❔ Need help adding a label from a child class to the screen that exists in the parent class
So im coding a board game and the board is its own class, now when the game ends I would like to show that by adding a label with some text to the screen, yet this label exists in the parent class because it takes up more of the screen than just the gameboard (which isnt the entire screen). Is there any way I can show this label only when the game ends (which is checked in the board class)? (please ask me more questions if needed this explaination is far from good :) )
151 Replies
is this using winforms? WPF?
windows forms yes
all im using
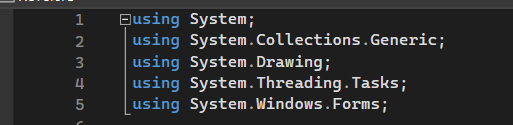
I didn't mean your
using
statements, I meant what GUI framework are you using 😛
but thats winforms, clearlyyeah, This is for a uni project and we get this kinda "preset" So i dont have to deal with most of the stuff like that :)
and the answer to your question is... yes! Yes you can show it based on a value from a different class. You just need to get a reference from the right thing to the other right thing somehow.
so as i have it now a method gets called if the game is finished
i would like to be able to make the method show a label thats inside of the other class
Do you understand the basics of classes and variables?
yes, classes im a bit worse at but methods and variables i get
i have some1 who could explain it to me if i dont understand tho, its just that he doesnt know how to do this either haha
so each "screen" or window you have is an instance of a class
The parent class has a list that goes through a foreach to set all UI elements to visible, If I could add this label to this list from the method in my child class I would know how to make everything work
you need to pass a reference to one of these instances to the other
probably the easiest way is if the one with the "game ends" check has a reference to the one with the label, but the other way works too
so the board is its own class and everything around it is a parent class
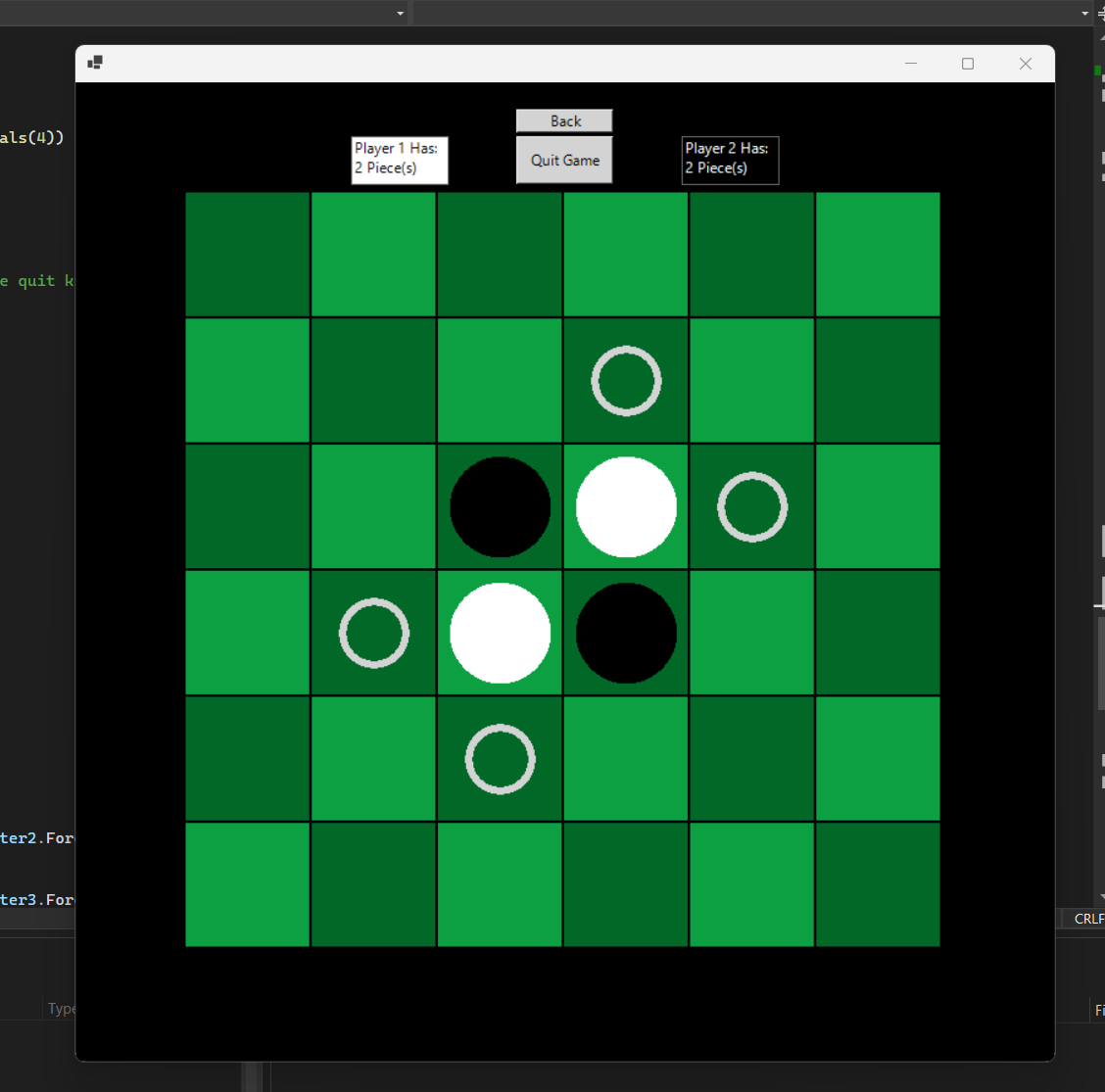
right, the board is a
UserControl
or similar?ye i have this check but its in the child class
dont worry about that
its a label
the board? the entire board is a label?
