must press either 1, 2, or 3
the loop is the
while (true)
is an "infinite loop" so it will go on forever. In general you don't want to code infinite loops if possible, but for console games like this, it is fine
return;
exits the current methodAlright
so even though there is a
while (true)
, when the user presses 3 while in the main menu, the return
will break out of the current method and end your code
Side Note: If you are interested in making console games there are lots of examples on GitHub. If you would like a link to some just ask.Sure, would Definitly like some reference
https://github.com/dotnet/dotnet-console-games here are some examples. and you can play most of them in your browser so you don't even have to download and compile them. but they are all open source so you can see how they are coded
Awesome thanks a lot!
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.