unique id - increment object name with 1, 2, 3 and etc
is there a way to create an object with a unique id/name each time, for example:
I'm trying to create a circles object but want it to increment each time its created - circles1, circles2, and etc. On click I need this to happen:
let circles = 0;
const circleCoordinates = [];
function onClickFunc(){
circles++;
circleCoordinates.push({circles : {x: mouseX, y: mouseY}});
console.log(circles); // doesnt create circles1 and etc, just circles obj
}
How to solve this?7 Replies
It doesn't create
circles1
, circles2
, circles3
, etc because you're not telling it to :p
thanks I did something similar:
I tried this:
let circle = 0;
circle++;
const circleID = circle${circle}
const circleObj = {x : mouseX, y: mouseY};
circleCoordinates.push({ [circleID] : circleObj });
but I;m not able to access its properties : x and yInstead of doing backticks on the beginning and end of a line, do three in a row (```) followed by the language, then on the next line you can start your code. End the code with three more backticks (```) on their own line and that's how you get code blocks. My above message was formatted like this:
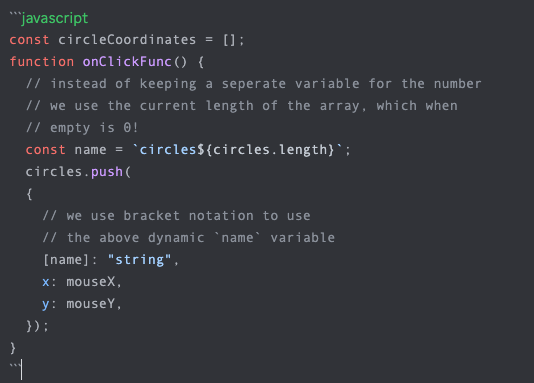
okay thanks
Back to the question at hand. I'm a bit uncertain about what exactly you're trying to do here.
I'm trying to create a circles object but want it to increment each time its created - circles1, circles2, and etc.(also, "etc" means, "and so forth"; no need to say "and etc." 😉 ) Are you wanting each object to have their own global variable with that name? Do you want to have one "master object" where you use the unique names to access them (
masterObj.object2
)? Because if you're pushing all objects to an array you already have a (mostly) unique ID: the array index. You can only have one value at each index, so do you need a name of object3
if your object is located in circleCoordinates[3]
?
I'm getting the feeling that this is an X/Y problem where you are trying to make the solution work and not asking how to accomplish your true goalso on click of the screen/canvas a circle is made.
I'm then trying to check in circles x and y coordinates in a distance function to calculate their distance
but from the approach I have, I cant access the x and y values
You're trying to calculate distances between circles? Yeah, this is an X/Y problem because that's not what you asked about. What's your code look like right now? What specific steps is your program supposed to do?
You'll probably need to make a codepen to share.