❔ ✅ My code is pretty close to the right solution, but one test case still fails
So I have this solution for LeetCode's bulls and cows puzzle https://leetcode.com/problems/bulls-and-cows/
One test case still fails as per screenshot. The code for checking cows' count is not correct obviously, but I have no idea at all how to fix it. Could anyone kindly point me in the right direction?
public class Solution {
public string GetHint(string secret, string guess) {
int[] cows = new int[10];
int[] bulls = new int[10];
int bullCount = 0;
//bulls
for(int i = 0; i < secret.Length; i++){
if(secret[i] == guess[i]){
bullCount++;
} else {
bulls[secret[i]-'0']++;
cows[guess[i]-'0']++;
}
}
int cowCount = 0;
//cows
for(int j = 0; j < guess.Length; j++){
if(secret[j] != guess[j] && cows[guess[j]-'0'] > 0)
{
cowCount++;
cows[guess[j]-'0']--;
}
}
Console.WriteLine(cowCount);
Console.WriteLine($"{bullCount} A {cowCount} B");
return $"{bullCount}A{cowCount}B";
}
}
LeetCode
LeetCode - The World's Leading Online Programming Learning Platform
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
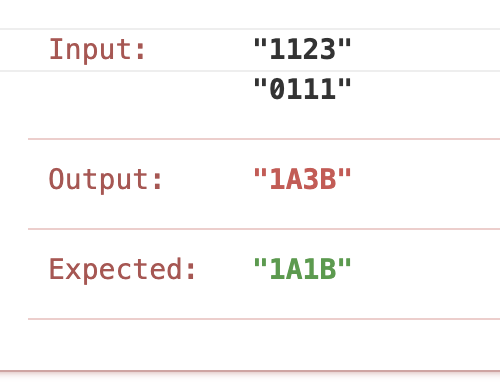
3 Replies
You'll need to make sure you're not counting a character from the guess string more than once when that character appears multiple times in the secret string
👍
Ok I have one more question @sinfluxx
I tried to remove the duplicate cow counts, but one of my code lines seems to be wrong. Maybe do you have any feedback on this?
public string GetHint(string secret, string guess) {
int[] cows = new int[10];
int[] bulls = new int[10];
int bullCount = 0;
//bulls
for(int i = 0; i < secret.Length; i++){
if(secret[i] == guess[i]){
bullCount++;
} else {
bulls[secret[i]-'0']++;
cows[guess[i]-'0']--;
}
}
int wrong = 0;
//cows
for(int j = 0; j < guess.Length; j++){
if(secret[j] != guess[j] && cows[guess[j]-'0'] > 0)
{
wrong++;
cows[guess[j]-'0']--;
}
}
int cowCount = secret.Length-bullCount-wrong; //this line does not seem to work
Console.WriteLine(cowCount);
Console.WriteLine($"{bullCount} A {cowCount} B");
return $"{bullCount}A{cowCount}B";
}
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.