how i can use custom queue
how i can use custom queue
45 Replies
GG @Ghos't, you just advanced to level 2!
It won't work correctly, I forgot that a lot of things use database inside the package, I think the right thing would be to do everything customized
In the next few days I will try to remove most of the parts where the database is used outside
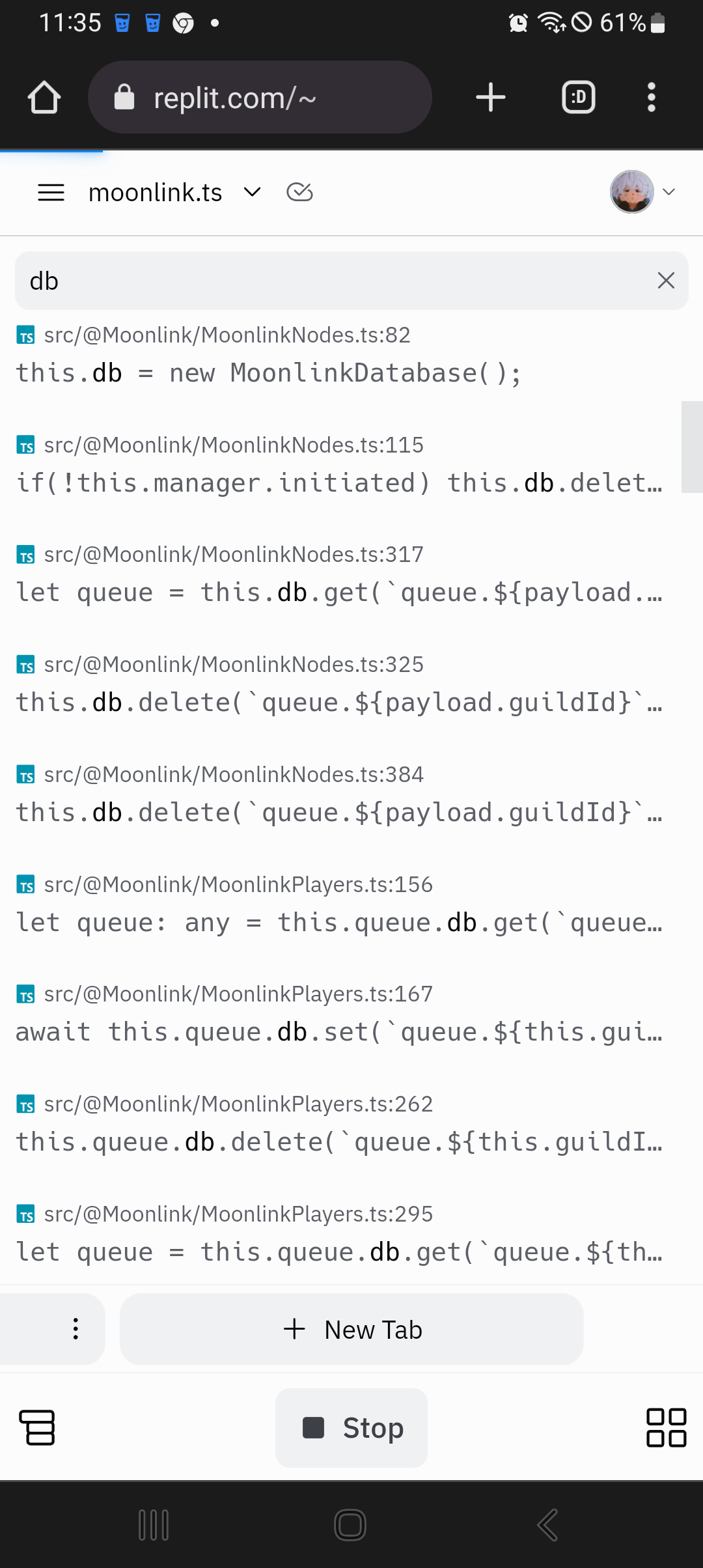
afraid-scarletOP•2y ago
@1Lucas1.apk didu fix it ?
@1Lucas1.apk when i use custom queue the pause not working and return true
When you use the default queue, what error does using multiple bots cause?
