❔ Switch case on Int type
Hey, so I have a class with variable
_type
and I want to store a variable type in it (long, int, short, ubyte etc etc).
Later I want to do a switch on _type
something like
How can I do this?23 Replies
_type
variable is of type Type
?yea
and when I create this class I do something like
new Class(typeof(int));
You can't switch on
typeof()
since it's not a constant
You have to use good ol' if
s
(also, can you use generics instead of typeof
in your class?)how exactly?
if you had a variable of the type you can do that switch syntax
nukleer bomb
REPL Result: Failure
Exception: CompilationErrorException
Quoted by
<@536372826161283072> from #bot-spam (click here)
Compile: 579.576ms | Execution: 0.000ms | React with ❌ to remove this embed.
new Class<int>()
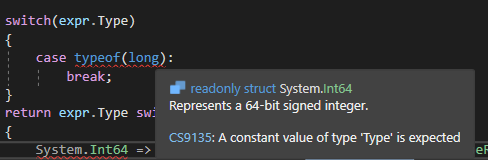
yeah, they were showing that it doesn't work
ah oke
the modix eval has an error itself
you can see it
yep, true
i'm not exactly familiar with generics
how would I then store this type in my class?
you wouldn't
you have it as the type parameter
there's not need to store it in any way
but how would I do a switch case on it later?
that depends on your use case
it'd be good to have more detail
alright
so let me explain it in more details
i am working on a tokenizer
i can have tokens like
i8
, i16
,i32
and i64
(for signed ints) and u8
u16
u32
u64
(for unsigned ints)
how do you mean "tokens"?
tokens are strings
where do they come from
i'm working on a simple programming language
and they come from my lexer
so
would return these tokens:
so, later using my NumberAST class I want to create LLVM consts
thats why i need to do switch case
to change the LLVMTypeRef
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.