ā Learning Regex
I've never learned to create regex strings for validation, and I'm wanting to learn how to use/create them. I've used them before, but it was just a mere "<something_here> regex validation" on google, copy/paste and go.
1. What is a regex string
2. How do I write them to get the format that I'm wanting?
3. For this specific purpose, the username should be able to be something like
someUsername
, some$Username
, or SomEU123sername!$
Thanks76 Replies
for reference, this is how I currently validate usernames, and I just want to become more efficient with it
1: regex is a pattern matching "language"/system that can be used in most if not all programming languages
2: You'll need to learn regex to do that š
I highly recommend https://regex101.com/
regex101
regex101: build, test, and debug regex
Regular expression tester with syntax highlighting, explanation, cheat sheet for PHP/PCRE, Python, GO, JavaScript, Java, C#/.NET, Rust.
it lets you see what your regex matches as you write it
ty š
you'll need to be more specific about what you want your regex to validate thou. Just the characters being used? or "at least one special char, one number, one capital and one small letter" etc?
granted, imho thats not a good usecase for a regex
It's nice they support .net regex now, that wasn't the case until recently I think.
yup, they added it recently together with rust and java 8
so playing with the site for a few seconds, I put in this username and got this
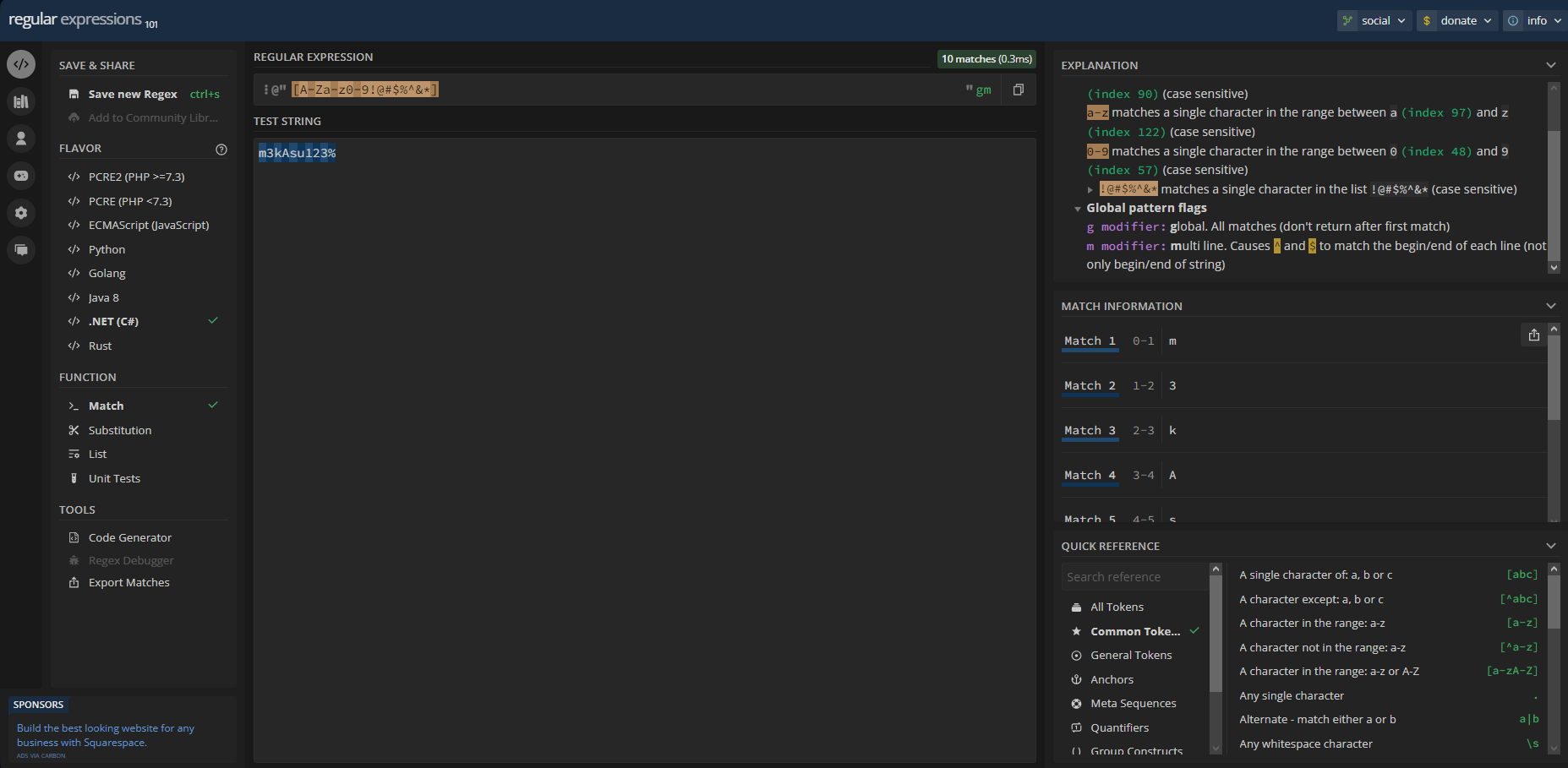
I do want it to be at least 1 char, 1 int, and 1 special
yeah you can see on the right in the "match information" that its matching each character as its own group
thats probably not what you want
I'd probably just use normal C# for that tbh
however, with this, I'm still not fully understanding so I want to try and see if I do understand
1. [A-Z] => matches case sensitive letters that are uppercase only
2. [a-z] => matches case sensitive letters that are lowercase only
3. [0-9] => matches numbers zero through nine
4. [!@#$%^&*] => matches these special characters case sensitive
right?
