94 Replies
Which part are you struggling with?
the starting
Show what code you have written so far
I haven't started yet
I would suggest giving it a try and if you have specific questions on code you can't get working you can ask here.
We aren't going to write it for you.
Then you need to start. The task is fairly well described already
Start with taking the required inputs and work from there
more donuts -> less money
u solved?
I solved it
(I know it was easy, but I'm a beginner so)
I did something
I ain't sure if its correct
Post it
using System;
class Program
{
static void Main()
{
const double costPerDonut1to4 = 2.00;
const double costPerDonut5to9 = 1.75;
const double costPerDonut10OrMore = 1.50;
const double costPerDonut12OrMore = 1.00;
const double hstRate = 0.13;
const double coverCharge = 0.25;
Console.Write("Enter your last name: ");
string lastName = Console.ReadLine();
Console.Write("Enter the number of donuts you want to purchase: ");
int donutsPurchased = int.Parse(Console.ReadLine());
double totalCost = 0;
if (donutsPurchased <= 4)
{
totalCost = donutsPurchased * costPerDonut1to4;
}
else if (donutsPurchased < 10)
{
totalCost = donutsPurchased * costPerDonut5to9;
}
else
{
totalCost = donutsPurchased * costPerDonut10OrMore;
}
// Check if the customer is eligible for HST exemption
if (donutsPurchased < 12)
{
totalCost += totalCost * hstRate;
}
totalCost += coverCharge;
Console.WriteLine($"Total cost: ${totalCost:F2}");
}
}
what bout this
Tip: use $code to post code in a more readable way
Posting Code Snippets
To post a code snippet type the following:
```cs
// code here
```
Notes:
- Get an example by typing
$codegif
in the chat.
- Change the language by replacing cs
with the language of your choice (for example sql
or cpp
).
- If your code is too long, you can post it to https://paste.mod.gg/ and share the link.```c#
using System;
class Program
{
static void Main()
{
const double costPerDonut1to4 = 2.00;
const double costPerDonut5to9 = 1.75;
const double costPerDonut10OrMore = 1.50;
const double costPerDonut12OrMore = 1.00;
const double hstRate = 0.13;
const double coverCharge = 0.25;
Console.Write("Enter your last name: ");
string lastName = Console.ReadLine();
Console.Write("Enter the number of donuts you want to purchase: ");
int donutsPurchased = int.Parse(Console.ReadLine());
double totalCost = 0;
if (donutsPurchased <= 4)
{
totalCost = donutsPurchased * costPerDonut1to4;
}
else if (donutsPurchased < 10)
{
totalCost = donutsPurchased * costPerDonut5to9;
}
else
{
totalCost = donutsPurchased * costPerDonut10OrMore;
}
// Check if the customer is eligible for HST exemption
if (donutsPurchased < 12)
{
totalCost += totalCost * hstRate;
}
totalCost += coverCharge;
Console.WriteLine($"Total cost: ${totalCost:F2}");
}
}
cs not c#, and you need the 3x ` at the end too
BlazeBin
A tool for sharing your source code with the world!
$codegif
here
Looks pretty good, but there are some errors in how you calculate the total cost. Maybe try calculating it manually (with a calculator) for 15 donuts using the original table and see if it matches up, and if it doesn't, why?
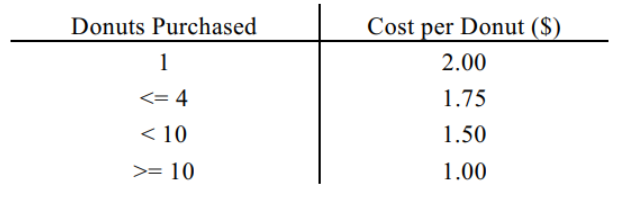
@BabySid that looks like a pretty good first attempt but I'm not sure you quite followed this table:
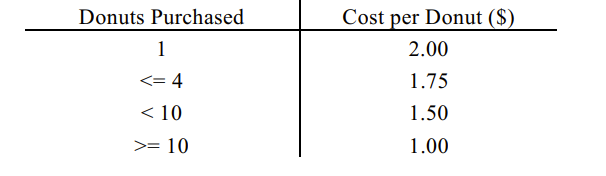
cs
hah I was first :P
🥷
I didn't understand it properly
could you explain it once more please?
Oooh wait
maybe I've misunderstood this slightly
Is this pricing $ for the total donuts or does the pricing only apply to the donuts above that level?
Like say I buy 15 donuts, does that mean it's 15*$1 = $15 or is it more than that because the earlier donuts are more expensive?