@Ghos't
afraid-scarletOP•2y ago
Other bots start using a single queue so there won't be a dedicated queue for each bot but I don't have this problem with distube
Eu acho que podemos contonar fazendo uma coisa
Vou voltar daqui a pouco
I spoke in Portuguese, I'll be back in a little while, I already know what to do to get around this
afraid-scarletOP•2y ago
No problem, I will translate it
take your time
@Ghos't you don't need to use the custom queue, delete that part there, and use the default that I changed the way it stores the data, it will separate a json for each clientId :), Have a great day, download the new version for apply this change
I tested it and it's working correctly
afraid-scarletOP•2y ago
thanks man
You are the best
afraid-scarletOP•2y ago
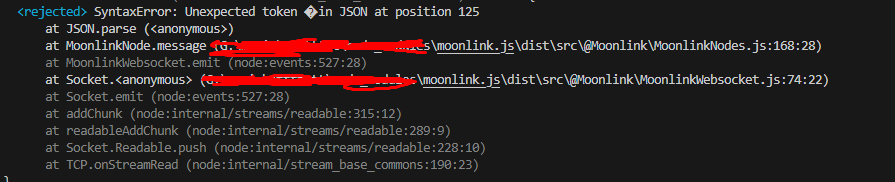
afraid-scarletOP•2y ago
@1Lucas1.apk
Did the error appear again?
afraid-scarletOP•2y ago
yes and pause not working idk why
queue.pause()
I was trying to see the problem since the time you sent me that last message
Only a little problem appeared in the IDE
I don't know where the error comes from
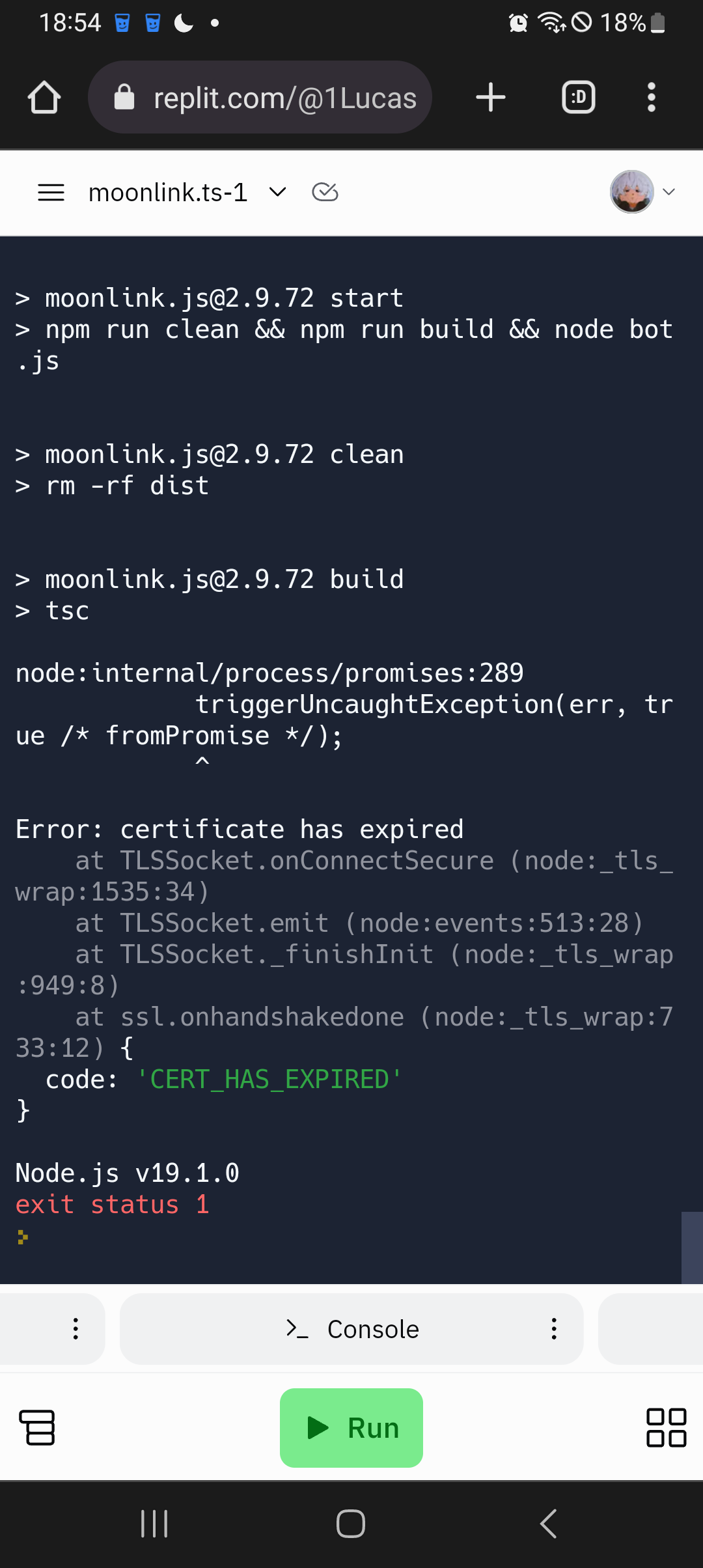
I've already deleted all the code and the error still hasn't disappeared, I just know that the bot is gone
@Ghos't Could you show the pause code part?
