Overloading "+" operator
I am adding fractions together and need to overload the plus operator, but I keep running into an error while I am building the code. Before even adding them together, he wants us to find the GCD (greatest common denom) to simplfy the fractions down before adding them together, which is where my error arises.
I get an error under "denominator" after the "Math.Abs(numerator)" and when dividing the denominator by the GCD
18 Replies
make a loop and go through numbers from 1 up until the denominator
in the loop, add all the divisible ones into a list
and then compare via the list
@Dinny
wanna know what's cool
i asked chat gpt
It seems like you're trying to simplify a fraction by finding the greatest common divisor (GCD) of the numerator and denominator. The issue you're encountering could be related to the data types you are using. Ensure that
numerator
and denominator
are of appropriate types, and the GCD function returns the expected result. Here's a potential solution:
In this code, I explicitly cast denominator
to uint
when calling GetGCD
to ensure that both numerator
and denominator
have the same data type for finding the GCD. Make sure you have a GCD calculation method, as shown in the code. If you don't have one, you can use the System.Numerics.BigInteger.GreatestCommonDivisor
method to find the GCD.
If you continue to encounter errors, please provide more information about the error message you're seeing or the data types of numerator
and denominator
so that I can provide more specific assistance.oh wow, thank you so much
hope chatgpt helps
š
and i have heard of usinf chat gpt nut i dont want things necessarily done for me
i wanna understand why and how
ok lemme look at what u put
it explained
yeah i see ur explanation i just hadn't read it yet
i kinda thanke du first lol
Hmmmmmmmmmmmmm
okay real quick, the area under the "finding gcd for num and denom," my prof didn't put the (uint)denominator with his code and everything works properly.
Ill send a picture
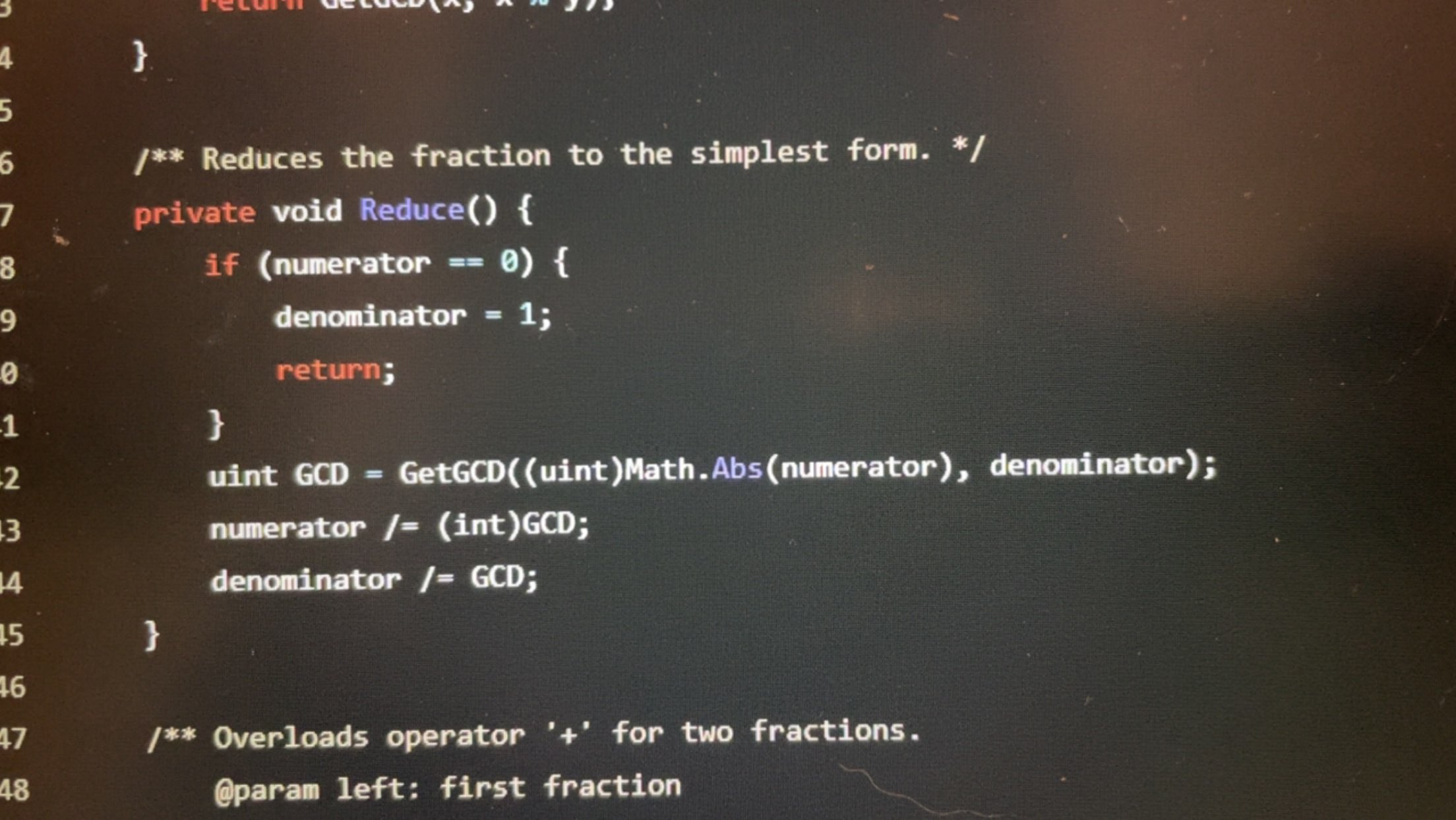
so did it work out?
whenever i try his code, like exactly, i get an error, but when he does it, it works fine
lemme see what the squiggal says hold on
it says i can't convert uint to int
Try unchecked((int)yourNumber) but be careful
it will convert yourNumber if it overflows Int32
to negative
wait hang on i have an idea
for this, i have a method for this in my code. it is this
he hasn't taught us recursion so, so we had to write this specifically from him
What was the problem again?
Type conversion?
yes
it keep ssaying i can't convert uint to int implicitly
Did you try what I said earlier
This one
unchecked((int)yourNumber) will be negative if yourNumber is greater than Int32.MaxValue
oh yeah i caqn try that now
but where would i put that ?
i've never heard of that until now so i am unsure
Where it says you can't convert
uint is much larger than int, and uint promises that it will be a positive integer
In beginner's terms
int can go both ways
It can be positive or negative