yes
wtf?
made with paint event args etc
ye its how we get thaught dw
its a seperate file
ok its weird as hell, but we can work with that
ye XD
so that class has the check for if the game is over
yes
and it calls the method
GameFinished
and the "parent", being the form itself, thats the one with the label you want to update when the game ends?
yes!
okay
so, there are two ways we can do this
we can do it quick and dirty
or using an event
which do you prefer
ye the event is what i had in mind
but i didnt know how to make a custom event
easy
what .NET version is this btw?
just to be sure
i had a similar idea by just using like simulate keypress with a key like f20 and then make that subscribe to an event in the parent class
lemme check
thats not quick and dirty, thats "crime against humanity"
how do i check..
LMAO true
open your .csproj
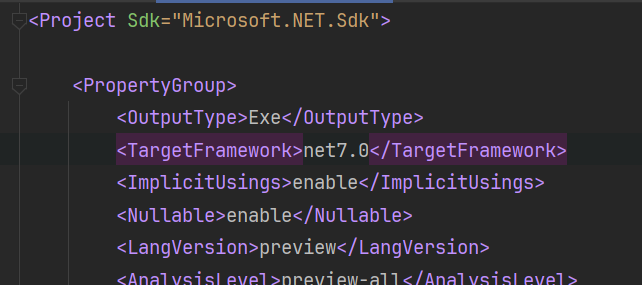
this is a .net 7 project
ah ok i see
7.0
wait

waiting.
7.0 windows
yeah thats fine
idk if its dsifferent
its just .net 7 with a "windows-only" restriction
because its winforms
oh fair
its fine
so, when the game is finished, do you want to send any info up the chain too
like, who won?
wait ye i prob should do that
i have some stats i want to send
okay, we can do that at the same time
cool
so first we make a class that represents that info
thats step 1
a class that should have a property for each value we want to send as part of the event
wait like an entire new class?
yes.
but that does not mean a new file too right?
different form a new .class
it doesnt have to be, no
jsut a new class
ok
something like this
ye i see
I dont know what you want to send, so I just made a string and an int
you put whatever you want there.
tell me when you have something. it doesnt have to be done now, we can add new stuff to it later
ok i see but i can use variables from my board class in this new class?
not directly
cuz that board class for example holds the amount of pieces per player and who won
but we will pass in information from there when we make an instance of this class
so you can create properties for that info here
we will "copy" the data from the gameboard into this class
then send (an instance of) this class to anyone listening on the event
ok and the
get; init;
is so i can use values there?kinda
ok ive never used this part of classes so thats cool
init
means its only settable when we are creating the class, after thats its immutableok i just have one variable for now thats fine
thats fine
can you show me the class?
isnt that the opposite of what we want?
no
thats exactly what we want
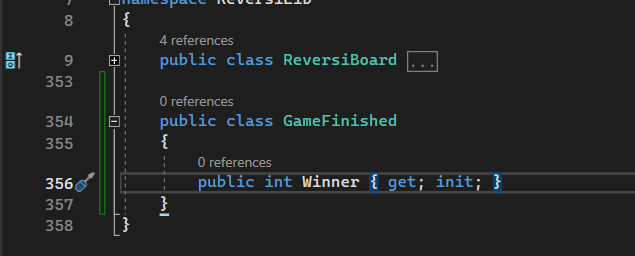
only the gameboard can say who won
everyone else can read it
hmm i see
okay, lets follow C# naming conventions
so we call it
GameFinishedEventArgs
ight
ok great
now, lets go to the board class.
