TS, derive type from key of another type
Let's say we have this type
and elsewhere I want to have another type:
I want the
category
of DataDisplay
to also be 'cat' | 'dog'
but I want to know if there's a way to have it be derived from DataFile
so if that changes, the DataDisplay
will automatically account for that. like DataFile['category']
but that doesn't work because of the | null
Solution:Jump to solution
sorry, I forgot to actually post the simpler way lmao:
```typescript
type DataDisplay = {
name: string;...
5 Replies
To achieve this, you can create a type alias for the common properties between
DataFile
and DataDisplay
, and then reuse it in both types. Here's how you can do it:
In this code, we define a CommonData
type alias for the shared properties (name
and id
). Then, in the DataFile
type, we use a union type to represent either the common properties along with the category or null
.
In the DataDisplay
type, we use conditional types to check if DataFile
is null
. If it is, the category
property is set to never
, effectively excluding it from DataDisplay
. If it's not null
, we extract the category
from DataFile
. This way, if the DataFile
type changes, DataDisplay
will automatically adjust to it.
Here's how you can use these types:
If you change the category
type in DataFile
, it will automatically reflect in DataDisplay
.Two things:
I think I found a simpler way. And I now realize it won't work, because in the actual thing I'm building for work that I can't share has to factor in translation stuff so the strings 'dog' or 'cat' could ending being different once translated into other values
Hi @Donny D I did not even look at your question. I was experimenting to see if the chat gpt answer would fool/satisfy you
I just copied and pasted
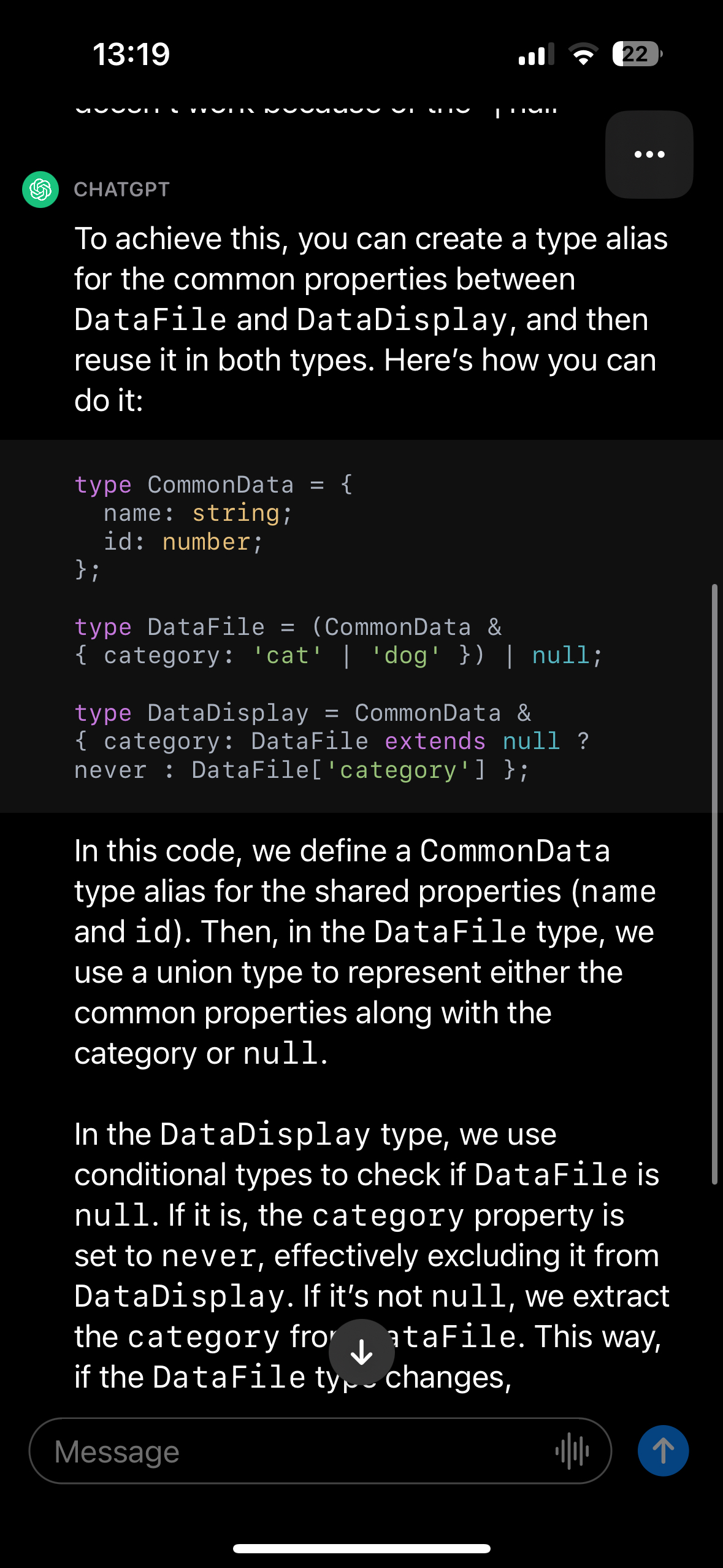
Solution
sorry, I forgot to actually post the simpler way lmao: