❔ Trying to map CSVHelper to weirdly formed CSV
I have this CSV and I am struggling to write the class to use when reading it with CSVHelper. I am only trying to read E_Grid.
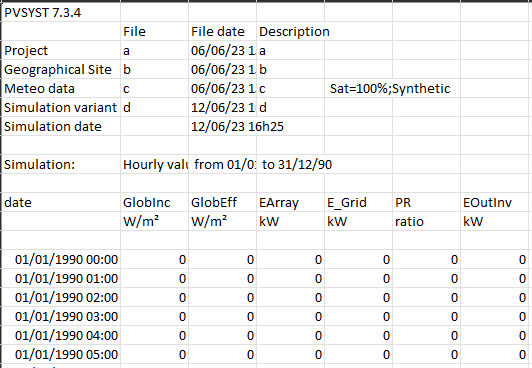
25 Replies
there is delimiter or its fixed ?
you can do semi-manual read of it
My current mapping is this:
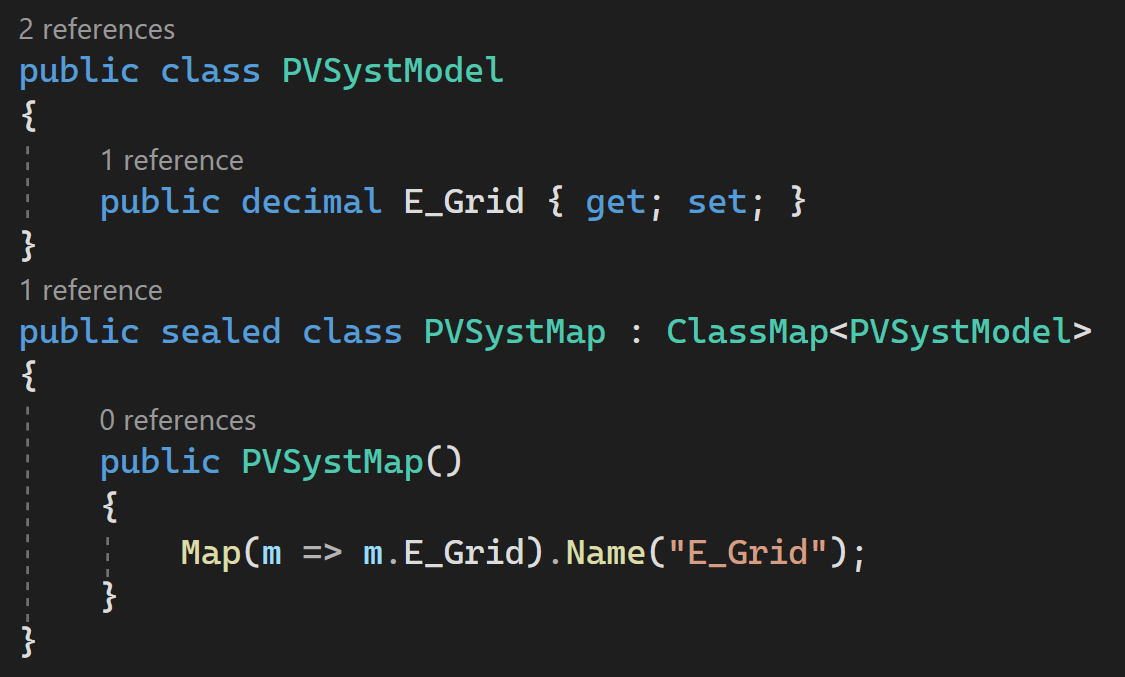
I've never had to deal with CSVs that don't have headings in the first row
dont use map for this case i guess
do a semi-manual if data is 'fixed'
like skiping the X first row
you can even set the number to skip in a application param so isnt fixed in code
What do I use as my delimeter?
if your file is a CSV oppen it with a normal file reader and not excel
you will see the delimiter
(if its a real csv)
usualy its ";"
the ; char
I have it open in notepad, I just have commas, no ;
so its coma
coma betwen fields ?
