Weather App
I'm building a simple weather app project. Current, I'm using open-meteo.com's API for weather data. One of the parameters I'm using is weather interpretation codes. The variable documentation is attached (see picture)
My question is, what's the best method at converting code into description? I know there's multiple ways, such as if/else if, case, sets, etc. (I'm assuming using Key / Value)
What would you recommend? Thanks.
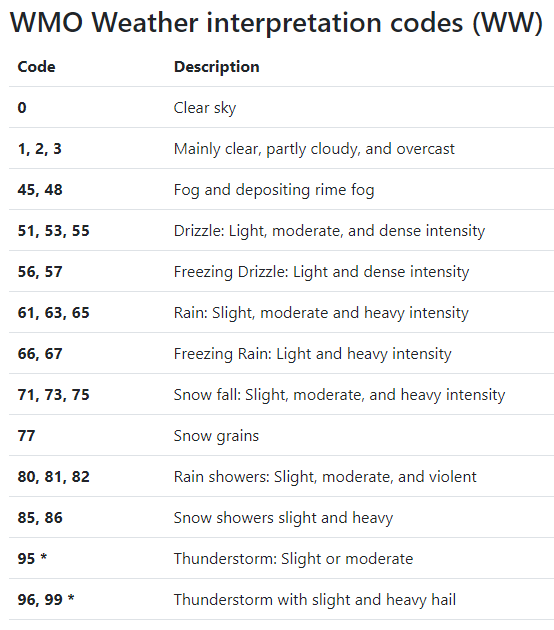
68 Replies
I'm personally thinking about using a Set.
Something like
Ah okay cool
Thank you I appreciate iut
Although just an array would be the easiest and the extra space is not really important
Definitely was not typing out 100 indexes
You can generate the code for the array
How so?
You then get
["Clear sky", "Mainly clear, partly cloudy, and overcast", "Mainly clear, partly cloudy, and overcast", "Mainly clear, partly cloudy, and overcast", , , , , , , , , , , , , , , , , , , , , , , , , , , , , , , , , , , , , , , , , , "Fog and depositing rime fog", , , "Fog and depositing rime fog"]

The one problem is
1, 2, 3 Mainly clear, partly cloudy, and overcast
I believe
1: Mainly clear
2: partly cloudy
3: overcast
Not
1: Mainly clear, partly cloudy, and overcast
2: Mainly clear, partly cloudy, and overcast
3: Mainly clear, partly cloudy, and overcast
Maybe ill just do this object and type them manually
in that case, a key/value object seems the best
Oh I see. I should have got that from "mainly clear" being nothing like "overcast" 🤭
using something like a Set?
Yeah just slap it in an object
Yeah but you will probably also want to contain information such as icons and if you're changing theming
I was actually going to try and implement that as well (icons)
I'd pack all of that information into a single object
something like this?
ahh right, otherwise with what I wrote, looping through would be 🤯
awesome thank you guys
Yeah with yours I'd make another object that holds a lookup, like my first code
these key/value pairs make most sense tbh. I overlooked and didnt think to assign object as the value
Yeah
As every item is different my first code can be ignored
Perfect thanks again 👍 ill post my codepen solution soon if you're interested in checking this out
Ping me when you do 👌
why not a map, instead of that object?
i know it is a bit more cumbersome to use, but has plenty of convenient methods, like
.has()
to check that the key existsThis might be messy
One thing is that it might only work with USA addresses
When I convert LAT/LONG into data for weather api, I use COUNTY/STATE/COUNTRY, for any address that doesnt have this, it should fail
Mind giving me some thoughts on how to improve? Thank you 👍 @ἔρως@z- ::theProblemSolver::
The key should always exist
I'm about to eat dinner and can't look too in-depth right now but it looks good
Thanks appreciate you willing to check for me 👍 pretty happy to be able to do majority of this without asking too many questions
Only had to ask question here regarding weather codes and how to get LAT/LONG from user without their input
should, unless the api changes
Would you be able to possibly give me feedback? I would really appreciate it. Looking to improve 👍 @ἔρως
you're relying too much in gps coordinates
you usually can't easily copy/see them in google maps on the phone, for example
this forces the visitor to do your job
https://open-meteo.com/en/docs/geocoding-api <-- you can pass this the name of a city, and return results
then you can allow people to select a city
also, you're setting styles in js - that's what css is for
just set a class on it, that changes the styles
also got this...
