Changing text depending on url data
Hi is it possible to change text in specific <p> elements depending on the url data?
Example:
?period=quarter&periodnr=2&year=2023
I want the ? to change to the number depending on the bold part in the url ( periodnr=2 )
Don't really know where to start searching, copy pasting my question doesnt help alot
8 Replies
You'll need to access the URL Search Parameters https://developer.mozilla.org/en-US/docs/Web/API/URLSearchParams
then you'll need to use querySelector to target the p https://developer.mozilla.org/en-US/docs/Web/API/Document/querySelector, so you'll have to give it an ID or a class
and finally set the contents of the p tag using something like element.textContent https://developer.mozilla.org/en-US/docs/Web/API/Node/textContent
Alternatively, you can add a span into the p just for the number
and then select that instead of the entire p tag
Also don't forget to handle if that URL parameter is missing
Thanks for thelp guys
Maybe I can be a little greedy. Is there a way to insert characters in text coming from a datatable?
I want change it to 46,990, though I cant do it hardcoded cause the entire number is pulled from a database. Changing it directly in the table would be inconvenient
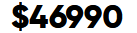
you can, it's mostly the same except for the URLSearchParams part
you have to pull it from the database, then do the rest the same
Gotcha
how you pull it from the database depends on your database setup, so I can't really say more about that without more info
Alright, Ill try doing it like you mentioned first. Appreciate the help