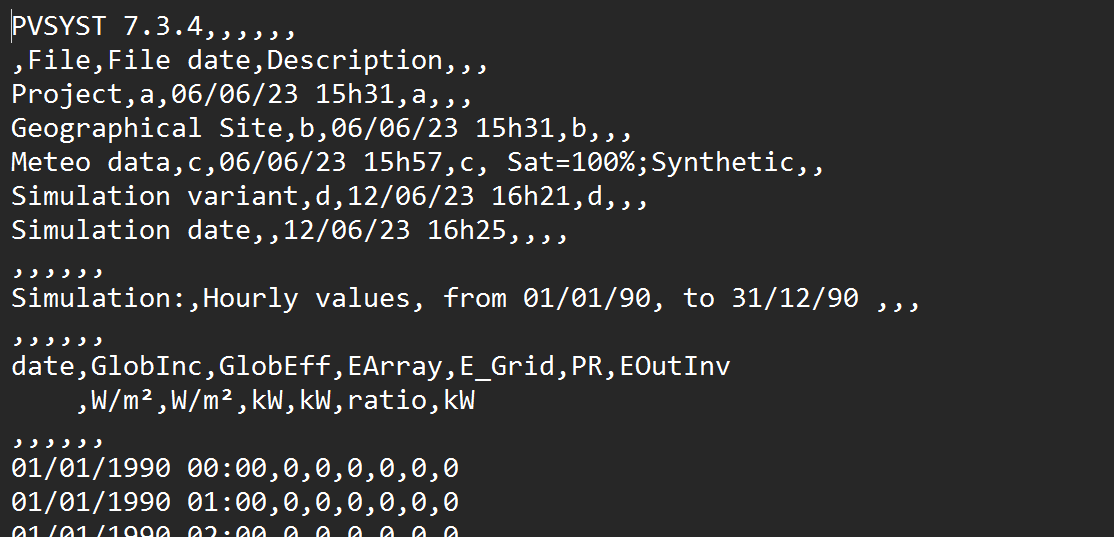
yep its coma delimiter
you can just do a Console.WriteLine(csv.GetField(4)); to see different value for the 5th field (start at 0 for the parameters of GetField = 1st value)
ran into "Synchronous reads are not supported" when starting the while loop
hmm weird, can you send me just few part of your csv ?
i may try
its working for me
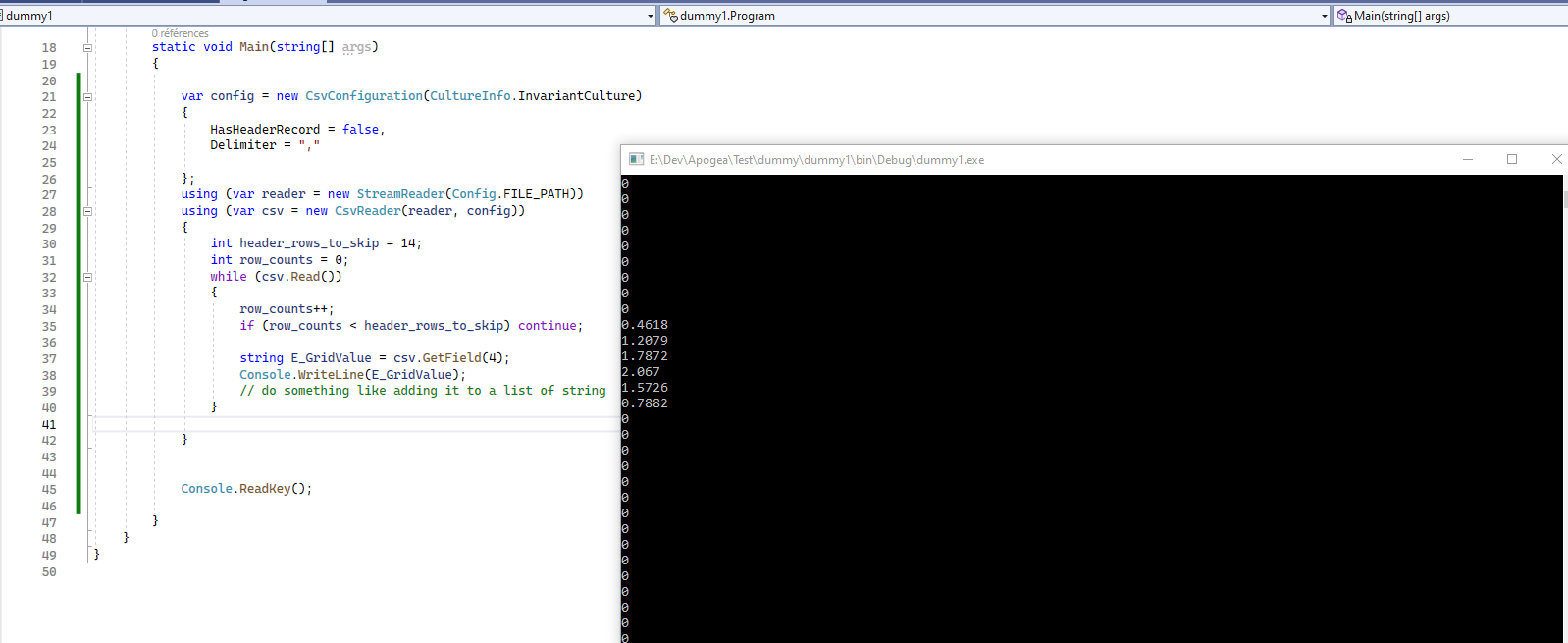
and to match your model i'll modify the code, but its just creating a list on starting then add the value to the list
I think my problem is the way I'm loading the file as it's thourhg an IBrowserFile
IBrowserFile ?
mebbe just try download it
i'm not confident with how stream work
as in uploading a file into blazor
almost time i'm doing what the doc say about filestream x')
but for a file on the drive
this work
set the file on your drive
and read it with the code above
you will see its work
Yes it does and thank you!
but for wich other streamReader to use i'm afraid i will not be a good help x')
no worries, thank you for all your help!
np and gl with your project :p
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.