✅ xUnit | Saved entity not found.
Hello, this time coming with yet again problems with tests. So I'm testing my deleting route, so i create the related entity in database, get it's hash - the delete route takes hash as an path parameter, and pas it down to the repository for delete. The creation works, checked the context if it exists and it does, but for whatever reason it cannot be found and deleted. I think it may be problem with how i've set up the contexts.
Repository
Test
21 Replies
what errors do you get? Or what status code does the http method you invoke respond with?
Oh sorry forgot to include that:
Right now:
But in some iteration of my tries it also said
Sequence is empty
or something like thatokay, Sequence is empty is from
.Single()
not finding anything
and value cannot be null
is almost guaranteed from _dataContext.PaymentDatas.Remove(report);
where report
is null
put some breakpoints down and check whats going on
you said you verified that the stuff worked, for sure?
ie, putting a breakpoint after that savechanges and manually looked?Oke, give me a minute for that
Yes, i especially changed the sqlite file name to something else and ran only this test, and it was created in it
okay
Huh, it suddenly started working?
you sure you had the latest version compiled and running?
because
value cannot be null
should not be possible, since you fetched the value yusing Single()
and not SingleOrDefault()
I think so? Well if running single test doesnt recompile the code then i guess i wouldnt have the updated code
I hate when stuff suddenly starts working because i dont know what was broken 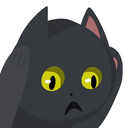
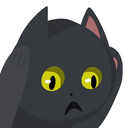
¯\_(ツ)_/¯
depends how you run it
via
dotnet test
im not sure it rebuilds everythingRunning it through visual studio so no idea either
okay, then it should, I imagine
but not 100%
Well nonetheless problem is gone for now, thanks once again!
yay
Oh on the topic of tests, i was wondering, if what i did to get the context in tests is the right way?
this sits in constructor of test, is there maybe better way to do it so i can access it in each and every test i create?
uhm, its certainly one way
I'd probably just resolve it from the service provider
So instead of calling the context dfirectly i should use the service for example creation of entities?
no, using the context is fine
I would highly recommend you stop using query syntax thou 😄
Oh thaqt was just me looking for any option that would fetch, i will switch to Fluent API asap
and prefer to use the async methods
so
await... SingleAsync();