regex is good at finding and extracting patterns from strings
regular expressions are great, they're good to know, but good to know when to use them is good too.
so apart from emails, when are they good to use?
the first 3, yes. some of those special chars might have special meanings in regex, depending on flavor and position
finding and extracting patterns from strings
would you mind to give an example of that?
^
so it would be better for me to use how I currently do it already, but given I were to do that, how would I modify that function to account for at least 1 char, 1 int, and 1 special?
use int variables for counts and return if each count is at least 1?
I'd probably just loop over each char in the string and set some bools to true when I find the right conditions
Regex is actually pretty bad for checking emails tbh, it can be a part of validating an email; but you can't rely on regex for many forms of validation alone.
oh ok so what I was imagining. An if/elif/else
as for a valid usecase for regex...
https://github.com/logpai/loghub/blob/master/Linux/Linux_2k.log
look at this file
just a sample log file
if we wanted to quickly extract the user and the id from each row, regex would be a decent option
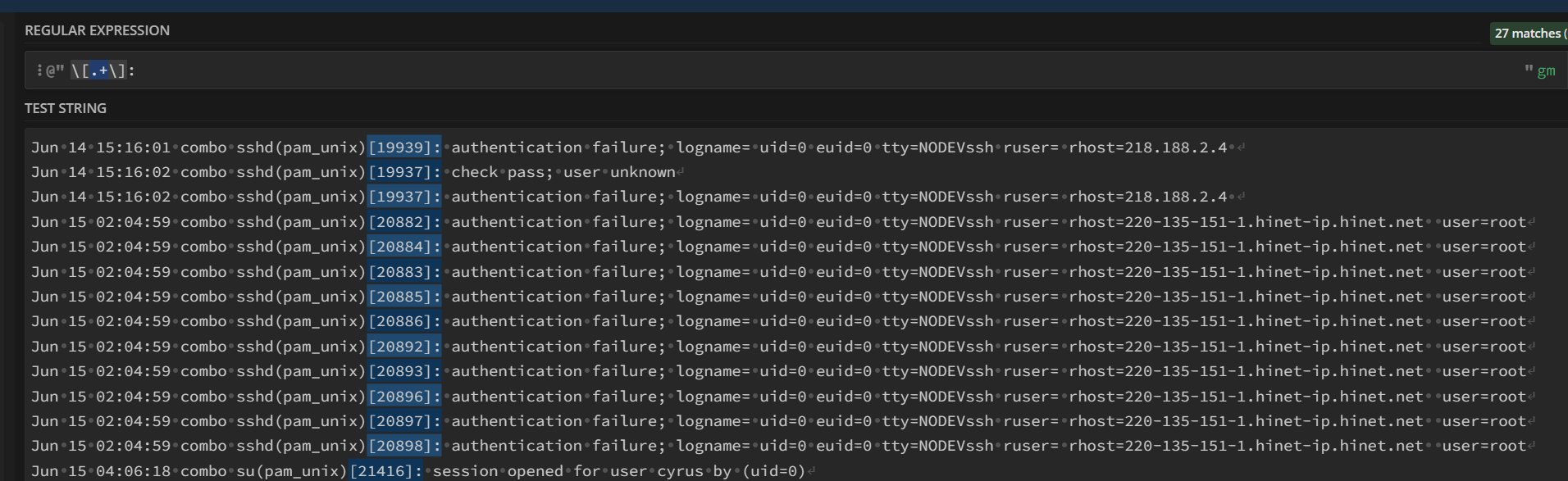
for example
Microsoft also has a documentation page on regex if that's more your style.