It should be the former
Based on how I read the assignment
yes its $15 plus cover charge
I thought so too, at first. But maybe not? idk
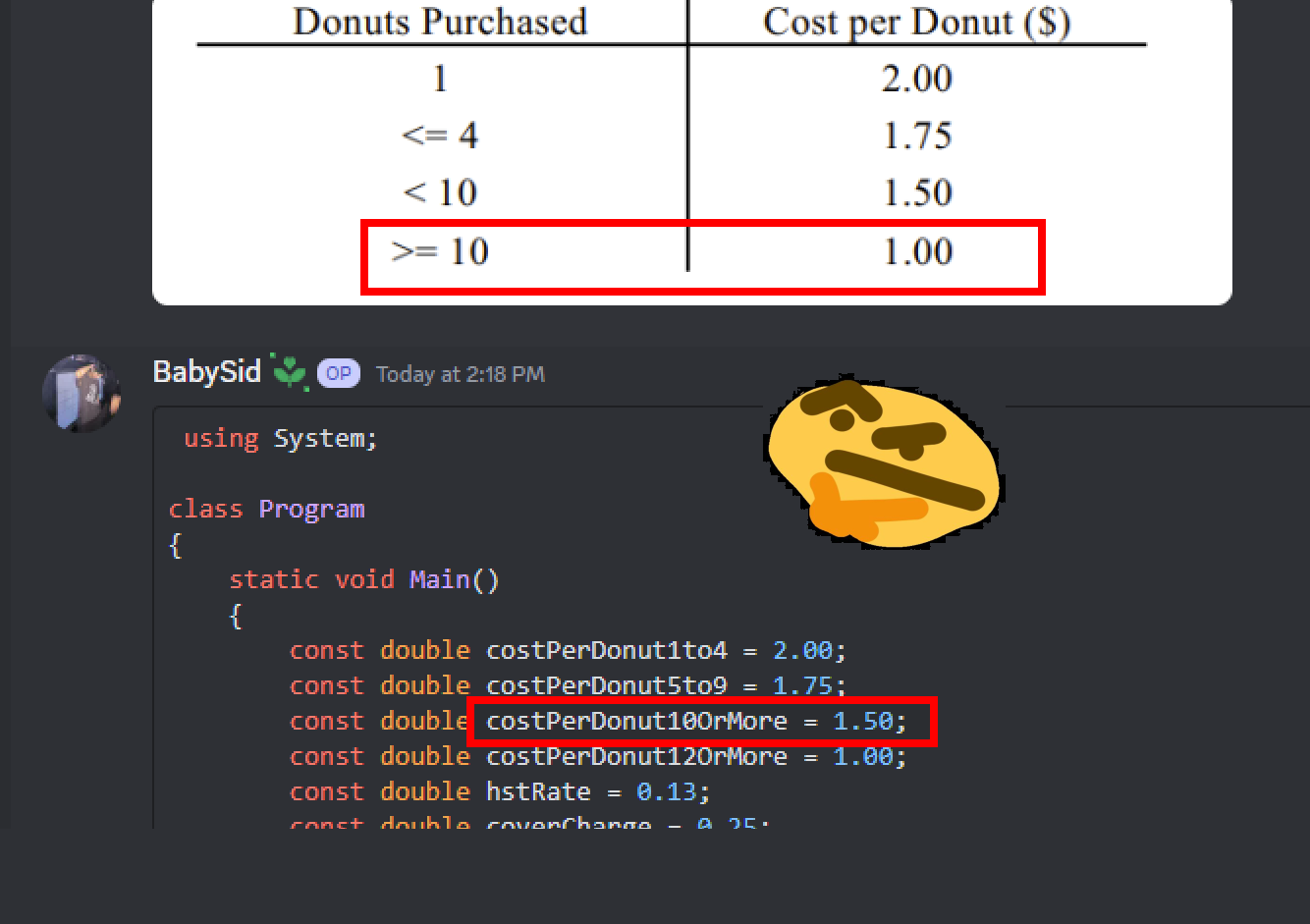
oh shi-
hold on
I see it
A single donut is also its own price, not 1-4 as a bracket
so 1 donut is 2 dollars
2donuts to 4 donuts is 1.75 dollars
5 donuts to 9 donuts is 1.50 dollars
10 donuts onwards its 1 dollar
right?
yeah
but
shi-
I'm still not sure if it's like each donut has it's own price or if all the donuts cost $1 if i buy 10 donuts
Like either all cost $1 each = $10
or 1st donut cost $2, second to fourth donut $1.75 etc. leading to a higher sum
I'd assume the simpler pricing from the wording, it's not income tax!
it's a bit ambigous
The table is pretty clear
but the problem is then it's much more expensive to buy 9 donuts than 10 lol
buy 12 donuts for the same price as 9? xD
doesn't make much sense from a business perspective
anyways, too much logic for a simple problem
🤷 I don't think it's meant to represent a legitimate business
uhgg
I need to fix this now
lol
I would use pattern matching I think to have a nice clean way of expressing the cost-per-donut calculation but for a beginner problem that's probably not a good idea.
