✅ Learning C# through console app
I have decided to take a step back from Avalonia to just focus on learning C# through a console application. This is all I have in my console app. I cannot for the life of me figure out how to get access to the username stored within the UserInfo that is returned from the load function. Any suggestions would be great
70 Replies
You're not actually setting
UserName
in the constructor of Program
, that's a variable called UserName
which is shadowing the property.so remove UserInfo from the decorator?
wdym "decorator"?
yep
ok it still doesn't like
UserInfo(db.LoadUser());
so what do I have wrong there?UserInfo
is your class. Calling it like that tries to invoke its constructor
but you only have the default parameterless constructor for that classI'm not going to lie. I was modeling this after the picture of my avalonia code
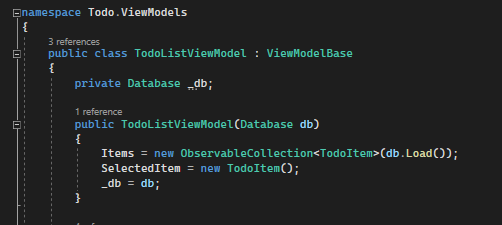
well, I suggest you stop doing that
bet
what do you mean?
I mean exactly that. Look at your UserInfo class.
do you see any constructors?
that's how I was taught to do those. I assume no? What's the proper way to do it?
no thats fine
its a very basic mutable POCO
that's even how the Todo guide on avalonia's website says to do it
poco?
this isnt avalonia.
right
"plain old Csharp object"
ah. nice acronym
its essentially a simple object
it just stores some data.
but look at the code you suggested
UserInfo(db.LoadUser());
what do you think this does?
it has 2 parts, the UserInfo(...)
and the db.LoadUser()
tries to pass a database function to a class
almost, but not quite
it runs the function and tries to pass the result to the constructor of your class
ok. that's not the behaviour I wanted. I wanted it to return the user object from the json file
thats what you are doing, more or less
hm.. ok
I think I'm doign this wrong anyways. The program needs to create a new json file with a
"setup": 0
thing in it. This is then used in the Program.cs file to check whether the user has setup their account or not. If not, then it launches a setup, otherwise, it pulls the user's username from the userinfo object from the json file
this is how my brain imagines this is supposed to go. What do you think?that seems very convoluted
well I missed creating the json file. fuck
isnt it a lot easier to just check if the
user.json
file exists or not?yea true
didn't think about that
and even abstract that
ok how do I abstract it?
well, the file might exist but contain invalid data. so try to deserialize it, and if that fails, either throw or return null or something
somehow indicate to your program that we need to create and save a new user
ok let me see what I can come up with
hint: a method that returns a
User?
seems reasonablewhat do you think about this?
Pretty good, but I'd like to change a few things
first,
UserInfo
, can we rename that to User
?
CheckUser
actually returns the user, so maybe.. LoadUser
or GetSavedUser
?
and finally, you are passing a Database db
into that method but never using it
I'd probably write it like...
that's because I have a database class in
/Services/Database.cs
that has a LoadUser funcitonYeah but you never use it 🙂
I have a database class that has a load user function. It's supposed to return the user, and then the UserName in the Program.cs file is supposed to set UserName equal to the username that is stored in the json file after created. If the file doesn't exist, then it returns a blank user. Can we use that instead?
I'm sorta thinking this new method can fully replace that one
you could move LoadUser and SaveUser into your database class if you wanted to
ok so going with my database class, how would I use that?
note that I assume you changed your db loaduser here to return null
no? it returns an empty user. I'll go change it
Yeah that doesnt really make sense
if you ask me
because then we can't easily check if we need to make a new user or not
I changed it to return null
great
again, I'll post my method for this
its a good idea to wrap any
File.Read/Write
methods in try/catch
since file IO can fail for whatever reason
you can remove the printing and static and const file path stuff as neededok I updated it to be wrapped in the try/catch
ok so I've started a class in
/UserSetup/UserSetup.cs
named UserSetup
How do I call that into the else statement in my Program.cs?Why is that its own class?
because I like to keep my code and program parts separate
This is somewhat cursed to the honest
You are running all your app logic in the program constructor
please don't do that.
And if you just want to have a way to create users, make a
static User CreateUser()
method, either in UserSetup
or in User
itselfok I made it static. How do I call it in the else statement in program.cs
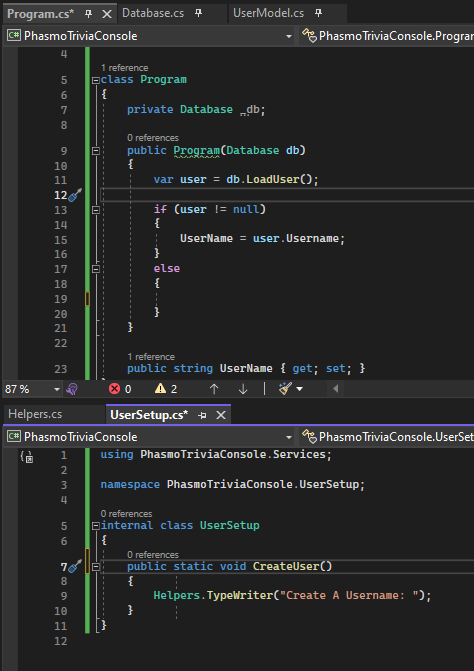
UserSetup.CreateUser()
but it returns a void atm, so thats not good
Actually, lets take a step back. Do you understand methods, return types and constructors?it doesn't like this
how so

Ah, you have a namespace AND a type both called
UserSetup
thats a no-noI didn't do anything. It generated that itself when I created the file
well yes
but if you make a folder and a type in that folder have the same name...
thats what you get
the default convention is that your directory structure => your namespaces
ok I fixed it. I just renamed the file and put in the using statement
Pobiega
Actually, lets take a step back. Do you understand methods, return types and constructors?
Quoted by
<@105026391237480448> from #Learning C# through console app (click here)
React with ❌ to remove this embed.
I don't ask to be an ass, it just seems that sometimes you just throw code at the screen and see what sticks kinda
Like the
UserInfo(db.LoadUser())
thing aboveI give a type like string, bool, User when one is needed. Otherwise, I just use void when I don't need to return anything
Well yes.
And you understand that calling a method will return something, unless that methods type is void, right? And thats how we pass information around
yes
So explain to me the reason why
CreateUser
would have a void return type?I changed it to return a user so that program can still access the user
good
thank you for your help 🙂
so I just tried to run my program to test what I have so far, and it told me that Program doesn't have a static main method suitable for entry point so what did I do wrong here?
so apparently I'm missing
so how would I work that into my Program.cs file here?
Usually
Program
doesn't have a constructor, but if you do want to have that then you could do this instead
You should really not be calling GameMenu.ShowMenu
in the constructor.Yeah move all your logic out of the constructor please
What you are doing is an anti pattern
so it would be this instead?
if this is how it's done, then how do I get access to Database db when I can't put it in the parameters?
could I pass the database through main like this
?
or would it be like this?
after testing, this last one i posted is a working solution. I'm not sure if it's the best way to go about it, however, it works