✅ EF Core Relations don't seem to work properly
I'm trying to model a fairly complex set of relations in EntityFramework core / ASP.NET, but am failing to create a new entry as the system insists it already exists; yet the corresponding table in the DB is empty
27 Replies
relevant part of my dbcontext init:
and finally how I'm trying to create a new entry:
error message is
are you trying to update or insert?
_dbContext.Mods.Update(result);
is sugesting you trying to update. you should use a Mod
that you read from the _dbContext
first to update, instead of new
ing up a new instanceupsert. if the entity exists -> update it, if not -> create
update() works just fine for that by detecting whether the primary key exists or not
tbh i havent used it like that before
but the error message complains about 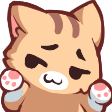
SupportedPlatform
which you new up too
have you tried useing the ones you read from db?
maybe it doesnt work that well for naviagation properties? 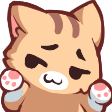
there are none in the DB
I am trying to insert them in this query
and i guess the platforms are unique too?
so
platforms
doesn't just contain duplicates?platforms
are unique and only contains the correct keys, yes.
SupportedPlatform
is essentially an IDictionary<Platform, string>
property on Mod
..which means serializing it into a separate tableWhy are you using IEnumerable?
https://learn.microsoft.com/en-us/ef/core/modeling/relationships/conventions also prefer conventions to explicit configuration
Conventions for relationship discovery - EF Core
How navigations, foreign keys, and other aspects of relationships are discovered by EF Core model building conventions
what else would I use?
List<T>
IEnumerable is not appropriate for representing a database relationship
well it never complained about it 😅
How are you supposed to add or remove from it?
I haven't gotten around to that yet, haha
Well you'll quickly discover you can't do that with IEnumerable 😛
ik but more like, I haven't needed to add/remove yet
so how do I use conventions to add constraints?
because that is what I've mainly been using the fluent api for
You explicitly configure what conventions don't suffice
okay, I've redone the DB and am now able to insert data properly.
however, I am unable to retrieve the supported platforms it seems
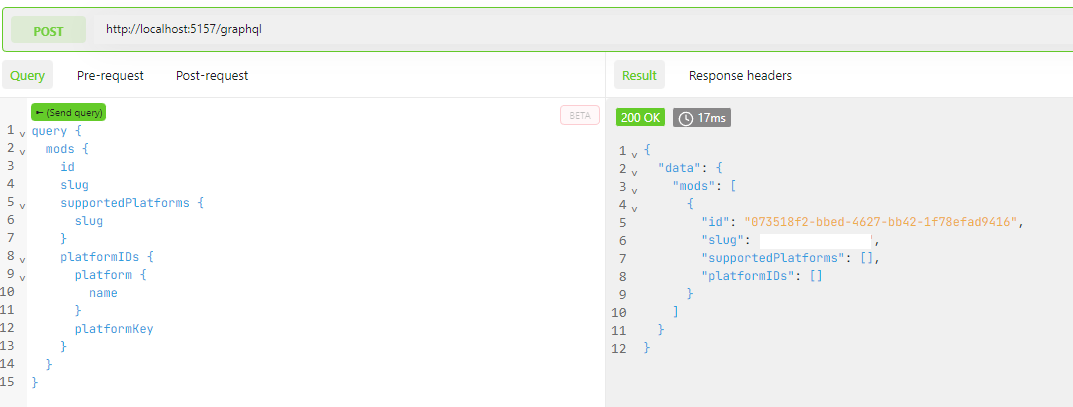
but the data does exist in the DB

so looks like the navigation properties aren't being populated 

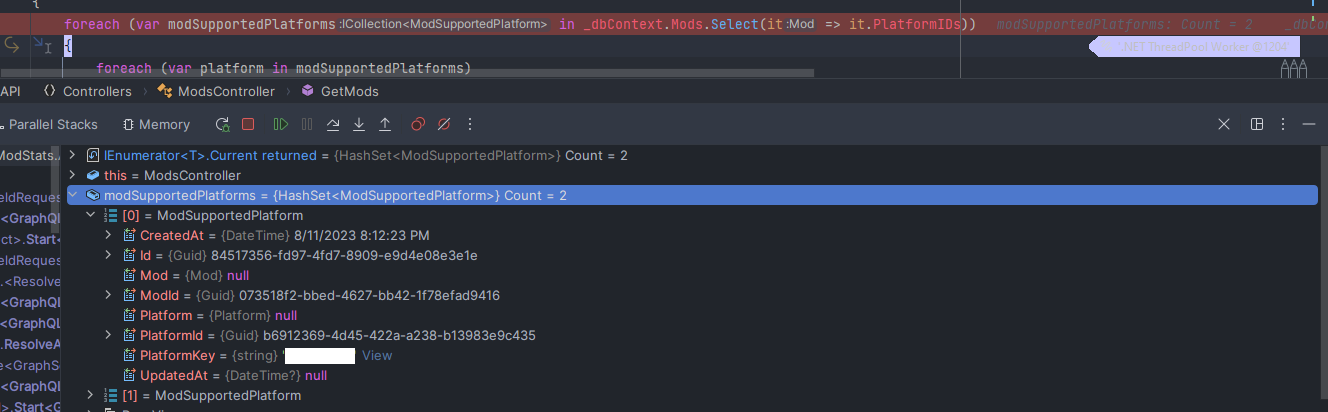
here's how I assign those relations:
Try letting EF infer by convention
Don't use configuration unless you need to
Also, you made and applied a migration right?
yes
update: tried it with implicit properties and even deleted + re-seeded the DB.
no change
also fwiw, when I send the above GQL query, this is the only database query that is executed:

any other ideas @jcotton42?
update:
solution was to enable lazy loading proxies via
Microsoft.EntityFrameworkCore.Proxies
, and mark all my navigation properties as virtual
.