✅ Can't use Machine Learning Model
Heyo! So I wanted to try out using an AI model to sort some pictures into one of two categories, the app I'm trying to use it in is a Console App in .NET Framework 4.7.2 targeted for x64, I've added a Machine Learning Model (called Stone_Diff), trained it and it works great, but I can't figure out how to use it. I copied the sample code the setup gave me, but it comes up as "The type or namespace name 'Stone_Diff' could not be found" and "The name 'Stone_Diff' does not exist in the current context". Here's the code I'm using:
Why's it erroring out, do I need to manually add some references or something? If so, I couldn't find how to do it with the normal Add Referenced menu, nor could I find a way to get
using Stone_Diff;
to work. Thank you for reading!74 Replies
Where exactly did you find that model? Google gives me nothing.
Also, any particular reason you're on .NET Framework and not modern .NET?
made it
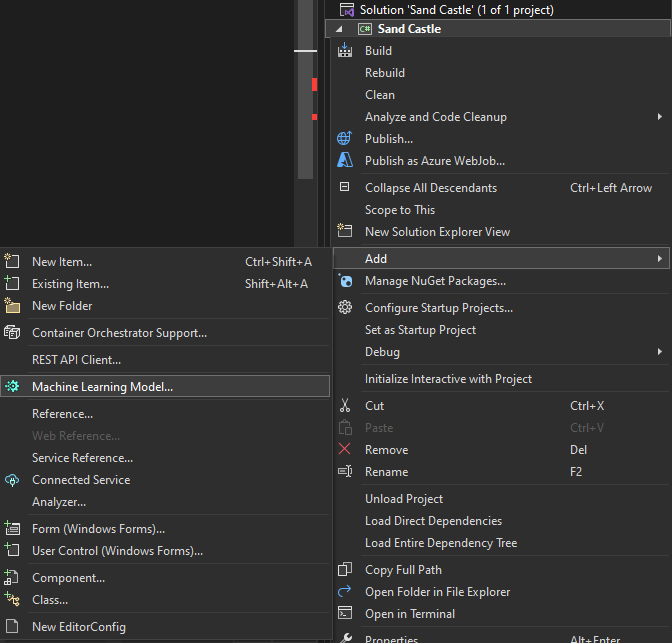
this is just a testing app for a winforms app that is my actual main project, didn't wanna add anything into that before knowing whether this would be a viable solution
The type or namespace name 'Stone_Diff' could not be foundthat usually means you forgot a
using
statement or a project referencetried both of those, same issue
¯\_(ツ)_/¯

also as a reference I can't find it in any of the lists
if you open up the generated
consumption
file, what does that look like?ok there we go
so the class is called
Stone_Diff
and is in the Sand_Castle
namespace
so where you are trying to use it, you need a using Sand_Castle
unless you're already in that namespacealready am
here's the main program
ok idk then, my only remaining guess is that this isn't compatible with framework
the few resources I found on this said it should work for framework
idk :(
can you reach this page?
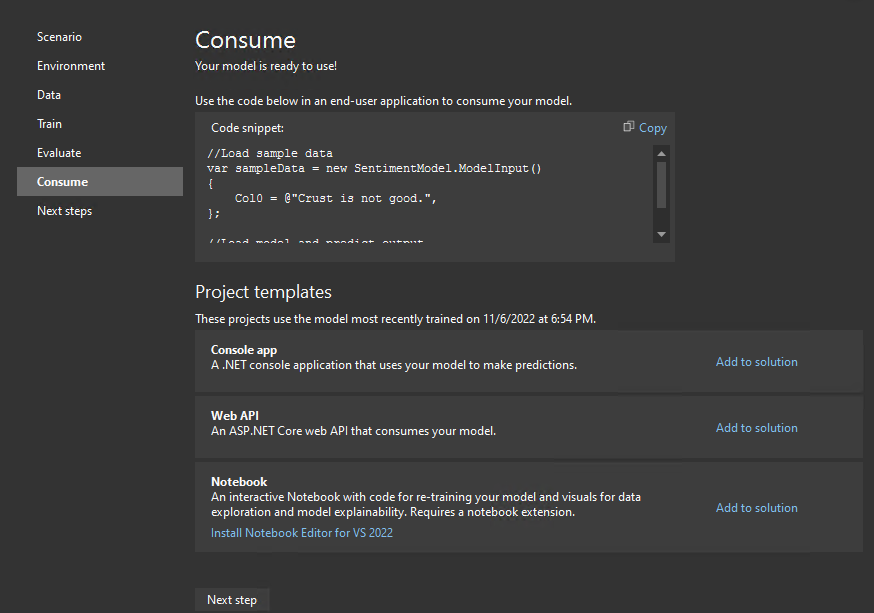
if so, what happens if you click "add to solution" for console app
it added a new project, this is the Program.cs
and the csproj for that project?
got 23 warnings as well lol
warnings are warnings, not errors
mhm, so thats .net 6
Microsoft.ML
targets .netstandard 2.0 so it should work on framework, at least that packagealso got this long ass warning
yeah because you are using version 4 and version 6 of that package
ah fair enough
not a good idea to mix modern .net with framework 😄
fair, but still, there must be a way to use the ML model with .NET framework
sure, but at the same time its no suprise the tooling (which was all made AFTER framework as discontinued) doesnt support it
Especially since it should theoretically work
Impossible to find resources on it thou, since again the entire thing was made after framework was already discontinued, so all guides/tutorials/articles all assume modern .net
"ML.NET runs on Windows, Linux, and macOS using .NET Core, or Windows using .NET Framework. 64 bit is supported on all platforms."
