Doesn't DiscordAPI reflect the latest role position?
I made a test guild for my bots.
The guild has this roles scoreboard(?)
- Owner
- Mod
- Member
Guild has these members:
- Me
- My bot
- Tom
I gave Tom "Member" role (its position is "1")
while I have "Owner" role (its position is "3")
This is a code the bot has:
GuildID is my guild
UserID is Tom's ID.
Then, in my anothor guild, (my bot belongs to)
I sent "test".
Console:
It is very a result I had expected.
Then, I gave Tom "Mod" role (its position is "2")
I send "test" in the anothor guild again.
Console:
The result was not changed.
I can't understand.
2 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!Thanks for your answer, but the problem is still here.
Current code:
Also, I found when the code gets restarted, He gets the latest one.
Now I am thinking of one bulldozing idea:
When processing finished
this way
?
Yes it is!
Thanks all you guys and have a nice day!
process.exit(0);
so that every time the command get called, my bot can get Tom/Himself's latest position.
completely 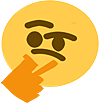