ReversiBoard
I guess?yep
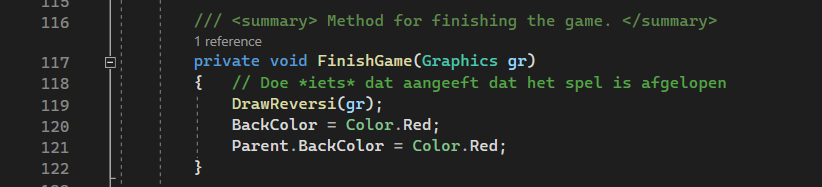
we need to make an event in that class
thats the method in the board class
we will use that soon
ah ok
first we need an event holder
should do the trick
it doesnt like the ? but its ok with it
did you disable nullable annotations, you bad person?
thats very naughty of you
but okay
not to my knownledge
its "optional" so dont worry too much
ah ok
i remove it?
well, can you tell me the error its giving you with it there?
ye sure

yeah okay
thats what I expected
that tells me you did indeed disable nullable annotations 🙂
thats okay, so remove the
?
could it be with the preset from uni? cuz I deff didnt on purpose
i did
so, what that line of code does is declare an event that other things can now subscribe to
cool
but lets wait with that, and fire the event!
so when the game ends, you run the
GameFinished
method, right?
or FinishGame
yep
cool
so go in that method
i am
and fire the event!
just like the += thing?
nah
thats a subscription
Handling and Raising Events - .NET
Learn to handle and raise .NET events, which are based on the delegate model. This model lets subscribers register with or receive notifications from providers.
I just realized I didnt link this before
ah ok ty?
so i just call it just like a method?
yeah kinda
OnGameFinished?.Invoke(this, ...);
where ... is a new instance of your event args
so we first gotta make one of those
and set the winner when we do so
yeah you missed one of the arguments
read my message again
oh bruh i read the dots as a pause
ok so how2
you know how to make an instance of a class, no?
well since this conversation my definition of class changed alot so
maybe?
a class is just anything declared with the
class
keywordi need to declare it first?
with = new()
right
thats not a declaration
thats creating an instance
which is what we want to do, but I just want to be clear, that is not a declaration
oh so its like a variable?
???
what are you smoking? can I have some?
as in a variable of time class?
type*
?
nvm
no, a class is a class
its not a variable
public class Steve {}
is a class called Steveye
note how classes can exist at top level, inside a namespace
methods, variables etc can't
mhm
only type declarations can be at the top level
ah i see
a variable is either local (inside a method) or a member (inside a class)
so now, we want to make a local variable inside our
FinishGame
methodight
of what type?
our event args class
ohhh wait i do think i had this
all the way back
in highschool
u mean like Class class = new (Class)?
kiiinda
[Type] [variableName] = new [Type]();
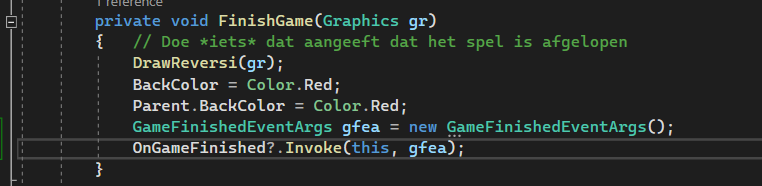
so like this
yeee
ok ye thats what i meant with new ()
its almost perfect
we just need to tell it who won
and i put that info in
gfea
?so change it to be
... new GameFinishedEventArgs() { Winner = 2;}
wait doesnt the ; have to be behind }?
especially if im giving it multiple variables
also i did
ok
well, now the event is done and being triggered
all we need to do now is make your window actually subscribe to it
yeah
but on what condition does it get called cuz it should be when
FinishGame
is being ran
or after*it runs when you invoke it
I thought that was obvious
so the parent class should have an method with object o and GameFinishedEventArgs gfea right?
kinda
you could do that, and wire it up
actually, VS will help us do that
so leave it alone for now
i thought it only set the variables in the class for every1 to read
thats what
new GameFinishedEventArgs()...
doesoh ye right
we then pass that to the event with invoke
invoking all the event handlers
go to the parent class, specially the constructor
the constructor is in the board class..
you dont know what a constructor is, do you? 🙂
uhm..
maybe
ok im in the parent class
this is getting hard without seeing code. can you screenshare perhaps?
i could
#dev-vc-1
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.