From Microsoft's Learn website
https://learn.microsoft.com/en-us/dotnet/machine-learning/how-does-mldotnet-work
What is ML.NET and how does it work? - ML.NET
ML.NET gives you the ability to add machine learning to .NET applications, in either online or offline scenarios. With this capability, you can make automatic predictions using the data available to your application without having to be connected to a network to use ML.NET. This article explains the basics of machine learning in ML.NET.
yup
and the package does indeed target .netstandard 2.0
but I googled for a good 10 minutes and found not a single example for .net framework 😛
especially not for those .mbconfig models
Well I needed a custom model, this seemed like the only realistic way for me to get that
I'm not saying you did anything wrong, I'm just saying the tooling might not support what you are trying to do
damn, I always get myself in these situations
the
consumption
file should give you access to the Stone_Diff
class, but perhaps its not being compiledso then is there a way to integrate a standard .net project into a framework project?
wdym?
.netstandard 2.0, yes
.net6, no
if I were to use the model in a separate project, could I send an input to that project, have it run and use the output in my framework program?
Why are you still using framework 7 years after its "death" thou?
thats my primary question, tbh
winforms, I adore winforms and it's what I learnt in university, nothing easier to work with for me than winforms, apart from finding myself in situations like these :D
and winforms works fine in modern .net
so uh.. again, why are you on framework?
also what I learned in uni
Thats a pretty bad excuse tbh
"I refuse to learn new things" is what it boils down to
more like I have a big project already in framework, I wouldn't even know where to start with migrating it and I sure as hell ain't rewriting all of it lol
also, if I were to set this to build as well, could it help?
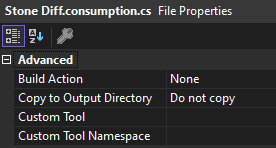
check what build action is on another .cs file
on the Pogram.cs files it's on Compile
ok, try setting it to compile
it built now, the unknown errors are gone, but when running it it throws this
in the consumption.cs
hm, did you remove the generated project?
the one for .net 6
if not, do that, and clean solution
done that, same issue
well, you'll need to track down why its trying to import a .net 6 version of
System.Runtime.CompilerServices.Unsafe
exquisite question
removing the .vs folder in the project might help
did that, cleaned and rebuilt, got this
if I just make a new project, retrain a model, never add a sample console app and try again lol
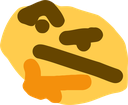
worth a try 🙂
might even be able to just copy the trained model over
hmm how would I import it into the new project
just copy the file
and add it to the project declaration
but eh, if it wasnt too bad retraining works too
it's just a couple hours
using CPU training on ~40k images
I mean, copying the file and fixing the csproj should be... 2-3 minutes ? 😄
I copied the .mbconfig and all its .cs files, as well as the model .zip itself
yep, then look in your original csproj and copy the related entries
hoookay, added all the needed NuGet packages, fixed a couple file path issues, same error as before
try looking inside the zip file
i have no idea whats in there
but maybe there is some .net 6 stuff
a few .key files, a few files with no extension, a TXT with my two categories it's supposed to sort stuff in, and a TXT called Version with the text
2.0.0-preview.22314.3+f99825a1bfdbf45463fff994735caee6eacdce78
no clue as to what any of them do
ooh okay, so I've updated all the nuget packages, there was missing a dll that I copied from the previous project, now I'm getting this output in the console
cool, so it actually runs
yup, now it runs
no idea what that output is though
different from what it showed in the sample output of the .mbconfig
Sand_Castle.Stone_Diff+ModelOutput
looks like what happens if someone does Console.WriteLine(x)
on an object that doesnt override tostringHmm
So then how do I use the output lmao
¯\_(ツ)_/¯
Can you show your program.cs code?
Just this again
Console.WriteLine(Convert.ToString(result));
kekw
thats the source of your problem
you need to access the properties on result
, and/or implement ToString
on itWell I didn't know how exactly it would output lol
Okay, will figure this out somehow, thank you for your help though <3
just in case you're wondering, I got it to work fully and it does exactly what I wanted it to, wrote it in as
Console.WriteLine(result.PredictedLabel + " with " + result.Score[1] + "confidence.");
I was able to remove the .mbconfig file and its associated .cs files altogether, I just copy-pasted the class from consumption into the Program.cs and all is well, thank you again for your help ^-^