this is definitely a lot
I feel like I'm way off with this
no that seems fine, except maybe that return statement at the end
so it just needs to return a bool
and for the love of god
remove the recursion
>_>
a validation method should only validate
its not responsible for also taking input and retrying until its valid
if you want that, make that its own method that uses the validation
you've seen how I do that with
ColorCon
etcI fixed it
nah that entire block needs to go
it's just there for example usage. I have the ColorCon in the program
Whenever ( hasLet && hasNum && hasSpec) is true you can stop btw
what about this
I removed it
yep a lot better, slap some
continue;
in each of the matching blocks
if it was a number, we dont need to check if it was also a special character (as McCheese said)
alternatively use else ifsuhm
if (hasLet && hasNum && hasSpec) => return;
????
:d
what the fuck is going on there lolI'm a tad confused here. Why wouldn't I check if a special character if the requirements are at least 1 number, 1 letter, and 1 special character?
it was an attempt at this https://discord.com/channels/143867839282020352/1162384486395281419/1162389881675333672
Mayor McCheese
Whenever ( hasLet && hasNum && hasSpec) is true you can stop btw
Quoted by
<@1032644230432182302> from #Learning Regex (click here)
React with ā to remove this embed.
well, we check each character
the return should be after the loop
a single character cant be both a number and a letter
so if it was a number, we say "yeah we found at least one number" and we carry on
doing this with the loop, right?
fixed that
yes exactly
right which is why there are 3 ifs, correct?
the loop is
for each char in the string
rightwhich is the reason for the continues
yes
continue here means we move to the next c in s
right
or
letter
in input
using your namessweet. well thank you again for your help. sorry about the recursion ^_^
You could also use
input.Any(char.IsDigit)
.I actually have one more question. I can't seem to find which built-in method checks if a
(letter)
is either capitol or lower-caseyou could, but that would loop over the string 3 times (assuming you want to check for letter, number and special char)
no lie, I typed in google
IsDigit c#
and couldn't find anything lol
š
Oof, usually yields more results if you search for your problem instead of a possible solution.
ok bet because I want to shorten
string alphabet = "ABCDEFGHIJKLMNOPQRSTUVWXYZabcdefghijklmnopqrstuvwxyz";
so that the validation can check whether the letter matches regardless to it's upper/lower case standpointchar.IsLetter
Username 'Spiderman123#'is ValidGenerics are nice
Mekasu0124
REPL Result: Success
Console Output
Compile: 650.267ms | Execution: 60.476ms | React with ā to remove this embed.
why doesn't it recognize that an exclamation point is a symbol?
char.IsPunctuation is what you should use
it doesnt recognize many like @ # $ or %
arion
REPL Result: Success
Result: bool
Compile: 429.229ms | Execution: 31.244ms | React with ā to remove this embed.
oh ok. I got you. thanks š
For symbols they mean Unicode symbols like
Ā©
(Copyright sign)oh ok that makes sense
Though there is an issue of
$ not being punctuation and being a symbol, so you could do
char.IsPunctuation(c) || char.IsSymbol(c)
easy enough. makes sense. ty š
could I combine the two for efficiency?
hmm, @Mekasu0124 are u a student by any chance? if so you can get Jetbrains Rider or Resharper for free via student plans
A typical thing resharper would recommend is called "Replace with method group"
becomes
alphabet is unused, numbers too, also what do you mean by "combine the two"? the two if checks? not really since they check for distinct things, you could do something like
hmm, <ping> are u a student by any chance?I am self-taught. I am a student going for my Bachelor's in Computer Science with a focus in Software Development and Cyber Security.
If so you can get Jetbrains Rider or Resharper for free via student plansI don't like jetbrains anything and I don't know what resharper is
A typical thing resharper would recommend is called "Replace with method group"I didn't write the function which is why I replied to pobiega. I know he's probably asleep but he's been helping me make what I've already done more efficient which is why I asked if it would be efficient to call a method within the validator.validator: x => Helpers.IsValidUsername(x),
becomesvalidator: Helpers.IsValidUsername,
alphabet is unused, numbers too,yea I know. I removed them, just didn't remove them here.
