❔ Converting null iteral or possible null value to non-nullable type
I'm getting this hint:
Do I need to do something about it?
59 Replies
need is a strong word, but you probably should
it means you are not respecting null annotations
ie
string name = MethodThatCanReturnNull();
string name
is not string? name
we're saying name definately has a value
but since MethodThatCanReturnNull
returns a string?
you get a warningSo what should I do? I'm new to C#
can you show the code in question?
Give me a second
but in general, you'd check for null and handle it
or use a nullable type on the left side too
Do you understand the concept of null and why we must handle it?
https://paste.pythondiscord.com/6YDA
This is the code
right
Because it can cause errors otherwise I guess
exactly
Im assuming
srIni.ReadLine()
returns string?
and not string
It's a line from an .ini file
I get that.
in general, that code is very error prone.
IndexOf
might return -1 (if the needle wasnt found)It is, but doesn't matter in my case
What should I do about the null hint tho?
I mean, if you are going to say "yolo i dont care about potential errors", then nothing
its a warning that you have a potential error
just like I informed you that indexof can return -1
thats a potential error
It wasn't handled before. I'm just rewriting a part of the code
So I was wondering if I have to do something about it
Well, what do you want to do?
Ignore it, or deal with it?
Fix it, but idk how
assuming your ini file isnt broken, you can safely ignore it
but if there is a chance someone fucks up the ini file, it'd be better to be safe and handle it
How would you handle it?
With a try and catch around it? Because that's already there
nah
First step is deciding how to handle it, as in, "can I recover from this?"
lets say that when you try to read the
strServer
it returns null
can you recover from that? would your program still work without a value for strServer, or can we default it?
if recovery is impossible, its best to throw an exception or otherwise terminate the methodYou mean if the main purpose of the program could still be fulfilled or if everything would work?
yeah kinda
maybe not "main purpose" but "this piece of codes purpose"
if
strServer
is null, we can't try to split it, that will crashWithout the strServer it wouldn't
right
then I suggest you add some null or throw. example
this will read the value, and if the value was null, it throws an exception
Is ?? saying if null?
yep
if the value on the left is null, use the right instead
Should I do the same for the other variables?
yes
Is it okay to throw so many new Exceptions?
its not happening unless the value was null
and only one will ever throw
as throwing will stop the rest from executing
Got it
And then it's fixed already?
the only downside here is that it looks a bit nasty
but you could fix this with putting it in a method
or an extension method
plenty of ways to solve it 🙂
So do ?? ThrowExceptionMethod()
no I was thinking more...
How does that work
you'd make an extension method that checks the value for null or throws
eh, not sure its a great idea now that I think about it
its less obvious what it does
So the way I planned would be better?
?
I mean this way
strServer = srIni.ReadLine() ?? throw new Exception("Unable to read server from ini file");
imhoAlright then I'mma do that
if you just slap a method for the throw you gain nothing
But what's the difference between this and the try and catch that's already around it?
a try catch catches exceptions.
what we are doing is converting a possible
NullReferenceException
into another exception with a much better message
so that its easier to find the problem
if we dont throw on null here, the program WILL throw an NREAah so this will be caught by the try and catch then?
yes
That is awesome
but NREs have terrible error messages
so we throw a better exception
Great, that also got rid of the hint
yep
since you are handling the potential error 🙂
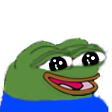
You'll need to handle those differently then
How would I do that?
I'm sure you can figure it out
There is already a try and catch with a throw around the part where the mail is sent
That should be fine right?
Impossible to answer.
It stops an exception from bubbling up, but what happens inside your code and it's state is... well, only you can answer that
Coding really isnt as easy as just wrapping a trycatch around everything 🙂
It looks good to me, so I'll leave it
Do you know by any chance what
does when client is an instance of SftpClient?
It sets the buffer size to be 4 kb
Which means what?
The comment says "bypass payload error large files"
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.