✅ How can I make my code reusable?
Hi!
I asked a question before to calculate the average of 5 text boxes in one textbox. I wrote a code:
I want to use this in more situations but I don't want to define every textbox and make it really redundant.
6 Replies
The simplest way would be to have
CalculateAverage
take in a list of values, instead of know all the values itselfJust make a list that holds each textboxvalue and then loop through the list and divide that by 5.
assuming you want to be be able to use this method in other forms, that might have different boxes and even other amounts of it
Thanks the idea, I will try it guys. But how will I tell him to which textboxes needed to be calculated? Mostly there are 5 or 20 textboxes will be calculated.
I draw an example:
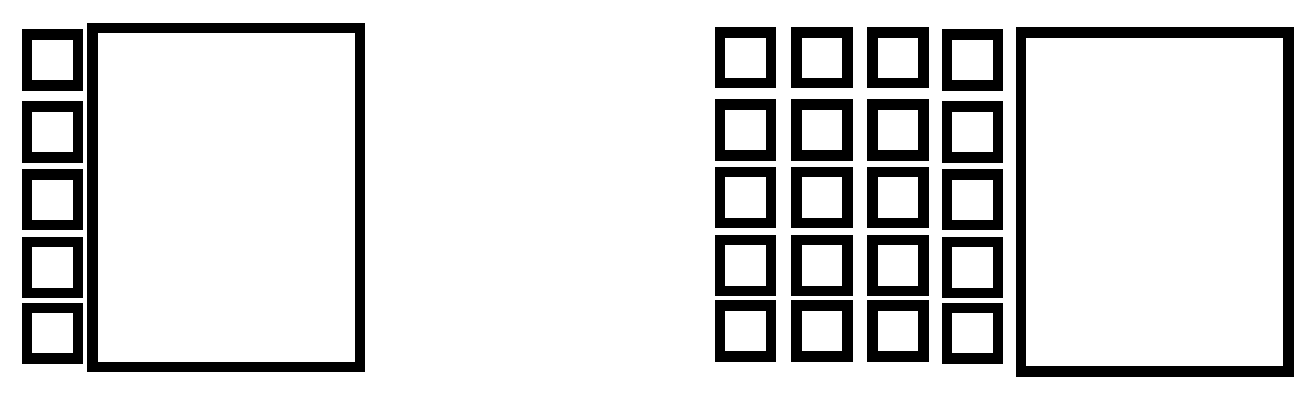
List<TextBox> and then grab the ones that are needed?
Ohh, okey