ummmm
added some comments
Why does the hstRate calculation need changing?
oh shit
nvm
it doesn't
wow I'm stupid
it's just another way of doing it
The only other thing is just to check you're outputting all the required information at the end 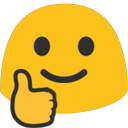
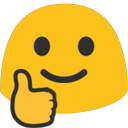
wow okay
lemem give this a whirl
Also maybe consider which data type should be used when money is involved
And as @Isaaaak pointed out that you can add the cover charge earlier, especially as the instructions say the cover charge is pre hst
oh okay
so I check for hst after adding the covercharge?
yep
Yeah 🙂
hmmm
okay
thank you so much
because the cover charge is also taxed
I'll brb
oh
cs
how about now
its working
the subtotal is correct
1. What happens if a customer orders 1 donut? In the table it says 1 donut should cost $2
2. What happens if the customers says they want 0 or a negative amount of donuts? What should happen? (what does the original question say?)
3. Which datatype should be used when we are talking about money in C#? Is double correct or is there a better one?
4. Are you outputting everything the original question says you should output?
5. Is the last "else"-statement necessary?
cs
I fixed for the 0 or negative one
first one as well
Also how would I output this ?

Same as you outputted cost, just copy that two more times and change the text + variable
(in the right order ofc)
very nice, works good
wait
I didn't comprehend this
Console.WriteLine($"Total cost: ${totalCost:F2}");
Copy this, but change it slightly so you get the right text and the right variableThere is a crashing bug in that code.
aha
gotcha
wait wha?
You have two "if"-statements here. What problems could arise?
Do you get the correct total cost if you order 1 donut?
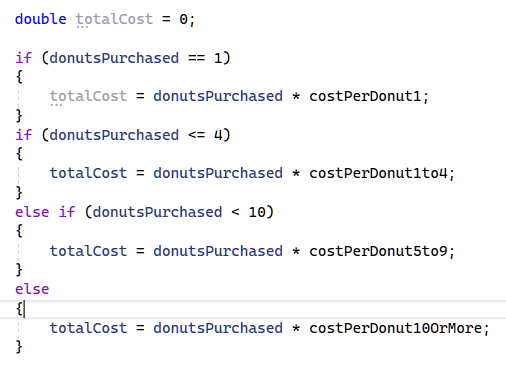
See what happens when the user types "three" instead of "3"
I don't think there are any requirements that the user should be able to input anything else other than integers?
Users never follow the rules 😉
Although I think the instructions did say you can assume it will be a valid integer.
But in other contexts you would want to think about this kind of thing.
Yep, error-handling is important
cs
it displays this as well now
😭
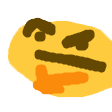
holy hsit
I'm dumb asf
cs
apologies
Turn on "warnings as errors" 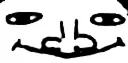
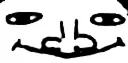
no worries ofc
ya'll are life savers
thank you so 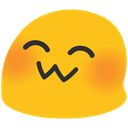
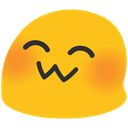
Compiler Warning (level 3) CS1717 - C#
Learn more about: Compiler Warning (level 3) CS1717
1. What happens if a customer orders 1 donut? In the table it says 1 donut should cost $2. What should the total sum be when a customer buys 1 donut (calculate manually and compare to what your program says)? If they don't add up, why?
wait nvm you fixed this one^^
2. Which data type should be used when we are talking about money in C#? Is double correct or is there a better one?
3. Is the last "else"-statement necessary?
the first one I've calculated I guess
for the second one we've been taught double only
third one 😁
ooh wait
I've found something
If we calculate 1 donut manually
we get
(2+0.25)*1.13 = $2,54
but the program says
$2.51
How come?
I'll check
just a sec
I'll give you a hint if you don't figure it out
I discovered something else as well
I still can't see this
can you figure out why 😢
I think it looks good?
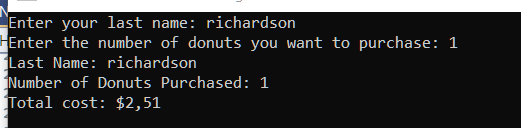
hold on
but
when I input 0 or any negative number
it doesn't show the following
for rest of em it shows the following
For me it does
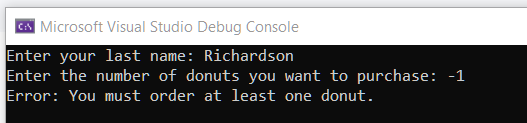
Maybe you have it so your console instantly closes when the program ends?
If so, you can just add an empty
Console.ReadLine();
under the error statement
You should put something like:
so the user isn't left staring at the screen wondering why the program doesn't finish.
oh
yeh
I fixed it
thank you so much
still wrong total cost though
hmmhm
nono
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.