In the meantime I will try to fix this environment error
afraid-scarletOP•2y ago
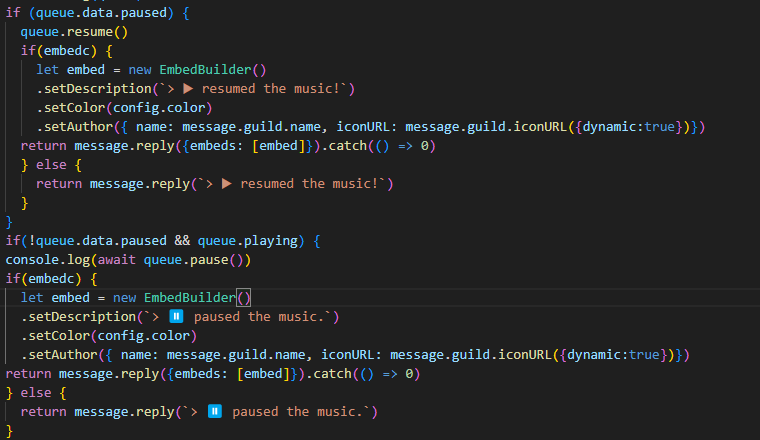
What defines queue?
afraid-scarletOP•2y ago

?play all i want
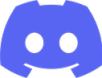
<:Nota_Musica__Mlink:960665601574055966>╺╸
Title:
<:Estrela_Mlink:960660485999587348>╺╸
Uri:
<:emoji_21:967836966714503168>╺╸
Author:
DisneyMusicVEVO
<:emoji_23:967837516558393365>╺╸
Duration:
0 Days, 0 Hours, 3 Minutes, 185 Seconds
Request for: 1lucas1.apk
Olivia Rodrigo - All I Want (Official Video) is playing now
?eval client.moon.players.get(message.guild.id).pause()
?play dumb dumb
?play dumb dumb
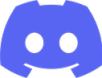
<:Nota_Musica__Mlink:960665601574055966>╺╸
Title:
<:Estrela_Mlink:960660485999587348>╺╸
Uri:
<:emoji_21:967836966714503168>╺╸
Author:
mazie
<:emoji_23:967837516558393365>╺╸
Duration:
0 Days, 0 Hours, 2 Minutes, 132 Seconds
Request for: 1lucas1.apk
?eval client.moon.players.get(message.guild.id).pause()
?eval client.moon.players.get(message.guild.id).pause()
?eval client.moon.players.get(message.guild.id).resume()
Okay, the error is the most beast there is! num if
@Ghos't I published the version correcting this bug
afraid-scarletOP•2y ago
thx man I appreciate that
You are welcome
afraid-scarletOP•2y ago
I still face a problem when I use the pause command, the first bot responds, but the second bot does not respond to the command
GG @Ghos't, you just advanced to level 3!
afraid-scarletOP•2y ago
first bot queue.data
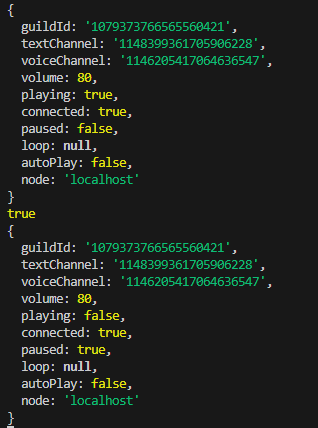
afraid-scarletOP•2y ago
second bot queue.data
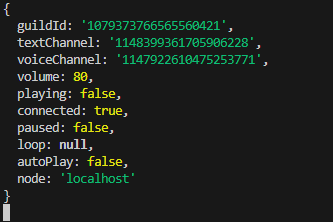
afraid-scarletOP•2y ago
@1Lucas1.apk
Mercy, I already left a console.log in the codes lol
afraid-scarletOP•2y ago
-
@1Lucas1.apk sorry for mention
Man, then I would need to see half of the code to find where the problem could be 😦
Send me the server he is on in private 🙂
afraid-scarletOP•2y ago
@1Lucas1.apk dose the new update fix the problem