✅ What is the best way to store basic C#?
Hi, I'm testing my C# code and learning from it. For example deserializing and serializing JSON using system.text.json, I have the working code in program.cs. Now i want to store it in a folder preferably same vscode folder to review it in future and test some other code. What's the best way to do it?
29 Replies
Normally you'd just make another project
C# and .NET are project based, not file based (the way python or js are)
And just having a bunch of .cs files lying about is
* not very practical
* hard to find what you are looking for
* will cause problems for the compiler
so I'd probably use something like obsidian or some other "note-taking" app to keep snippets in, if thats what you are after
or, make a new project
Exactly, I'm using obsedian currently to take notes
But was looking for a solution to keep all the code in one project and use it to test in program.cs
okay
here is what I do
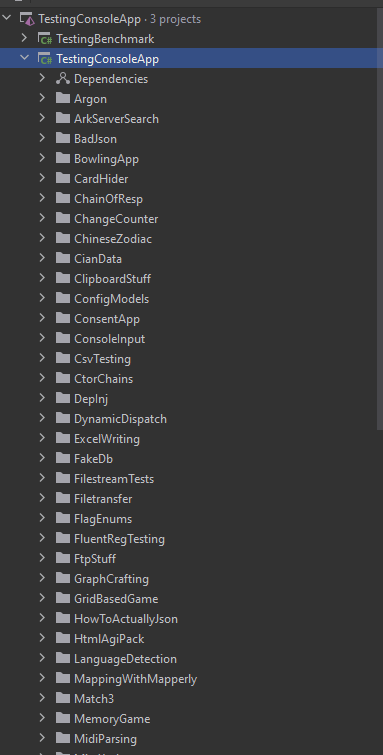
all those folders are just... folders. My program.cs looks like this
so to avoid making a new project, every small snippet I make in its own "Program" class in its own namespace, and I just call that method from my real program method
I have ~200 of these "mini-programs" inside this project
i tried to create a folder and a class in it
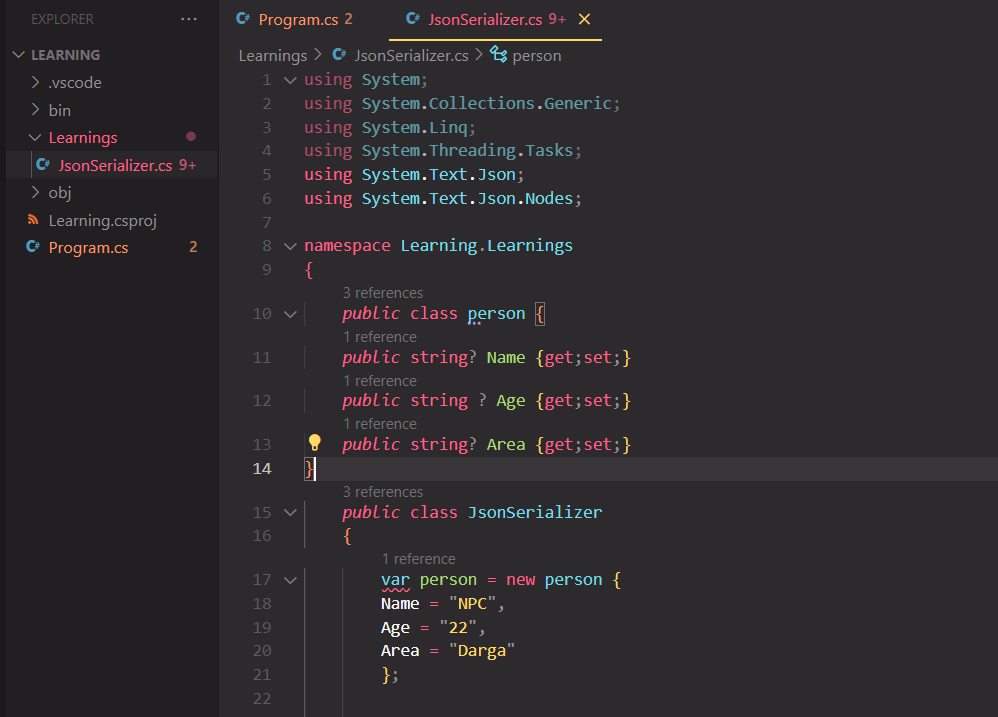
inside the folder what's the structure?
waitttt that's brilliant, I also want to create something like Learnings.JsonSerializer.Run(). how do i do it?
do i like create a main method in Jsonserializer.cs and call it from the Main program.cs?
lemme try that
@Pobiega can you let me know what I should keep in JSonSerializer.cs class to call it from my program.cs file?
Hm, well first, don't call it
JsonSerializer
that name will conflict with the System.Text.Json
class by the same name 😄
What I would do is make it a bit nestedGotcha, will change it's name
Learnings.JsonSerialization.Program.Run()
the first two are namespaces, then a class, then the run methodAlright, let me try that
I have a few other important tips for you 🙂
open up the terminal in VS Code and write
dotnet new editorconfig
please go ahead
alr
this will create a default .NET editorconfig file that will help you stick to the default rules for naming and code style
next, dont use
namespace xxx { ... }
anymore
prefer namespace xxx;
this is called "file scoped namespaces" and is the defaultit did create a editorconfig file
great
classes are always named with
PascalCase
meaning person
should be Person
so you mean not to keep the classes and program main method inside namespace {} but just create namespace xxx; and create classes etc??
in fact, Im sure you have a C# code formatter installed with VS Code?
point taken
file-scoped namespaces apply to everything in that file
so there is no difference between
and
except that the top one is more readable, since you have one less level of indentation
i see, gotcha! this is good will stick to namespace xxx; one
what formatter do you use? i have prettier but i think thats only for html and stuff
Well, I don't use VSCode 😛
Rider is my preferred IDE, with VS being my second. VS Code is a distant third
but if I had to use VSCode, I'd use the C# Devkit
lets see if we have a tag for it... $vscode
Follow the instructions here on getting started with DevKit for C# in VSCode: https://code.visualstudio.com/docs/csharp/get-started
Get started with C# and .NET in Visual Studio Code
Getting Started with C# and .NET Development in Visual Studio Code
there you go
I'd highly recommend using regular VS if you can thou
gotcha
I have VS installed
there ya go
i can use it too, it's like when i do simple tests like creating arrays or something i learn on the go when i'd want to test i feel using vs easier
but if i had to use VS then basically i'd create console app and just like what you did create multiple folder for each concept and execute it from main program.cs?
that wouldnt change based on IDE/editor
if you dont want to make new projects, thats what you need to do.
and even then, you're limited to one project type (usually console)
if you want to experiment with ASP.NET Core or WPF, that must be a new project
right right, I just want one single project for everything C# concepts
Thanks mate! learnt a lot
Yep, it's working fine! just tested it.