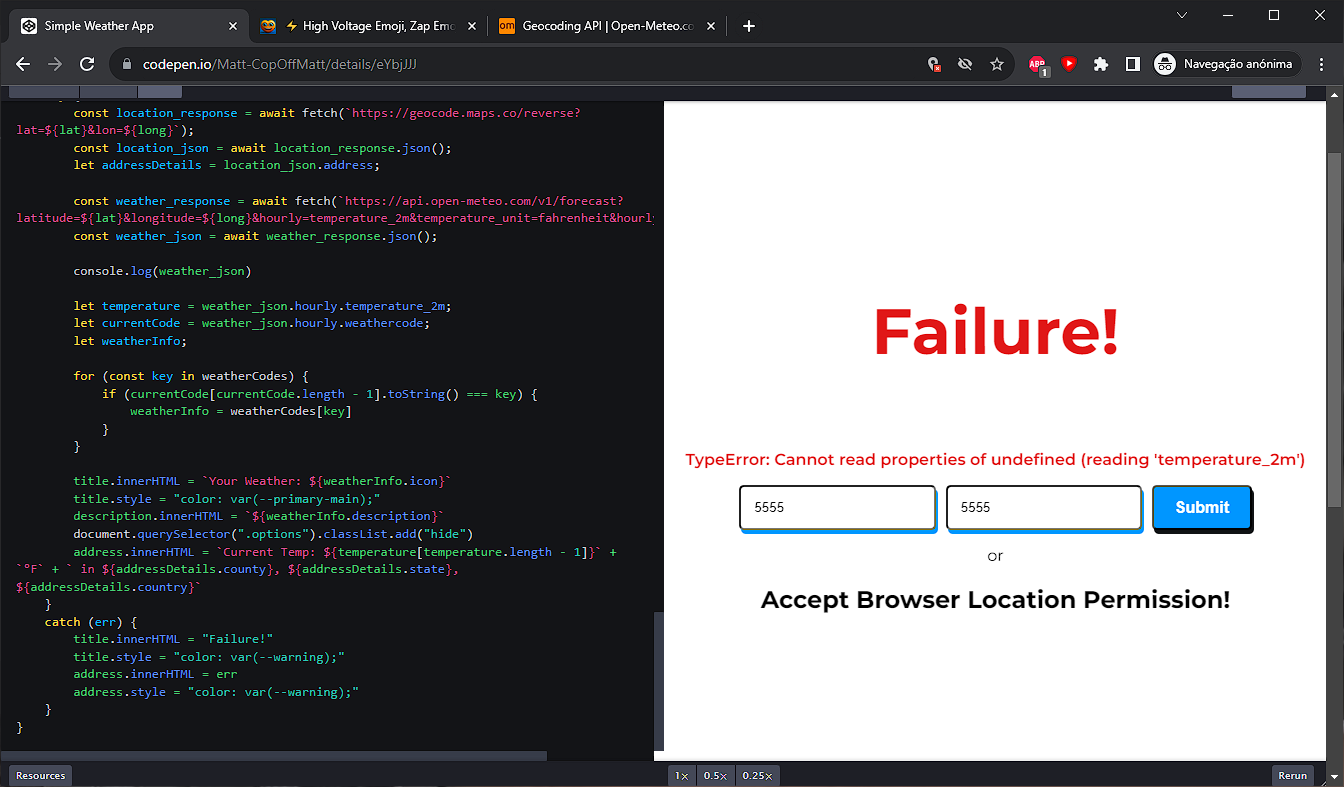
https://emojiterra.com/high-voltage/ <-- by the way, you can use this for signaling "thundering"
EmojiTerra
⚡ High Voltage Emoji, Zap Emoji, Lightning Bolt Emoji
Emoji: ⚡ High Voltage (Danger | Electric | High Voltage | Lightning | Voltage | Zap) | Categories: 🌑 Sky & Weather, 💯 Top 100
these right here:
could have
🌧️⚡
or 🌧️❄️⚡
and for the emojis, make sure you use an emoji fontI that error is because I didn’t add the logic for non-usa
The emojis and stuff were just basic, didn’t go too in-depth. This is just another practice project to learn more about working with JS
From a JS POV is there anything that should be written differently besides relying on cords?
and besides removing the css stuff?
Should the large array be moved to an external file and I retrieve the data to main?
no, it's fine there
Okay cool I’ll work on fixing some of those things thanks for your input
document.querySelector(".options").classList.add("hide")
<-- this is not bad, but you should store the result of document.querySelector(".options")
in a variableThere are a few instances where I removed the variables
My thought process was that if it’s not used more than once to not store as variable
and i would store it all outside the function
then, make the function fetch the data only
your function does waaaaaaaaaaaaaay too much
And the manipulate data outside with the result of the fetch?
outside the function, you present the result
Okay
if you have a function called
getWeather
, why are you displaying stuff from it?
it's supposed to ... get weatherTrue
Good point tbh
that's all it does: nothing else, nothing more
Just out of curiosity, are there any notable differences between fetch.then and using async functions?
also, you don't have a good way to handle errors
In terms of try/catch?
the difference is that you can just do
await fetch
in terms of the api returning something unexpected or an errorYeah but they do the same thing. Is there a performance difference or something, or just preference?
Wouldn’t it fail the try and log the error though catch
it's more of a "can i do it?" thing
Similar to
maybe, maybe not
if you had proper error handling, you could handle unexpected data
What would you suggest?
well, the function could return 2 types of data
Adding if/then and logging any fails?
a successful one, and an error one
if it is successful, display the data normally
an error one, you can have a description on it, and display it, like how you're doing now
Should I add another parameter to the function?
Or do like if / throw error within the function
no, just return
why?
just use a form, with a
submit
handlerAhh that’s what I was looking for
I was trying to figure out how to be able to also press enter for submission
Really appreciate the feedback you give me across most of my posts btw, I’ve been learning a lot
you're welcome