✅ Why does my list get zeroed?
I am getting swamped with this puzzle https://leetcode.com/problems/find-all-anagrams-in-a-string/
My WIP is as follows;
public class Solution {
public IList<int> FindAnagrams(string s, string p) {
List<int> items = new List<int>();
int i = 0;
while(i < s.Length){ //loop through the s which is longer than p
if(p.Length == s.Substring(i).Length && p.Contains(s.Substring(i))){ //edge case where s and p are of the same length
items.Add(p.Length);
} else if(p.Contains(s.Substring(i))){
items.Add(p.Length);
}
i++;
}
return items;
}
}
This code gives me the result as per attached photo. How come my list does not store anything?LeetCode
LeetCode - The World's Leading Online Programming Learning Platform
Level up your coding skills and quickly land a job. This is the best place to expand your knowledge and get prepared for your next interview.
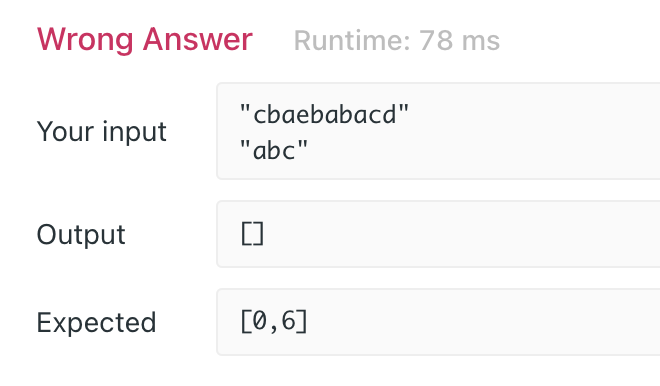
2 Replies
I don't think it's that the list doesn't store anything, it's that you never add anything to the list in the first place
The question is about searching for anagrams, and I'm not sure your solution takes that into account. (For example, looking at the sample answer above, you need to take every 3-character substring of cbaebabacd and check whether that substring can be rearranged into abc)
It looks like your solution just checks for substrings that match "abc" exactly without rearranging the letters
Ahhh ok thank you so much for pointing that out 🙏