So I've made a bot, but the command doesn't work, at least they don't get recognized. Any Ideas why?
15 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!
- โ
Marked as resolved by OP
Unknown Userโข14mo ago
Message Not Public
Sign In & Join Server To View
Am I thinking right or not?
Unknown Userโข14mo ago
Message Not Public
Sign In & Join Server To View
oh.
Let me check if it works now
Still nothing... Nothing even in the console
Unknown Userโข14mo ago
Message Not Public
Sign In & Join Server To View
That's what I did before even I started coding
but yeah
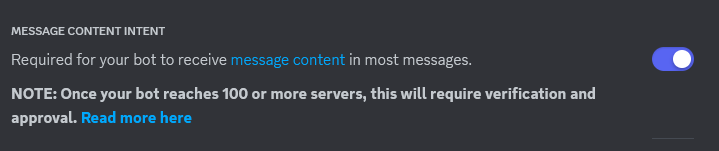
Unknown Userโข14mo ago
Message Not Public
Sign In & Join Server To View
It logs in and it doesn't seem to grab the messages 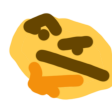
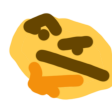
Unknown Userโข14mo ago
Message Not Public
Sign In & Join Server To View
Unknown Userโข14mo ago
Message Not Public
Sign In & Join Server To View
I just added to the intents
GatewayIntentBits.MessageContent
. I'll check if it works right now
It works now! Thanks for the help @Jรด ๐๐๐ฆ๐ป
<a:AAE_Yippee:768160251077197904>