8 Replies
There are 4 "parts" to a for statement
int i = 1
is the "initializer, it runs once before the loop starts
i <= 10
is the condition, this needs to be true for the loop to continue and is checked at the beginning of every iteration
i += 2
is the post-iteration step, it runs at the end of each iteration, before the next one.
Finally, you have the "body", thats the code between { }
that dictates what to do in each loop iterationA for loop is relatively simple, it loops a piece of code, and at the end of each cycle at does something and checks whether it should continue looping. A for loop consists of four parts:
- 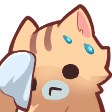
int i = 1;
: the initializer of the loop. It runs once at the start of the loop, and in this case declares a variable called i
and sets its value to 1
.
- i <= 10;
: the condition. This is the condition which determines whether the loop should continue looping, and it runs once at the start of the loop to determine whether the loop should run at all, and then once after every cycle. In this case, it checks whether the variable i
is less than or equal to 10
.
- i += 2
: the... actually I don't know what this is called, so I'll just call it the incrementor. This runs after each cycle of the loop and usually manipulates the variable declared in the initializer. In this case, it adds 2
to the variable i
.
- Console.WriteLine(i);
: the body. This is what the loop should do on every cycle. In this case, it writes the value of the variable i
to the console.
The total effect of this loop is that a variable i
is declared and set to 1
, then it writes the value of i
and increments it by 2 and keeps doing this until i
is not less than or equal to 10 (i.e. just greater than 10).
(I spent a lot of time writing that but you sniped me 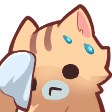
ty
thanks for the help guys, im new to c sharp
for is a classic loop that exists in many languages, so its good to understand
javascript has it, C has it, rust has it, etc
ok
thx
$close
Use the /close command to mark a forum thread as answered
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.