also what do you mean by "combin the two"? the two if checks? not really since they check for distinct things, you could something like
if (hasCapLet && hasNum) => return true;
the validator is a true/false check so if I call a function that returns true/false then I should be able to call it. I ran it in a repl.it (see screenshot) and it worked as expected with returning a single true or a single false.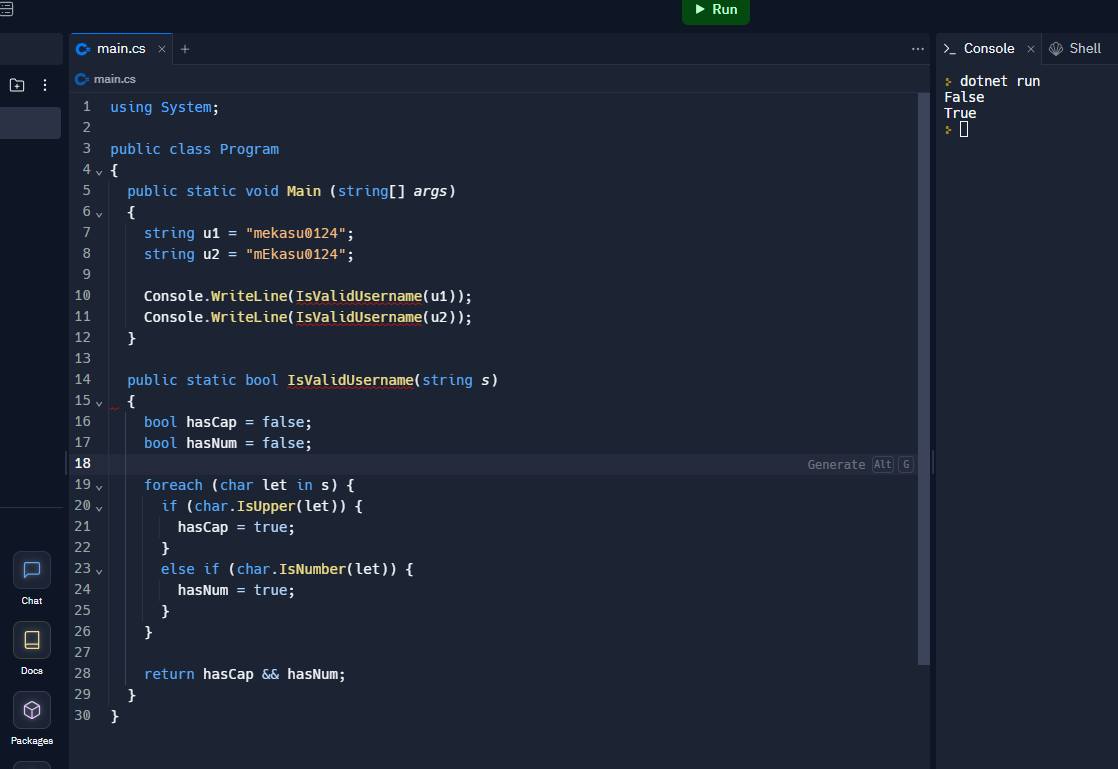
like this?
i've modified some small things
like the GetStringFromConsole is now a Predicate<string> (exactly the same thing as what the Func<string, bool> did, just more clear)
and the early exit checks for IsValidUsername via
Also, regarding Jetbrains products, Resharper is available on Visual Studio, it basically provides code suggestions for certain things
I set the username equal to the
GetStringFromConsole()
function call. So how is changing it to a predicate and removing the x =>
going to pass the user's input to the validator?
I know I didn't initially show that. That's why I'm askingChanging the type from a
code
A simple console app like this doesnt really need aggressive memory optimizations, but Its pretty much just a benefit to know such things are more optimized than other things
An established delegate like
Func<string, bool>
to a Predicate<string>
has 0 actual change when it comes down to it, but rather it makes use of already established delegates.
Removing the x =>
is a visual change too (kinda)
I say kinda, since it technically doesnt allocate a new delegate for the validator (it uses less RAM)
But do note, RAM optimizations doesnt rly need to happen until your code needs it. I started C# from Unity game modding so i've seen my fair share of 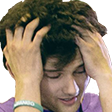
Action
is actually just
Action<T>
is just
A Func<T>
is just
C# has a few built-in delegates like this
Most common ones are Action
,Func<T>
,Predicate<T>
and EventHandler<T>
A Predicate<T>
is just
that was a whole bunch of french
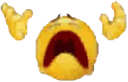
š
The only documentation I know how to read is Python
all the rest of it comes from YouTube "How To's" and StackOverflow
I uhh, might be a bit of a documentation freak
C# documentation got a bit boring so I switched to learning more about winapis (touching windows from almost any programming language)
C# and WinAPI documentation look nearly identical in terms of layout
eg. Predicate<T> vs LocalFree
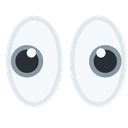
I'm glad you can read it š it hurts my brain
thanks for your help. I'm off to bed