CS0120 - Object refrence required for the non static field, method, or property "object.Equals(obj)"
Hello! I am a self learning programmer beginning with c#.
I am trying to code a randomized Math Quiz in a Console app, and i am currently trying to validate the user input and then have it print "Correct" or "False" depending on the answer.
Now, im not sure as how to approach this, but i have a general idea of the code and i coded it, its just that i have no idea how to make it check whether the answer was correct, and it gives me the aforementioned error.
Heres my code:
// START HERE
Console.WriteLine("Welcome to this Math Quiz!");
Console.WriteLine("This is your first question");
// RNG
Random rnd = new Random();
float random1 = rnd.Next(-100, 100);
float random2 = rnd.Next(-100, 100);
Console.WriteLine("{0} + {1}?", random1, random2);
// Checks if answer is correct
float answer = random1 + random2;
Convert.ToBoolean(answer);
if (Console.ReadLine(Equals(answer)))
{
Console.WriteLine("Correct!");
}
else
{
Console.WriteLine("False!");
}
26 Replies
if (Console.ReadLine(Equals(answer)))
will be your problemyeah i know that, i just need a fix
i know the cause i just dont know the solution
You're using Equals incorrectly, https://learn.microsoft.com/en-us/dotnet/api/system.string.equals?view=net-7.0
String.Equals Method (System)
Determines whether two String objects have the same value.
so what would i use to detect the user input?
You can use Equals, check the link to see the correct syntax
Equals(String)?
That's as a member of the string class, i.e. You'd have to call
myStringOne.Equals(myStringTwo)
not just Equals(myStringTwo)
(how would it know what it's comparing with?)
Check the code examples in the link and you'll see how it's being used// Checks if answer is correct
int answer = random1 + random2;
Convert.ToBoolean(answer);
int answeruser = Console.ReadLine();
if (answeruser.Equals(answer))
{
Console.WriteLine("Correct!");
}
else
{
Console.WriteLine("False!");
}
so this is the improved code, the issue now is that it cant convert "string" to "int", how do i fix this?
@SinFluxx
Convert.ToInt32(answeruser) should work right?Sort of 🙂 TryParse is what you should use instead though: https://learn.microsoft.com/en-us/dotnet/api/system.int32.tryparse?view=net-7.0
Int32.TryParse Method (System)
Converts the string representation of a number to its 32-bit signed integer equivalent. A return value indicates whether the operation succeeded.
Tryparse isnt a command in the version i use
4.8 net c# 7.8? i think
Convert works fine, now the issue seems to be that it cant make an "answer"
it doesnt seem to detect the correct answer, it just prints false, even though the answer is correct
TryParse should be available, the issue with convert is if a non numeric value is entered you'll hit exceptions
itellisense doesnt display it and its not registered as a command for some reason
i think convert works fine for my purposes, i just cant figure out why the code doesnt seem to detect the correct answer as correct
Convert.ToBoolean(answer);
What are you trying to achieve with that line? And why are you using float
for the number to guess but converting the user's guess to an int
?i wrote Convert.ToBoolean due to an error thats somehow fixxed now ´without it
the second point is just a mistake
Correct those and try again then
still doesnt detect it as a correct answer
// START HERE
Console.WriteLine("Welcome to this Math Quiz!");
Console.WriteLine("This is your first question");
// RNG
Random rnd = new Random();
int random1 = rnd.Next(-100, 100);
int random2 = rnd.Next(-100, 100);
Console.WriteLine("{0} - {1}?", random1, random2);
// Checks if answer is correct
float answer = random1 - random2;
string answeruser = Console.ReadLine();
Convert.ToInt32(answeruser);
if (answeruser.Equals(answer))
{
Console.WriteLine("Correct!");
}
else
{
Console.WriteLine("False!");
}
the code im usingfloat answer = random1 - random2;
whats with that
It's a float
so convert to int?
Just change
float
to int
still doesnt work
sorry, it's because your
Convert.ToInt32(answeruser);
line isn't doing anything, it needs to assign it to a variable, so you could just get rid of:
And replace it with:
int answeruser = Convert.ToInt32(Console.ReadLine());
it works now, thanks man
no problem 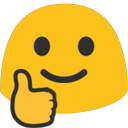
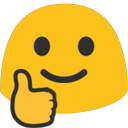
Use the /close command to mark a forum thread as answered