β Error on my try and catch
It says that the variable 'ex' is declared but never used and how do i make the username and password more secure?
22 Replies
To make it more secure, i know that you should definitly use Query parameters and not string concatenations
$sqlinjection
Always parameterize queries!
https://i.imgur.com/uePzr0S.jpeg
Do not concatenate the query, example:
But instead always parameterize your queries. Look up the documentation for said Database library. If you are using
System.Data.SqlClient
refer to this https://learn.microsoft.com/en-us/dotnet/api/system.data.sqlclient.sqlcommand.parameters?view=dotnet-plat-ext-7.0#examplesIndeed your Exception ex is not used, i dont think this warning prevents you from running the app but maybe you could consider logging the exception, or writing it to the console with Console.WriteLine ?
rip the youtube i follow
is bad @>@
SQL Injection is VERY dangerous
Will lead to data breaches, and destruction.
i remember my teacher covered that
but i always seems to forget
thanks it works
now the applications is able to run
will work on my code to prevent sql injection
thank you so much ^^
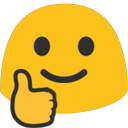
No worries βΊοΈ
Using the way you say
I should code it using this way
The @ username is from the html asp-for I think
Its not the @ for the html asp for, i dont think so
there is a good example of the use of parameters on the link above
And when you create your command :
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.like this? but there is an error at varchar. checked my database datatype is varchar(50)
but before i change anything. my code doesnt work. how can i troubleshoot?
The ExecuteNonQuery method doesn't work for scalar result i guess this could be one of the problems
You are trying to Select a row in the database, right ? ExecuteNonQuery is used for queries like update, delete and add
I think what you are looking for is ExecuteReader
Retrieving Data Using a DataReader - ADO.NET
Learn how to retrieve data using a DataReader in ADO.NET with this sample code. DataReader provides an unbuffered stream of data.
But there are other problems too, you should make use of "using" statements when you create your connection so that the garbage collector can Dispose it after you are finished using it
Also, when you say that your code doesn't work, what is the error that you see ? Something must be displayed i guess
i think is because i didnt create a data access layer before i do the api π€
https://www.c-sharpcorner.com/article/crud-operation-with-asp-net-core-2-1-mvc-using-ado-net/
But when i at this step my code like faced issue.
CRUD Operation With ASP.NET Core 2.1 MVC Using ADO.NET
In this article you will learn about CRUD Operation with ASP.NET Core 2.1 MVC using ADO.NET.
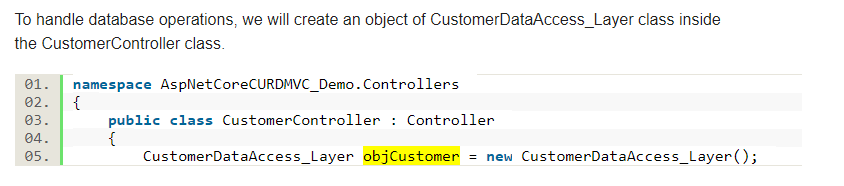
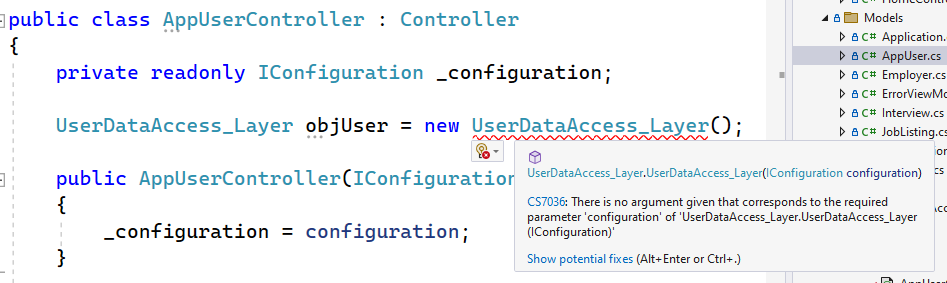
this one seems to cant be used
not sure why also having red lines @>@
even tho

Where is your sql command tho?
Data access layer is not mandatory
It is a good practice but not having one doesn't prevent your code from running
i forget about it @>@
but the reader["Id"].ToString() and command.ExecuteReader() having red underline
I've seen some things that could be coded better, so maybe you can change your code towards something more like this :
https://paste.mod.gg/ptnqnnqqkrtx/0
Keep in mind that i did not try it since i don't have your app on my computer. Maybe there are little things to change, but hopefully this will give you a better idea of how to make your code successfully run π
BlazeBin - ptnqnnqqkrtx
A tool for sharing your source code with the world!
And you are using an ExecuteReader for only one value (the User id), this is not optimal, you could run ExecuteScalar if you just want the Id.
If you want more than just one value, then ExecuteReader is a good fit !
Was this issue resolved? If so, run
/close
- otherwise I will mark this as stale and this post will be archived until there is new activity.