Creating a modal after a button click shows an "Unknown Interaction" error
I've created a system which dynamically creates a sequence of embeds, components and select fields for a Setup command. My hope is that I can also add into it an option for creating a Modal, so when I need a text input from the user I can show it in a more elegant way than just "type a message in chat".
Whenever I send a modal using this system (clicking a button can generate a Modal element), the modal shows up on Discord, but the bot crashes with an
DiscordAPIError[10062]: Unknown interaction
error.
Researching, I came across this:
Showing a modal must be the first response to an interaction. You cannot defer() or deferUpdate() then show a modal later.Does this mean that this kind of action is not possible, and that modals are exclusive to being used immediately after a Slash Command is executed, or is there a way to implement this? Error code: Code:
8 Replies
- What's your exact discord.js
npm list discord.js
and node node -v
version?
- Not a discord.js issue? Check out #other-js-ts.
- Consider reading #how-to-get-help to improve your question!
- Explain what exactly your issue is.
- Post the full error stack trace, not just the top part!
- Show your code!
- Issue solved? Press the button!Intended behaviour:
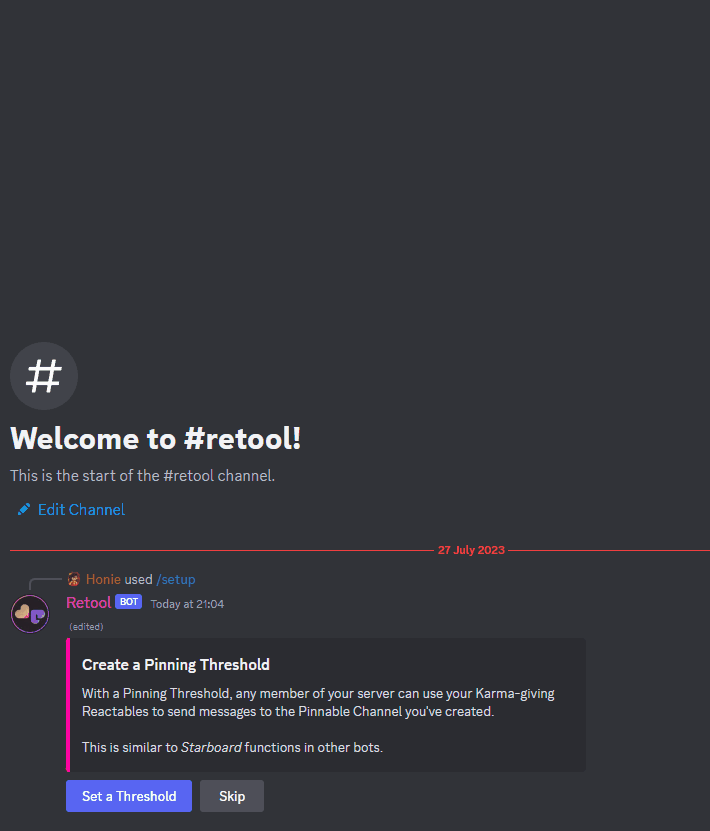
I'm not clear why you're calling i.deferUpdate() when the rest of the code before interaction.showModal() shouldn't take long to execute. You should be able to just remove that line without any issue. Calling collector.stop() also seems redundant since it has a max of 1 and would end anyways
What you saw about not being able to defer modal submits is correct, however I don't think it actually has an effect on what you are trying to do in this case
You're right about collector.stop() and the reply being edited not being necessary, but not deferring the update causes a This interaction failed message to appear after three seconds. The collector is awaiting a response, maybe?
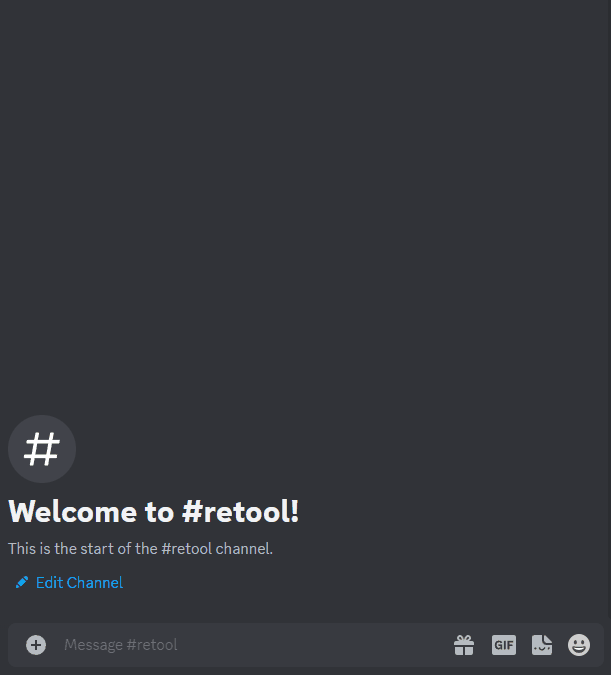
(That being said, the modal error doesn't appear anymore when not deferring the update, so that's progress.)
@Honie did you ever get this to work?
I believe I did make it work, but ended up scrapping the feature - returning to the same modal more than once works inconsistently
I'll dig into the code later and see if I can give you a sample